Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial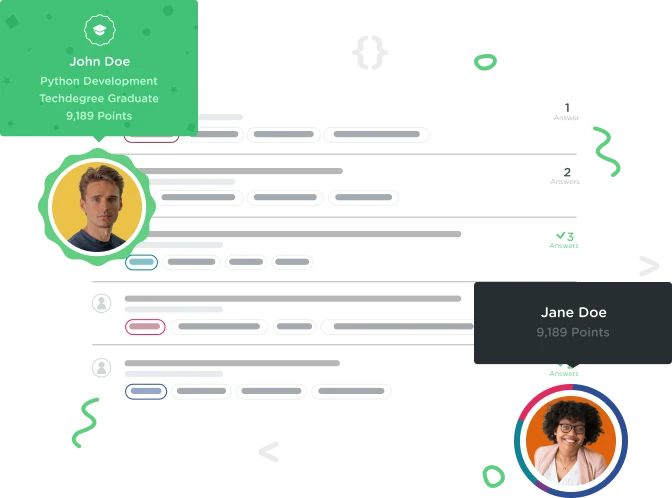

Jose De La O Hernandez
9,857 PointsAdding (value) to each key on an array!
So I have an array (say $array(1,2,3,4,5)). I need to write a function that will add (say 1) to each element so when I put $array into my "addonefunction" my return value when I print_r(addonefunction(array)) I will get $newarray(2,3,4,5,6). Can anyone guide me in the right direction as to how to do this?
5 Answers
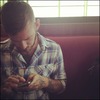
Erik McClintock
45,783 PointsJose,
The issue lies within the foreach loop, as Shawn stated; your code is getting cut short by having the return statement inside the loop (remember, "return" breaks the loop).
Beyond that, though, if you simply move the "return $value" outside of the foreach, you're still only going to retrieve one value, though this time it will be the last value in the array.
I suggest doing one of the following:
1) Inside your function, create a new array ($newArray), then inside the foreach, for each value, array_push that value into your new array, and then after the foreach, return your new array.
2) Use the & symbol in your foreach declaration, like so:
foreach($arr as &$value) {
//code goes here
}
...the & symbol passes a variable by reference so that it can be modified. Read more here:
http://php.net/manual/en/language.references.pass.php
Whichever method you choose to go with, you still need to move your return statement outside of the foreach loop. Also, make sure you're returning the $arr, not the $value.
Let me know if you need to see some examples for clarification.
Hope this helps!
Erik
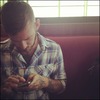
Erik McClintock
45,783 PointsJose,
It sounds like you're looking for the "foreach" loop! Take a look at the documentation found at the following link and see if this will do the trick for you:
http://php.net/manual/en/control-structures.foreach.php
You'll use the foreach loop to iterate each value in your array, and for each iteration, increment the current value by one ($value++).
Hope this helps!
Erik

Jose De La O Hernandez
9,857 PointsHello! Thanks for the quick response. Unfortunately, however, I seem to be missing an important step.... here is what my code looks like:
<?php
function myArrayFunc($arr) {
foreach($arr as $value) {
$value = $value + 1;
return $value;
}
}
$myArray = array(5, 3, 2, 5, 7);
echo "My array looks like:\n";
print_r($myArray);
echo "and if I add one to each of them, it looks like:\n";
print_r(myArrayFunc($myArray));
?>
OUTPUT:
My array looks like: Array ( [0] => 5 [1] => 3 [2] => 2 [3] => 5 [4] => 7 ) and if I add one to each of them, it looks like: 6
Sooo, I am able to print my original array but when I try printing the second one, I simply get 6.
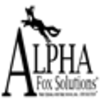
Shawn Gregory
Courses Plus Student 40,672 PointsJose,
Looking at your code, by you having your function's return inside of the foreach loop, the loop will only run once then exit out of the function. You need to place your return outside of the loop for it to work. That way it'll run through the loop then afterwards, exits out of the function. Try it and see if it works.
Cheers!

Jose De La O Hernandez
9,857 PointsThank you very much for the help I was able to get this code working d(^.^)b

Jose De La O Hernandez
9,857 PointsSooo.. now I am working on another problem and this one is a little bit more in depth.
My first problem was this creating a function that, when given a date in a certain format, will return it in a defined format. Here is my answer to that:
<?php
function myDateFormatter($dateStr){
$newDate = date("Y-m-d", strtotime($dateStr));
return $newDate;
}
$date1 = "May 13, 2007";
echo "The date $date1 formatted with my function is " . myDateFormatter($date1) . "\n";
// The output should look like:
// The date May 13, 2007 formatted with my function is 2007-05-13
// Another example
$date2 = "january 1 2014";
echo "The date $date2 formatted with my function is " . myDateFormatter($date2);
// The output should look like:
// The date january 1 2014 formatted with my function is 2014-01-01
?>
The second problem was the one you guys helped me with.
Now the third problem is this:
<?php
function myDateFormatter($date) {
// COPY YOUR myDateFormatter() FUNCTION CODE FROM CHALLENGE #1
}
function formatDateArray($dateArray){
// PUT YOUR NEW CODE HERE!
// You need to return an array that contains all of the dates in the
// $dateArray array formatted using the myDateFormatter() function above.
}
/*
DON'T TOUCH THE CODE BELOW
Your output should look like:
My date array looks like:
Array
(
[0] => may 30th
[1] => jan 1
[2] => May 13, 2007
)
and if I format them, it looks like:
Array
(
[0] => 2014-05-30
[1] => 2014-01-01
[2] => 2007-05-13
)
*/
$dateArray = array("may 30th", "jan 1", "May 13, 2007");
echo "My date array looks like:\n";
print_r($dateArray);
echo "and if I format them, it looks like:\n";
print_r( formatDateArray($dateArray) );
Please note that I DO NOT want the answer but a little help in guiding as to how to begin this.... By the looks of if, I assume these problems were designed to build one into the other. Is that a correct assessment?