Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial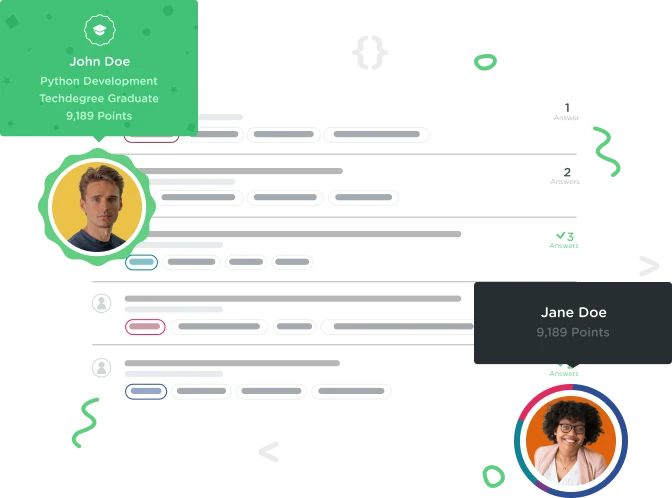

Brian Schmitz
11,167 PointsAdding the ready state function
Can someone please advise on what I am missing here in adding the conditional statement. Thanks
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if(xhr.readyState === 4);
};
xhr.open('GET', 'sidebar.html');
xhr.send();
5 Answers
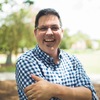
Dave McFarland
Treehouse TeacherYou set the innerHTML property to the responseText
property of the xhr
object:
document.getElementById('sidebar').innerHTML = xhr.responseText;
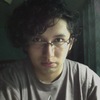
JJ RomMar
9,906 Pointsvar xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if(xhr.readyState === 4 && xhr.readyState === 200 ){ xhr.status; };
};
xhr.open('GET', 'sidebar.html'); xhr.send();
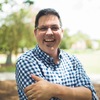
Dave McFarland
Treehouse TeacherYou should add a set of curly braces to the conditional statement:
if (xhr.readyState === 4) {
}

Brian Schmitz
11,167 PointsThanks Dave, can you help me out with the last part here? What do I need to pass to the innerHTML. Do I need to set a variable and then set that variable equal to .innerHTML?
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if(xhr.readyState === 4) {
if(xhr.status === 200){
}
}
document.getElementById('sidebar').innerHTML = ?
};
xhr.open('GET', 'sidebar.html');
xhr.send();

Gabriel Ward
20,222 PointsHi Dave, thank you for your great tutorials. I really wanted to ask you a question directly and thought I might be able to reach you here. I'm going through the Front End Web Development track, and I'm wondering, does one need to also be an expert in PHP? You say in the videos here that we'll not be going into the backend side of AJAX. There is so much to learn and it can seem a bit overhwhelming. Do you code both backend and frontend? I'd be extremely grateful for and advice/thoughts you have to offer.
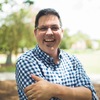
Dave McFarland
Treehouse TeacherThis doesn't look right:
<script type="text/javascript" src="path/to/instafeed.min.js"></script>
The path/to/
part means you have a folder named path
and inside that a folder named to
.
Have you tried:
<script type="text/javascript" src="instafeed.min.js"></script>

Brian Schmitz
11,167 PointsI just adjusted that (removed the "path/to") and I didn't see anything come through. I went into my Instagram account and it shows that instafeed is an authorized application to access my photos, friend list, and profile info (not that I want all of that info anyway to show on the page).
Per the documentation on GitHub:
Instafeed with automatically look for a <div id="instafeed"></div> and fill it with linked thumbnails. Of course, you can easily change this behavior using standard options. Also check out the advanced options for some advanced ways of customizing Instafeed.js.
I added that div to my html and tried again and didn't see anything either.
Because I am trying to pull from a specific account I can't really tell if I need a client ID for this? I did register for a UserID and accessToken and have that inserted into the code per my post before. Based on the code on instafeed for pulling from a specific user account - it doesn't look like I would put a client ID anywhere. Only if I wanted to pull photos with a specific tag name would I need client id.
I must be missing something small....Any thoughts? Or alternative ways you would get these photos?
Thanks, Brian

Brian Schmitz
11,167 PointsThat div I added to my html was
<div id="instafeed"></div>
Thought maybe that would pull it through but I didn't see anything.
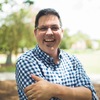
Dave McFarland
Treehouse Teacherwhat is the consloe saying now? what are the errors?

