Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial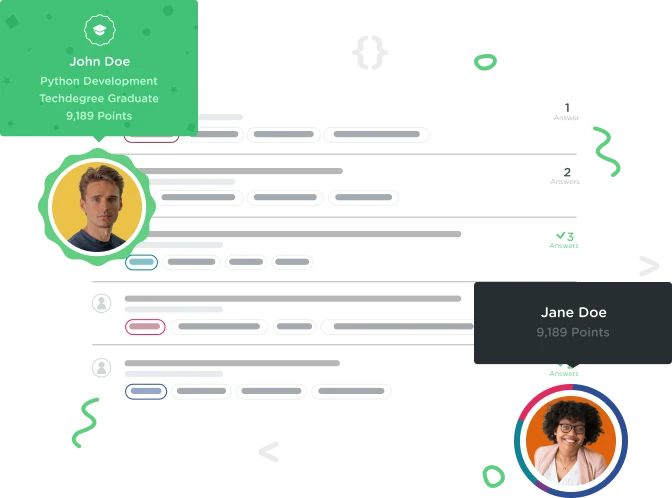

Eric VanLenten
15,423 PointsAdditional functionality: Adding and/or updating user info in database.
Here is the /register post route code which will take the user's information and insert it into Mongo. The User.create method is used to create a new user in the database.
// POST /register
router.post('/register', function(req, res, next) {
if (req.body.email &&
req.body.name &&
req.body.favoriteBook &&
req.body.password &&
req.body.confirmPassword) {
// confirm that user typed the same password twice
if (req.body.password !== req.body.confirmPassword) {
var err = new Error('Passwords do not match.');
err.status = 400;
return next(err);
}
//create object with form input
var userData = {
email: req.body.email,
name: req.body.name,
favoriteBook: req.body.favoriteBook,
password: req.body.password
};
// use schema's 'create' method to insert document into Mongo
User.create(userData, function (error, user) {
if (error) {
return next(error);
} else {
req.session.userId = user._id;
return res.redirect('/profile');
}
})
} else {
var err = new Error('All fields required.');
err.status = 400;
return next(err);
}
});
After the user is registered and logged in, I want to update his information in the database. As an example, let's say I want to record the number of times the user has visited the 'Contact' page of the website. So the first time he visits the Contact page, a new field will be created in the database for the user with the value of 1. Every time he visits the 'Contact page afterwards, the value will be updated. (Would I need to make any modifications to the UserSchema?) Any help would be greatly appreciated.
Code for /contact route:
// GET /contact
router.get('/contact', function(req, res, next) {
return res.render('contact', { title: 'Contact' });
});