Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial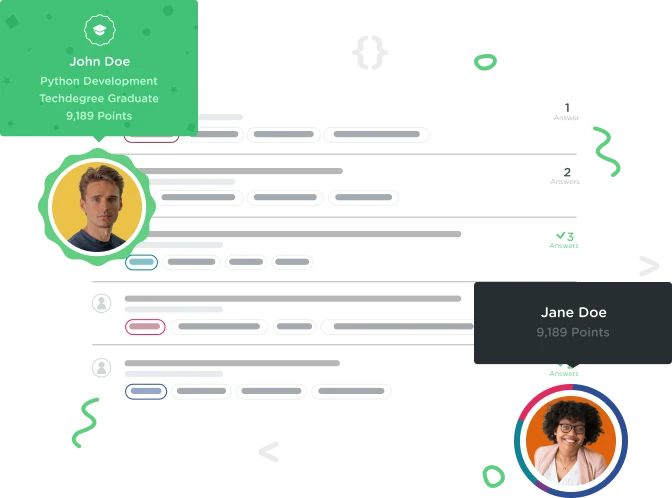
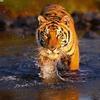
Konrad Pilch
2,435 PointsAdding movement
HI,
How can i add movement to my ball?
//Variables
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
var ballRadius = 25;
var x = canvas.width/2;
var y = canvas.height/2;
var dx = 5;
var dy = 5;
//Movement
var upPressed = false;
var rightPressed = false;
var downPressed = false;
var leftPressed = false;
//Ball
function drawBall() {
ctx.beginPath();
ctx.arc(x, y, ballRadius, 0, Math.PI*2);
ctx.fillStyle = ("red");
ctx.fill();
ctx.closePath();
}
//Draw
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBall();
//Ball Movement
requestAnimationFrame();
}
draw();
I know that the left is number 37, top 38, right 39 and down 40, and i get the kind of logic of if or swich statement, but how can i make it to move, the ball? and in future id like it to jump as well : p
2 Answers

Clayton Perszyk
Treehouse Moderator 48,850 PointsHey Konrad,
I was able to get the ball to move up, down, left, right. Here is the code; I added comments to explain what I did. If you have any questions, I would be glad to answer them.
//Variables
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
var ballRadius = 25;
var x = canvas.width/2;
var y = canvas.height/2;
// add event listener to move x or y
// up, down, left, right
// use the event's keyCode property to get the
// correct key code
document.addEventListener('keydown', function(event){
switch (event.keyCode) {
case 40:
y += 1;
break;
case 38:
y -= 1;
break;
case 37:
x -= 1;
break;
case 39:
x += 1;
break;
default:
throw new Error("Not a valid key.");
}
})
// draw ball is the same as your original
function drawBall() {
ctx.beginPath();
ctx.arc(x, y, ballRadius, 0, Math.PI*2);
ctx.fillStyle = ("red");
ctx.fill();
ctx.closePath();
}
//Draw
function draw() {
// clear the canvas, so a new ball can be drawn
ctx.clearRect(0, 0, canvas.width, canvas.height);
// draw ball with potentially new x and y coordinates
drawBall();
// pass the draw function as an argument
requestAnimationFrame(draw);
}
draw();
I haven't tried to make it bounce yet; I'm not sure what you are planning for the implementation (e.g. if a user clicks the ball, it should bounce up and down x times, or bounce across the screen, etc.).
I hope this helps.
Best,
Clayton
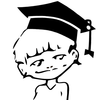
simhub
26,544 Pointshi Konrad,
here a little (very simple!) bounce function to keep the ball inside the canvas
//Bounce
function bounce () {
if ((x + ballRadius) > canvas.clientWidth) x -= 1;
if ((x - ballRadius) < 0) x += 1;
if ((y + ballRadius) > canvas.clientHeight) y -= 1;
if ((y - ballRadius) < 0) y += 1;
};
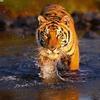
Konrad Pilch
2,435 Pointsthank you :D Do you know how could i add gravity to it so the ball starts on the canvas?
I need to select the bottom of the canvas, and then say to it that if it touches the ball, then there should be a collision that the ball will not be able to go throw it something liek atha?
Im trying to make a gravity, and then the movement, and now i was simply trying to figure out how to implement keys to a drawing canvas.
This stuff is reallly exciting.
Konrad Pilch
2,435 PointsKonrad Pilch
2,435 PointsThank you :) One question, how does this work?
Konrad Pilch
2,435 PointsKonrad Pilch
2,435 PointsAnd what about this technique? Im trying to learn OOP by making a mario like game : p
http://nokarma.org/2011/02/27/javascript-game-development-keyboard-input/
Clayton Perszyk
Treehouse Moderator 48,850 PointsClayton Perszyk
Treehouse Moderator 48,850 PointsThe callback function, which is the second argument passed to the addEventListener method, receives an object that corresponds to the event, and contains information relevant to that event. You can call it whatever you want but event makes the most sense. This object contains several properties, among them the keyCode property, which is used in this case to decide which switch statement to run. If you want to inspect it, you can put console.log(event) in the code and when run, open up the developer console in the browser and see what the event object contains.
Here are some helpful resources:
Clayton Perszyk
Treehouse Moderator 48,850 PointsClayton Perszyk
Treehouse Moderator 48,850 PointsAs far as oop in javascript goes, here are some resources Iv'e found helpful:
I would suggest taking the code you have right now and apply oop principles to it (i.e. make a Ball constructor, etc.). Then you can take what you've learned and apply it a mario style game.