Brian Schmitz
11,167 PointsHey Dave,
The console is saying the same things as before:
Failed to load resource: the server responded with a status of 404 (Not Found)
Uncaught ReferenceError: Instafeed is not defined
I actually had that same code and instafeed.min.js file loaded into my page when I sent you the errors the last time.
I'm not sure...
Thanks, Brian
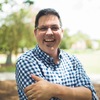
Dave McFarland
Treehouse TeacherHi Brian
These errors mean the instafeed.js is NOT loading. At this point, that is the only problem. For whatever reason the instafeed.js file is NOT where your <script>
tag says it is. I don't think I can help any further without seeing your files and file structure. You need to make sure the instafeed.js file is in the workspace, and that the <script>
tag's `src
property is pointing to it. That's the problem.

Brian Schmitz
11,167 PointsThanks for the help, Dave. I got it all figured out and running on the page so we are set. Appreciate the time!

Brian Schmitz
11,167 PointsHi Dave,
I was hoping I could ask you a question on another feature I need to add to the site I am working on - I have those Instagram pictures on the site - 4 evenly across the width of the page. I need to add a carousel or left to right scroll so that a user can scroll over to see more of the pictures once more then 4 are being pulled from Instagram. I would like to put the "<" ">" on both sided of the pictures and allow them to click on those to scroll left or right.
I've been searching through Google to see how to do that but I was wondering if you had an overview on how that is set-up, or a suggestion on how to go about adding that to my site? Could you help me get started in the right direction or point me to somewhere that you think would help?
Thanks for your time, Brian

