Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial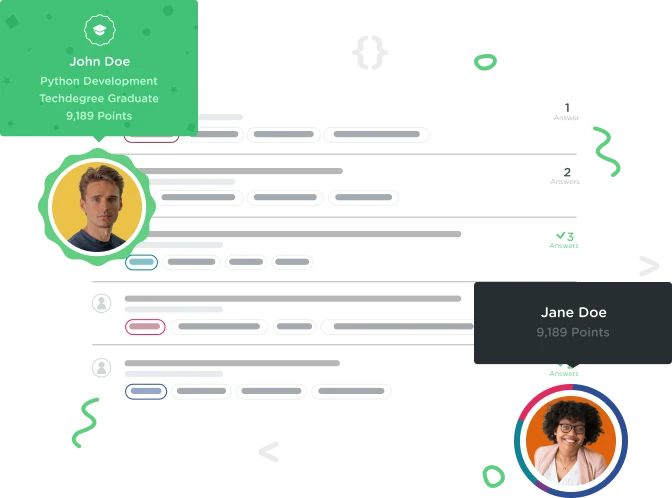

Joseph Ostovich
1,645 PointsAdding to the "Fun Facts" App?
Sometimes when you press the "Show another fun fact" button, the app will randomly generate the same exact fact you just viewed. Is there any way to make it where the facts wont appear again, until all of the facts of been viewed once?
2 Answers
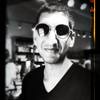
Taha Abbasi
12,865 PointsHi,
I was running into this too, and implemented a solution where the next Random Number is not the same as the last random number used. Which guarantees that the same fact will not be displayed right after the one you are viewing right now.
I also am holding my data in an ArrayList instead of just a regular Array, because I can add to and remove the size of the ArrayList later, from the web for example, but this is not related to your question, if you have questions about how I am doing this let me know.
FactBook class example: For your question, I first declared a member variable called:
private int mPreviousRandomNumber;
Then I did the below with my RandomNumber generator
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(mFactArray.size());
while(randomNumber == mPreviousRandomNumber) {
randomNumber = randomGenerator.nextInt(mFactArray.size());
}
mPreviousRandomNumber = randomNumber;
return mFactArray.get(randomNumber);
I basically generate a random number with:
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(mFactArray.size());
Then I check if this generated number is equal to the previous random number stored in mPreviousRandomNumber. If yes then I generate a new number in a while loop until the random number generated is different from the previous number generated.
while(randomNumber == mPreviousRandomNumber) {
randomNumber = randomGenerator.nextInt(mFactArray.size());
}
Once I have a new random number that is not equal to the previous. I then update the variable mPreviousRandom number to store the number I just generated to be used for checkin in the next iteration. and then I return this number
mPreviousRandomNumber = randomNumber;
return mFactArray.get(randomNumber);
This is not exactly what you are looking for in regards to the fact not repeating until all the facts are displayed, but you can use this same idea to modify the while loop a bit and accomplish what you are looking for.
Good luck!
Full FactBook code for reference:
package com.abbasiindustries.tahaabbasi.funfacts;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Random;
/**
* Created by tahaabbasi on 4/24/15.
*/
public class FactBook {
public ArrayList<String> mFactArray;
private int mPreviousRandomNumber;
public String getFact() {
// Storing facts in an Array
mFactArray = new ArrayList<>();
mFactArray.add("A quarter has 119 grooves on its edge, a dime has one less groove");
mFactArray.add("Mark Twain didn't graduate from elementary school.");
mFactArray.add("Slugs have 4 noses.");
mFactArray.add("The State of Florida is bigger than England.");
mFactArray.add("Ants stretch when they wake up in the morning.");
mFactArray.add("More people use blue toothbrushes than red ones.");
mFactArray.add("If you want something done ask someone busy.");
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(mFactArray.size());
while(randomNumber == mPreviousRandomNumber) {
randomNumber = randomGenerator.nextInt(mFactArray.size());
}
mPreviousRandomNumber = randomNumber;
return mFactArray.get(randomNumber);
}
}
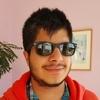
faraz
Courses Plus Student 21,474 PointsThe solution I implemented uses Collections.shuffle which mixes the order of the array before iterating through and printing all of the facts in the array. It also lets you start over and monitors the amount of restarts. Hope this helps you better understand a method of randomizing the order of each fact in the fact list without repeating any fact.
public int currentFact = 0;
public int restarts = 0;
public ArrayList<String> facts = new ArrayList<String>( Arrays.asList(
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built."));
public int listSize = facts.size();
@Override
protected void onCreate(Bundle savedInstanceState) {
final String TAG = FunFactsActivity.class.getSimpleName();
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
final TextView factLabel = (TextView) findViewById(R.id.factLabel);
final Button newFact = (Button) findViewById(R.id.newFact);
Collections.shuffle(facts);
Toast shuffleToast = Toast.makeText(getApplicationContext(), "Facts have been shuffled!", Toast.LENGTH_LONG);
shuffleToast.show();
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
Random randomGenerator = new Random();
newFact.setText("Show me a new Fun Fact!");
if (currentFact < listSize) {
factLabel.setText(facts.get(currentFact));
currentFact++;
}
else {
Log.v(TAG, listSize + " facts retrieved! No more facts available! Restart? Currently " +
restarts + " restarts!");
factLabel.setText("No more facts available!");
newFact.setText("Start Over!");
currentFact = 0;
Collections.shuffle(facts);
restarts++;
}
}
};
newFact.setOnClickListener(listener);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_fun_facts, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
Taha Abbasi
12,865 PointsTaha Abbasi
12,865 PointsP.S. I applied this to colors too.