Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial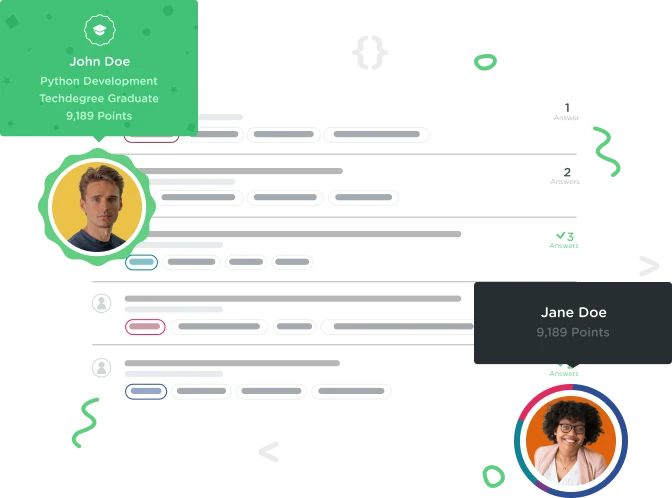
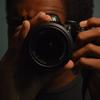
Jordan Welch
1,771 PointsAdding Sounds ERROR Crystal Ball has stopped.
Everything was working flawlessly with my application, but as I finished this lesson and went to test my sound my app will no longer run. Here is my activity_Main.java
package com.example.crystalball;
import android.graphics.drawable.AnimationDrawable;
import android.media.MediaPlayer;
import android.media.MediaPlayer.OnCompletionListener;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.animation.AlphaAnimation;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.crystalball.R.raw;
public class MainActivity extends ActionBarActivity {
private CrystalBall mCrystalBall = new CrystalBall();
private TextView mAnswerLabel;
private Button mGetAnswerButton;
private ImageView mCrystalBallImage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Assign the Views from the layout file
mAnswerLabel = (TextView) findViewById(R.id.textView1);
mGetAnswerButton= (Button) findViewById(R.id.button1);
mCrystalBallImage.setImageResource(R.drawable.ball_animation);
mGetAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String answer = mCrystalBall.getAnAnswer();
// Update the label with out dynamic answer
mAnswerLabel.setText(answer);
animateCrystalBall();
animateAnswer();
playSound();
}
});
}
private void animateCrystalBall() {
AnimationDrawable ballAnimation = (AnimationDrawable) mCrystalBallImage.getDrawable();
if (ballAnimation.isRunning()) {
ballAnimation.stop();
}
ballAnimation.start();
}
private void animateAnswer() {
AlphaAnimation fadeinAnimation = new AlphaAnimation(0, 1);
fadeinAnimation.setDuration(1500);
fadeinAnimation.setFillAfter(true);
mAnswerLabel.setAnimation(fadeinAnimation);
}
private void playSound() {
MediaPlayer player = MediaPlayer.create(this, raw.crystal_ball);
player.start();
player.setOnCompletionListener(new OnCompletionListener() {
public void onCompletion(MediaPlayer mp) {
mp.release();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
All help would be greatly appreciated I'm so close to finishing this app, I can taste it. Ben Jakuben Also I can't get markdown to work but that doesen't bother me very much
12 Answers

Ben Jakuben
Treehouse TeacherAh, disregard my other post. It jumped out at me here. The errors in Eclipse should help you identify where the problem is, but check out this line:
MediaPlayer player = MediaPlayer.create(this, raw.crystal_ball);
The raw
directory is a child of res
, and to access all those directories we always need to use the R class, like this:
R.raw.crystal_ball

Guillaume Maka
Courses Plus Student 10,224 PointsCan you provide us a screenshot ?
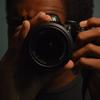
Jordan Welch
1,771 Points
Ben Jakuben
Treehouse TeacherYour screenshot is missing the appcompat_v7
folder, which is required under the latest Android tools update. Did you intentionally or accidentally delete that?
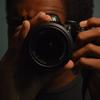
Jordan Welch
1,771 Pointsthanks ben we are making progress I added that back and I got rid of the red x on the style xml now to fix this activity main :/

Ben Jakuben
Treehouse TeacherOkay, if the red x's are gone on the style files, then hopefully all you need to do is clean and rebuild again. You need to force the R class to be regenerated again now that everything in the res
directory is error-free.
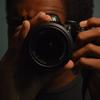
Jordan Welch
1,771 PointsI know how to clean but how do you rebuild?

Ben Jakuben
Treehouse TeacherIt's probably building automatically for you. If you clean, do you still get errors? If so, can you screenshot them again?
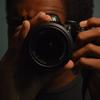
Jordan Welch
1,771 Pointsafter cleaning nothing changed :/ http://gyazo.com/c3bde108c17262bb91e835d54b6a1b58 this is so strange i looked on google and its hard to find anyone with similar problems to me

Ben Jakuben
Treehouse TeacherYou may need to add the appcompat library as a library project again. Right click on your project, go to Properties, then click on Android. The Library section at the bottom should contain a reference to appcompat_v7 with a green check mark. If it's missing or with a red x, add it back in using the Add button.
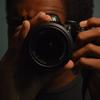
Jordan Welch
1,771 PointsI have a green check mark.