Matthew Kustes
9,304 PointsBrian Schmitz what was the fix for the instafeed.js ---- I'm having the exact same problem.
I also realize this is an old thread.
Brian Schmitz
11,167 PointsBrian Schmitz
11,167 PointsHi Dave McFarland,
I am reaching out hoping you could help me out with a couple things. I am adding a couple pieces of functionality to a home page I am building for a business. Its a pretty basic home page using HTML, Bootstrap 3.0, they also are using a new service called Cratejoy that takes care of the subscription part of the service. The client wants to add a video to the top of the home and then an Instagram Reel farther down the home page - just like this site does: http://www.zamplebox.com/. I am wondering what particular code would you suggest? Would I want to add Ajax for the Instagram Reel to continually update the page as new updates come in? Or what type of Javascript code would I use there?
For the video, I was going to use HTML5 <video> tags with <source src> inside for that part. Is there a different route you would suggest? They want the video to take up the entire top part of the page, with the "play" button for the user to click on to start the video, just like the zamplebox site.
I would very much appreciate some help with this if you have the time. I've hit a road block and could use some direction.
Thank you for your time. Brian
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherBrian Schmitz
I don't believe you can use just JavaScript and AJAX to get Instagram images on a page. You can use AJAX but you need a server-side program that talks to Instragram. Because of the way their authentication works, you have to connect to Instragram using a special access token -- this isn't something you would want to reveal in your code, because then anyone looking at your code would have access to that token and could access Instragram as you -- bad idea.
In other words you can use AJAX, but you have the AJAX talk to your server, and your server talks to Instagram, who sends the image data back to your server and then your server returns it to the client via AJAX.
You can read more about their authentication at http://instagram.com/developer/authentication/
For the video an video tag should be fine. I don't have experience creating a full page video like on zamplebox, but it's common so a quick Google search should point you in the right direction.
Brian Schmitz
11,167 PointsBrian Schmitz
11,167 PointsHi Dave,
Thanks for the info. I was looking at that documentation and doing some research and I saw this plug-in at: instafeedjs.com
It looks like a pretty simple way to add photos to your website - just downloading a file and then adding some code. I also got a userID and accessToken as the documentation noted. My current code is this (and I am working right on a Workspace within Treehouse here for my text editor).
In my JS folder I have downloaded and added the "instafeed.min.js" file.
<script src="http://code.jquery.com/jquery.js"></script> <script src="js/bootstrap.min.js"></script> <!-- Instafeed code below--> <script type="text/javascript" src="path/to/instafeed.min.js"></script> <script type="text/javascript"> var userFeed = new Instafeed({ get: 'BSCHMITZ9', userId: 1395425564, accessToken: '1395425564.467ede5.c4e1d31e4f6c4d879b2790427ae89d5c' }); userFeed.run(); </script>
I'm not seeing that code pull the pictures from my account onto the website though with this code. Do you see any issues there or anything I am missing to make this work? Am I missing "scope" criteria for what I want to pull? If so, how to I add that into the code?
I know you're busy but I do very much appreciate the help, been working on this for a while and would like figure it out.
Thanks a lot, Brian
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherHi Brian Schmitz
I'm not familiar with instafeed.js. Have you opened the JavaScript console to see if there are any errors?
Brian Schmitz
11,167 PointsBrian Schmitz
11,167 PointsThanks, good point. I got these two messages in the javascript console:
Failed to load resource: the server responded with a status of 404 (Not Found) link across from the error: http://web-sa2edmjg87.treehouse-app.com/path/to/instafeed.min.js.
Uncaught ReferenceError: Instafeed is not defined link across from the error: web-sa2edmjg87.treehouse-app.com/:129
I double checked the code that I pasted above to make sure it matched what the documentation noted. Do you see anything by chance that might be causing these errors? I'm assuming it has to do with the code I have inside the script tags there. Is that right? When I downloaded the Instafeed code that is in my js file on workspaces, it gave me a line of code. I just saved that and inserted into the js file by "uploading file".
Thanks again for the help, Brian
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherBrian Schmitz
Looks like the problem is the 404 error. The instafeed.js file isn't loading to the web page. Make sure you have the correct path to the instafeed.js file. Did you upload that file to workspaces? To make it easy -- just put that file in the same folder as your web page, then link to it with the script tag like this:
<script src="instafeed.min.js"></script>
Brian Schmitz
11,167 PointsBrian Schmitz
11,167 PointsFor some reason I cant write in any code or copy and paste my code over from workspaces, it just removes it when I post the comment. How are you adding code like you did above? Then I can show you what I have, I think that might be easier.
But yes I do have a file in my JS folder in workspaces called instafeed.min.js, and I basically copy and pasted from the instafeed documentation the following:
<script type="text/javascript" src="path/to/instafeed.min.js"></script>
Thanks for your time, Brian
Brian Schmitz
11,167 PointsBrian Schmitz
11,167 PointsIt removed the script tags I tried to copy and paste above. Not sure how to get code to post here, like you have done. That makes it much easier.
Thanks again
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherBrian Schmitz
Check out this forum post: https://teamtreehouse.com/forum/posting-code-to-the-forum
Brian Schmitz
11,167 PointsBrian Schmitz
11,167 PointsThanks, that helps. So in my workspaces I do have a JS folder with the following files:
bootstrap.min.js / bootstrap.min / instafeed.min.js
Then in my index.html home page I have the following code inside script tags at the bottom of my page:
See any issues there? Could it be that I am not connecting the bootstrap JS files correctly? I essentially copy and pasted the instafeed code above from the instafeed site.
When I clicked the "download" button to download the instafeed file from their home page this is the code that was given, so the following code would be inside my instafeed.min.js file:
// Generated by CoffeeScript 1.3.3 (function(){var e,t;e=function(){function e(e,t){var n,r;this.options= {target:"instafeed",get:"popular",resolution:"thumbnail",sortBy:"none",links:!0,mock:!1,useHttp:!1};
Thanks for the help, Brian