Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial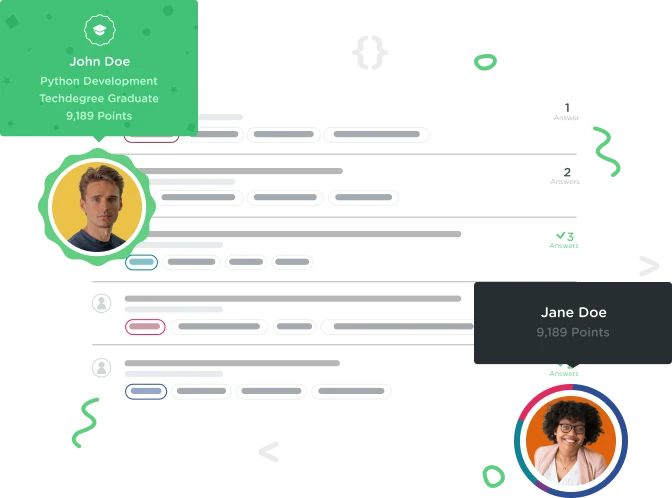

Jeffrey Robinson
2,481 Pointsadd_item
I've tried a number of solutions to this problem but there is so little feedback I'm not even sure if I'm even reading it right.
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def __init__(self, item):
super().add_item(item)

Jeffrey Robinson
2,481 PointsGood evening Dave - thank you for taking the time to answer my question. The question is:
"Now override the add_item method. Use super() in it to make sure the item still gets added to the list."
I feel like a dummy, but I don't even know what that means. Override the add_item method?
1 Answer
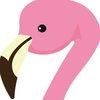
Dave StSomeWhere
19,870 PointsHey hey Jeffery,
The concept of inheritance is very important and you should probably do some follow up searches on it and go through the video again until it makes sense. No use to moving on until you got this .
In task 1 you created a sub class of Inventory
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
# specify a class in the parenthesis and you have a subclass
class SortedInventory(Inventory):
pass
The SortedInventory class at this point is the exact same as Inventory - because - it inherited everything from Inventory, which is two methods one called __init__()
and one called add_item().
Now we have the ability to add additional properties (variables) or methods (functions) to our subclass. We also have the ability to say. Hey I want to do something different with one of the two methods inherited from Inventory - this is called overriding the method. All we need to do to override the method is to define in our subclass. Like you did with __init()__
and should of done with add_item()
. When you override a method your subclass method is executed instead of the base class method.
Once you override the method, you might still want to execute the base class method in addition to executing your subclass features. This is where super()
comes into play. Super calls the base class method. Hope that makes sense - just let me know
What it should look like is:
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self, item): # override add_item
super().add_item(item) # call the Inventory add_item() method
# put SortedInventory specific code here
Does that make any sense?

Jeffrey Robinson
2,481 PointsGood morning Dave,
Thank you very much. Yes, that totally makes sense. I was not picking up what it meant to "override" from the video. Thank you for your help.
Jeff
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsAre you stuck on task 2?
Double check the requirement - it looks like you are attempting to override the init() method - is that the ask?