Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial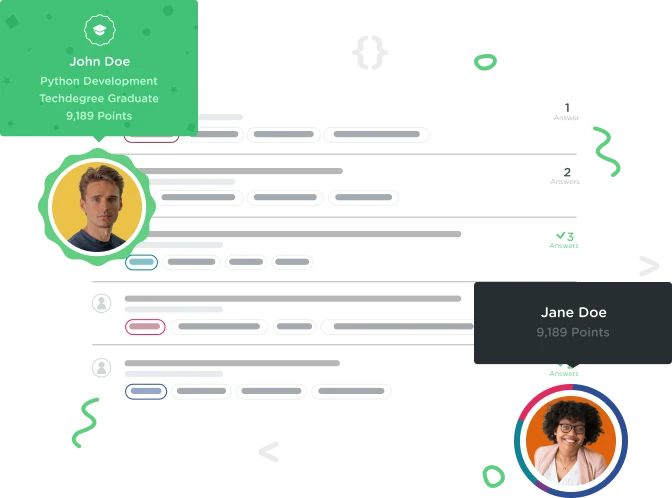

Al3n MicL
6,364 Pointsadd_list code challenge (1 of 2)
Don't know why I get this error returned from the editor, is my code missing something:
# Note: both functions will only take *one* argument each.
# add_list([1, 2, 3]) should return 6
def add_list(li):
li = []
total = int()
for item in li:
total += li[item]
return total
#Error: add_list didn't return the right answer. Got 0 when adding [1, 2, 3], expected 6.
2 Answers
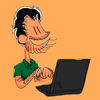
Juan Martin
14,335 PointsHello my friend.
As I see, you have 3 mistakes in your code:
- You shouldn't initialize li = [] as you're getting its value directly from the function itself. Doing li = [] will reset the value.
- total must have a value, and that's 0 because you'll use it to sum all the numbers inside the list. There's no need to say it's an int as giving it the value 0 Python will know is an integer.
- Inside the for loop, you have to do the sum with the item because the item is the value (number) inside the list (your li variable).
Here how it should look:
def add_list(li):
total = 0
for item in li:
total += item
return total
Hope this helps :)

Charlie L.
10,120 PointsJust to add to Juan's awesome answer. I wanted to further explain what your code is currently doing.
def add_list(li):
li = []
So what you've done here is taken a list and then changed the list. So if you were given a list of [1, 2, 3], "li = []" now makes [1, 2, 3] -> [] which essentially is an empty list.
total = int()
The "int()" method needs to be used on a value. I think you wanted to set it to be 0 which you could easily set it by saying total = 0.
for item in li:
total += li[item]
return total
The only thing wrong with this part is the "li[item]" part. You're using indexes instead of the actual item in the list. In a list of [1, 2, 3] when you use li[item], you're pulling the 1st index, then the second index, and then the third (which actually doesn't exist in this list). If you want more information, look up splicing.

Al3n MicL
6,364 PointsYes, thanks for your further clarifications, especially your third example. For in loops do implicit iteration so using the member accessor for the indexes in the list is not necessary.
Thanks for your input.
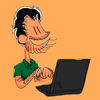
Juan Martin
14,335 PointsExcellent explanation my friend and also thanks for pointing out my answer as awesome :)
Al3n MicL
6,364 PointsAl3n MicL
6,364 PointsAhh, I now see the error in my syntax! Didn't realize that declaring li as a blank list inside the function would re-init whatever list arguments were passed into it. Also my for loop declaration was a bit off. Little mistakes like this can lead to unnecessary frustration so thanks for pointing them out.
Cheers!
Juan Martin
14,335 PointsJuan Martin
14,335 PointsI'm glad I could help you my friend :)