Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial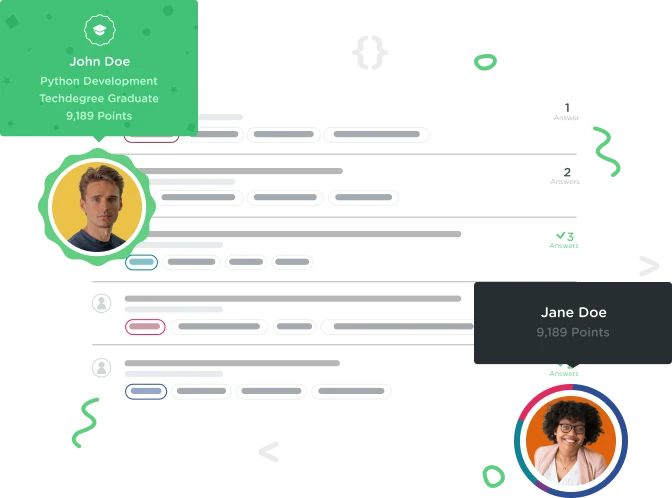

john vallone
890 PointsAdding numbers from an Array
How do you pull numbers from an array and add them into a sum?
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while sum < 44 {
counter += 1
sum = counter
}
2 Answers
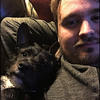
Matthew Long
28,407 PointsSometimes it helps to take a step back and reread what the problem is. You are correct that the variable counter
is used as an index for the array numbers
. However, from what I'm seeing your while loop isn't yet set up properly.
The first challenge: Create a while loop. The while loop should continue as long as the value of counter
is less than the number of items in the array
. (Hint: You can get that number by using the count property) They basically gave you the answer in plain english. In Swift, this is written as:
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
while counter < numbers.count {
counter += 1
}
You would read the above in English as while counter
is less than the length of numbers (or number of items in the numbers array..), increment counter by one.
For the second challenge: Using the value of counter
as an index value, retrieve each value from the array and add it to the value of sum
. This is where your prediction of "use counter
as an index for the numbers
array" comes in! This would look like sum += numbers[counter]
. This code takes the value of each index and adds it to the sum
variable.
The issue now is to decide where counter is incremented and where the sum is calculated within your while loop. In what order should these lines appear? I'll leave that for you. Happy coding!

john vallone
890 PointsI get it! That is very helpful.... I actually ran the numbers.count in my playground to get the "7" (though I could have counted it).
I didn't think to include that as an "entity" (or commend?? not sure what "numbers.count" is called). Also... I only thought of += as a means of adding a number to another value (or incrementing a value); but as you have stated, numbers.count resolves to a number which would then be added to "sum." I realize I'm stating the obvious but thanks.
I am (very) new to programming and am having some "ah ha!" moments. This is truly fun!!
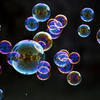
Jialong Zhang
9,821 PointsTo get information from array, use number["number inside"], so the counter starts with 0, and then increase by 1 for every loop.
you can read your codes this way,
first try: while 0 < 7
second try: while 1 <7
and so on
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
sum += numbers[counter]
counter += 1
}
print(sum)

john vallone
890 PointsThis is very helpful! Thank you for your help and posting :).
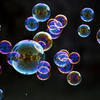
Jialong Zhang
9,821 PointsYouβre welcome! Glad I could help.
john vallone
890 Pointsjohn vallone
890 PointsI didn't understand the concept of the index here. The variable counter indexes my array (I think) calling consecutive data elements within the numbers array and adding them to sum with each iteration not to exceed 7.
should be:
let numbers = [2,8,1,16,4,3,9,10]
var sum = 0
var counter = 0
// Enter your code below
while counter < 7 {
}