Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial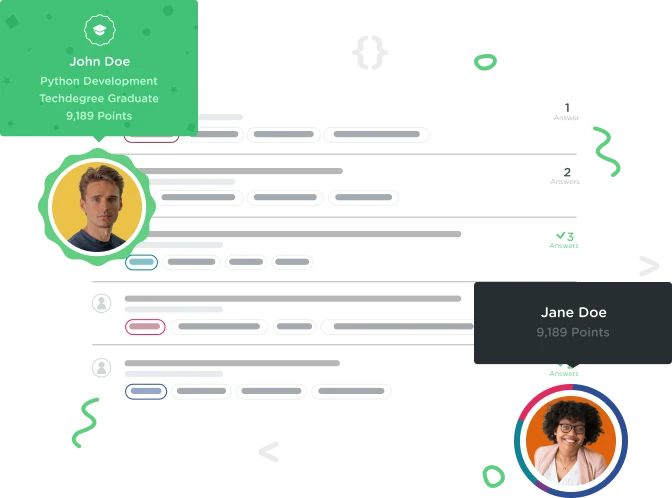
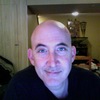
Charles Harpke
33,986 PointsAdding Validation to todo items error in testing
run this test bin/rspec --format=documentation spec/features/todo_items/create_spec.rb, and here is my result: Failures:
1) Adding todo items displays an error with content less than 2 characters long
Failure/Error: expect(page).to have_content("There was a problem adding that todo list item.")
expected to find text "There was a problem adding that todo list item." in "There was a problem adding that todo list item"
# ./spec/features/todo_items/create_spec.rb:41:in block (3 levels) in <top (required)>'
# ./spec/features/todo_items/create_spec.rb:40:in
block (2 levels) in <top (required)>'
2) Adding todo items displays an error with no content
Failure/Error: expect(page).to have_content("There was a problem adding that todo list item.")
expected to find text "There was a problem adding that todo list item." in "There was a problem adding that todo list item"
# ./spec/features/todo_items/create_spec.rb:30:in block (3 levels) in <top (required)>'
# ./spec/features/todo_items/create_spec.rb:29:in
block (2 levels) in <top (required)>'
here is my create spec file:
require 'spec_helper'
describe "Adding todo items" do
let!(:todo_list) { TodoList.create(title: "Grocery list", description: "Groceries" ) }
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
click_link "New Todo Item"
fill_in "Content", with: "Milk"
click_button "Save"
expect(page).to have_content("Added todo list item.")
within("ul.todo_items") do
expect(page).to have_content("Milk")
end
end
it "displays an error with no content" do
visit_todo_list(todo_list)
click_link "New Todo Item"
fill_in "Content", with: ""
click_button "Save"
within("div.flash") do
expect(page).to have_content("There was a problem adding that todo list item.")
end
expect(page).to have_content("Content can't be blank")
end
it "displays an error with content less than 2 characters long" do
visit_todo_list(todo_list)
click_link "New Todo Item"
fill_in "Content", with: "1"
click_button "Save"
within("div.flash") do
expect(page).to have_content("There was a problem adding that todo list item.")
end
expect(page).to have_content("Content is too short")
end
end
and my new.html.erb:
<%= form_for [@todo_list, @todo_item] do |form| %>
<% if @todo_item.errors.any? %>
<div id="error_explanation">
<h2><%= pluralize(@todo_item.errors.count, "error") %> prohibited this todo item from being saved:</h2>
<ul>
<% @todo_item.errors.full_messages.each do |message| %>
<li><%= message %></li>
<% end %>
</ul>
</div>
<% end %>
<%= form.label :content %>
<%= form.text_field :content %>
<%= form.submit "Save" %>
<% end %>
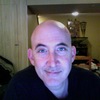
Charles Harpke
33,986 PointsHere is my todo_item.rb class TodoItem < ActiveRecord::Base belongs_to :todo_list
validates :content, presence: true,
length: { minimum: 2 }
end
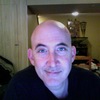
Charles Harpke
33,986 PointsI found it....argh I expected to find text "There was a problem adding that todo list item." in "There was a problem adding that todo list item"
I was expecting that the sentence ends with a period, but apparently it does not.
Charles Harpke
33,986 PointsCharles Harpke
33,986 Points