Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial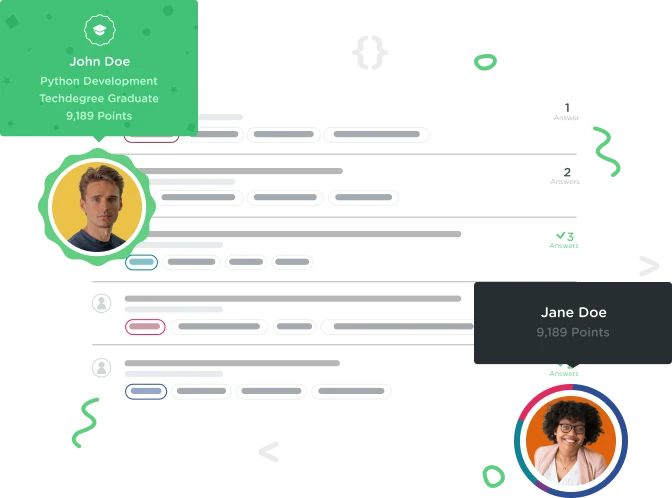

Dennis Dee
1,306 PointsAdding Secondary Data with a SimpleAdapter task 3
I am pretty lost on the Array being specified in this task.Now create a SimpleAdapter variable. It's constructor has 5 parameters. 1. The context (use 'this'). 2. The array to be adapted. 3. The layout for each item (use 'android.R.layout.simple_list_item_2'). 4. An array of keys needed to map values (use the 'keys' array). 5. An array of int IDs where the values are placed (use the 'ids' array). Everything seems pretty straight forward except I cannot seem to be able to find out the array to be adapted. Any help would be appreciated, here are the lines of code in question.
String[] keys = { KEY_NAME, KEY_URL };
int[] ids = { android.R.id.text1, android.R.id.text2 };
SimpleAdapter = new SimpleAdapter(this,websites,android.R.layout.simple_list_item_2,keys,ids);
4 Answers

Dennis Dee
1,306 Pointsimport android.os.Bundle;
import android.widget.SimpleAdapter;
public class WebsiteListActivity extends ListActivity {
public static final String KEY_NAME = "name";
public static final String KEY_URL = "url";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_website_list);
String[] keys = { KEY_NAME, KEY_URL };
int[] ids = { android.R.id.text1, android.R.id.text2 };
SimpleAdapter = new SimpleAdapter(this,websites,android.R.layout.simple_list_item_2,keys,ids);
final HashMap<String, String> mName = new HashMap<String, String>();
final HashMap<String, String> mUrl = new HashMap<String, String>();
mName.put(KEY_NAME, "Treehouse");
mName.put(KEY_URL, "http://teamtreehouse.com");
mUrl.put(KEY_NAME, "Michael Quiapos");
mUrl.put(KEY_URL, "http://michaelquiapos.com");
ArrayList<HashMap<String, String>> websites = new ArrayList<HashMap<String, String>>();
websites.add(mName); websites.add(mUrl);
}
}

Brian Chewning
7,270 Pointsimport android.os.Bundle; import android.widget.SimpleAdapter;
public class WebsiteListActivity extends ListActivity {
public static final String KEY_NAME = "name";
public static final String KEY_URL = "url";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_website_list);
String[] keys = { KEY_NAME, KEY_URL };
int[] ids = { android.R.id.text1, android.R.id.text2 };
SimpleAdapter = new SimpleAdapter(this, websites, android.R.layout.simple_list_item_2, keys, ids);
// Add code here!
HashMap<String, String> name = new HashMap<String, String>();
name.put(KEY_NAME, "one");
name.put(KEY_URL, "two");
HashMap<String, String> url = new HashMap<String, String>();
url.put(KEY_NAME, "three");
url.put(KEY_URL, "four");
ArrayList<HashMap<String, String>> websites = new ArrayList<HashMap<String, String>> ();
websites.add(name); websites.add(url); } }
What am I doing wrong?

Peter LABORDE
2,124 PointsYou have not given your SimpleAdapter a name eg, adapter
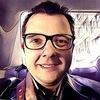
durul
62,690 Pointsimport android.os.Bundle; import android.widget.SimpleAdapter;
public class WebsiteListActivity extends ListActivity {
public static final String KEY_NAME = "name";
public static final String KEY_URL = "url";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_website_list);
String[] keys = { KEY_NAME, KEY_URL };
int[] ids = { android.R.id.text1, android.R.id.text2 };
// Add code here!
HashMap<String, String> site1 = new HashMap<String, String>();
HashMap<String, String> site2 = new HashMap<String, String>();
site1.put(KEY_NAME, "d" );
site1.put(KEY_URL, "dd" );
site2.put(KEY_NAME, "e" );
site2.put(KEY_URL, "ee" );
ArrayList<HashMap<String, String>> websites = new ArrayList<HashMap<String, String>> ();
websites.add(site1);
websites.add(site2);
SimpleAdapter adapter = new SimpleAdapter(this, websites,
android.R.layout.simple_list_item_2,
keys, ids);
setListAdapter(adapter);
}
}