Ben Jakuben
Treehouse TeacherAh, I just caught it in your latest screenshot. At some point all your import statements were thrown out the window. Go back into MainActivity.java and hit Ctrl+Shift+O to organize your imports. That should pull everything in that you need.
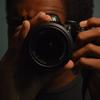
Jordan Welch
1,771 Pointshttp://gyazo.com/577de8b5dca79cc6557b76dc96e89f6f errors went away for a quick second and then they came back, this is so frustrating

Ben Jakuben
Treehouse TeacherThis may be silly, but that latest screenshot shows unsaved changes to MainActivity.java. Did you save it after reorganizing imports?
If you saved it and it still doesn't work, zip your project and email it to help@teamtreehouse.com and I can troubleshoot it locally.
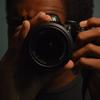
Jordan Welch
1,771 PointsBen you are the man! It's always the little things haha. I really want to thank you so much for all your help. Due to this simple conversation i'm probably gonna stick with this website after my free trial! Have a great day, many thanks.

Ben Jakuben
Treehouse TeacherHaha - that's programming in a nutshell! They get fewer and fewer with more experience, but the little stuff still gets all of us.
Glad you had the patience to stick with it. Hopefully it'll be smooth sailing now as you go along. :)
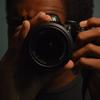
Jordan Welch
1,771 PointsPlease don't hate me haha I have 0 errors but when I try to run the app it still crashes here is my updated code
package com.example.crystalball;
import android.graphics.drawable.AnimationDrawable;
import android.media.MediaPlayer;
import android.media.MediaPlayer.OnCompletionListener;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.animation.AlphaAnimation;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
private CrystalBall mCrystalBall = new CrystalBall();
private TextView mAnswerLabel;
private Button mGetAnswerButton;
private ImageView mCrystalBallImage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Assign the Views from the layout file
mAnswerLabel = (TextView) findViewById(R.id.textView1);
mGetAnswerButton= (Button) findViewById(R.id.button1);
mCrystalBallImage.setImageResource(R.drawable.ball_animation);
mGetAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String answer = mCrystalBall.getAnAnswer();
// Update the label with out dynamic answer
mAnswerLabel.setText(answer);
animateCrystalBall();
animateAnswer();
playSound();
}
});
}
private void animateCrystalBall() {
AnimationDrawable ballAnimation = (AnimationDrawable) mCrystalBallImage.getDrawable();
if (ballAnimation.isRunning()) {
ballAnimation.stop();
}
ballAnimation.start();
}
private void animateAnswer() {
AlphaAnimation fadeinAnimation = new AlphaAnimation(0, 1);
fadeinAnimation.setDuration(1500);
fadeinAnimation.setFillAfter(true);
mAnswerLabel.setAnimation(fadeinAnimation);
}
private void playSound() {
MediaPlayer player = MediaPlayer.create(this, R.raw.crystal_ball);
player.start();
player.setOnCompletionListener(new OnCompletionListener() {
public void onCompletion(MediaPlayer mp) {
mp.release();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main,menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}

Ben Jakuben
Treehouse TeacherCan you create a new post and paste in this code and the new errors you are getting? When the app crashes it should log errors in the logcat tab at the bottom of Eclipse. Check that and let us know what it says in the new post. (This one is getting too messy. )
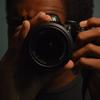
Jordan Welch
1,771 PointsBen Jakuben just made a new post https://teamtreehouse.com/forum/crystal-ball-misc-errors-cont

Guillaume Maka
Courses Plus Student 10,224 PointsCan you post the code of res/values/styles.xml ?
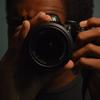
Jordan Welch
1,771 Points<resources>
<!--
Base application theme, dependent on API level. This theme is replaced
by AppBaseTheme from res/values-vXX/styles.xml on newer devices.
-->
<style name="AppBaseTheme" parent="Theme.AppCompat.Light">
<!--
Theme customizations available in newer API levels can go in
res/values-vXX/styles.xml, while customizations related to
backward-compatibility can go here.
-->
</style>
<!-- Application theme. -->
<style name="AppTheme" parent="AppBaseTheme">
<!--
All customizations that are NOT specific to a particular API-level can go here.
-->
<item name="android:windowNoTitle">true</item>
<!-- Hides the Action Bar -->
<item name="android:windowFullscreen">true</item>
<!-- Hides the status bar -->
</style>
</resources>
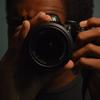
Jordan Welch
1,771 Points<resources>
<!--
Base application theme, dependent on API level. This theme is replaced
by AppBaseTheme from res/values-vXX/styles.xml on newer devices.
-->
<style name="AppBaseTheme" parent="Theme.AppCompat.Light">
<!--
Theme customizations available in newer API levels can go in
res/values-vXX/styles.xml, while customizations related to
backward-compatibility can go here.
-->
</style>
<!-- Application theme. -->
<style name="AppTheme" parent="AppBaseTheme">
<!--
All customizations that are NOT specific to a particular API-level can go here.
-->
<item name="android:windowNoTitle">true</item>
<!-- Hides the Action Bar -->
<item name="android:windowFullscreen">true</item>
<!-- Hides the status bar -->
</style>
</resources>
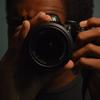
Jordan Welch
1,771 PointsI can never get markdown to work.

Ben Jakuben
Treehouse TeacherUse backticks ` instead of single quotes. :)

Guillaume Maka
Courses Plus Student 10,224 PointsIn eclipse go to window menu > Show view > other and in General double click on "Error Log", the new view will appear at the bottom maximize the window to show all the error an take a screenshot or if you want just share the project on github.
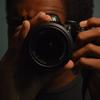
Jordan Welch
1,771 Points
Guillaume Maka
Courses Plus Student 10,224 PointsOups my mistake ! wrong view instead of Error log view show the Problems view and filter to show only errors. Sorry.
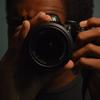
Jordan Welch
1,771 Points
Guillaume Maka
Courses Plus Student 10,224 PointsIt seem eclipse can't find the theme Theme.AppCompat.Light
<style name="AppBaseTheme" parent="Theme.AppCompat.Light">
replace with (also in v14 and v11 styles.xml):
<style name="AppBaseTheme" parent="android:Theme.Light">
or if you want to use the AppCompat theme see; http://stackoverflow.com/questions/17870881/cant-find-theme-appcompat-light-for-new-android-actionbar-support
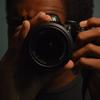
Jordan Welch
1,771 Pointsdidn't change anything

Guillaume Maka
Courses Plus Student 10,224 PointsDid you clean the project ?
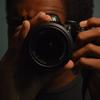
Jordan Welch
1,771 Pointsmany times

Guillaume Maka
Courses Plus Student 10,224 PointsFollow this setup: https://developer.android.com/tools/support-library/setup.html > Section Adding libraries with resources
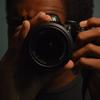
Jordan Welch
1,771 Pointsdone didnt fix anything

Guillaume Maka
Courses Plus Student 10,224 PointsDo you have the same directory tree http://gyazo.com/5341ef84196f8b768ae93320aa3b10b3 ?

Guillaume Maka
Courses Plus Student 10,224 PointsAfter this it should work I test it and it works on my machine !
Endre Juhasz
613 PointsAfter adding playSound, it actually throws a null pointer exception for me :
java.lang.NullPointerException at com.example.kn101.crystalball.MainActivity.playSound(MainActivity.java:63)
Line 63 is: player.start();
Is it not something like sound needs to be enabled for the virtual android device or something?
(I'm using Android Studio + Ubuntu 12.04)

Guillaume Maka
Courses Plus Student 10,224 PointsMake sure "player" variable is properly initialize (but as I see its initialize) I don't know if the virtual device has an option to enable it maybe check if the app need some permission to play a sound (I don't think so).
Endre Juhasz
613 PointsThe odd thing is, when I put a brakepoint to : player.start(); then the debugger says player=NULL. Should've been initialized by that point.
private void playSound()
{
MediaPlayer player=MediaPlayer.create(this, R.raw.crystal_ball);
player.start();
player.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mediaPlayer) {
mediaPlayer.release();
}
});
}
So it might not initialize properly the line before.
It still might be the virtual device though...

Guillaume Maka
Courses Plus Student 10,224 PointsI read the API reference and MediaPlayer.create that said:
public static MediaPlayer create (Context context, int resid)
Added in API level 1
Convenience method to create a MediaPlayer for a given resource id. On success, prepare() will already have been called and must not be called again.
When done with the MediaPlayer, you should call release(), to free the resources. If not released, too many MediaPlayer instances will result in an exception.
Parameters
context the Context to use
resid the raw resource id (R.raw.<something>) for the resource to use as the datasource
Returns
a MediaPlayer object, or null if creation failed.
The only thing we can verify its the context and the resid
The context should be valid because it's the Activity context and last the resid make sure the file exist (clean and rebuild the project to regenerate R)
Jordan Welch
1,771 PointsJordan Welch
1,771 PointsThanks but that gave me another error :/ It says R can't be resolved to a variable
Guillaume Maka
Courses Plus Student 10,224 PointsGuillaume Maka
Courses Plus Student 10,224 PointsTry to clean the project to regenerate R file and check if the res folder doesn't contain any error (sometimes errors in that folder break the R regeneration process).
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherOkay, that's progress! That means there's an error somewhere in your
res
directory. Do any files have a little red x in the Package Explorer? Does thecrystal_ball.mp3
file show up in there?Jordan Welch
1,771 PointsJordan Welch
1,771 PointsI have a red x in a lot of things ill tell you all of them
src com.example.crystalball mainactivity.java mainactivity Everything under main activity and on values and styles.xml this is just so crazy because a day ago everything worked near perfect! thanks for all the help ben and guillaume
Jordan Welch
1,771 PointsJordan Welch
1,771 Pointshttps://teamtreehouse.com/forum/crystal-ball-misc-errors-cont feel free to help me when you get a chance Ben Jakuben