Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial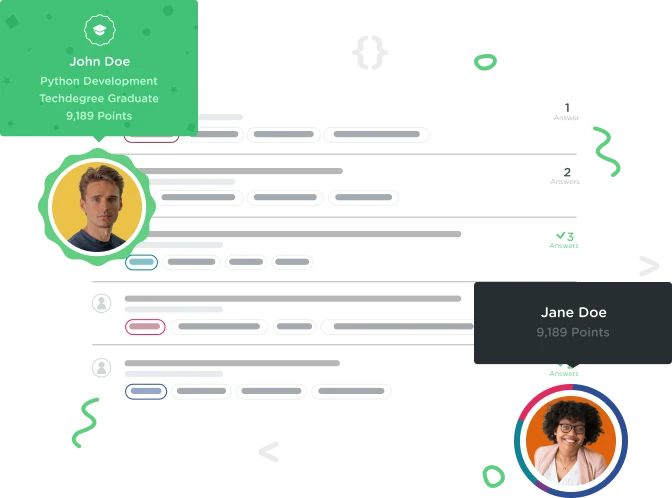
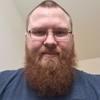
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsAddressBook OOP C#
Hi,
I just finished the C# objects course and now I'm trying to make the "Address Book" he suggested at the end of the course.
I'm having the problem that I can't remember much of it since I never really tried the OOP seriously but now I want to learn it - I need to re-watch the videoes again for sure.
For now I only created the intro to the Address Book but I'm having this feeling that I'm doing it wrong or it could be done better - I would love if someone could give me some input (I'm sure that Helpers.AddNewPerson can be done better but I have no idea how) :-)
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AddressBook
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Welcome to your personal 'Address Book'.");
Console.WriteLine("\nHere is a quick instruction how to use the 'Address Book':");
Helpers.Instructions();
while (true)
{
Console.Write("\nPlease enter your instruction: ");
var input = Console.ReadLine();
if(input.ToLower() == "quit")
{
break;
}
else if(input.ToLower() == "help")
{
Helpers.Instructions();
}
// Adding a seperate if/else if for the "new", "search" and "delete" instructions
if(input.ToLower() == "new")
{
Console.Clear();
Helpers.AddNewPerson();
}
}
}
}
}
Helpers.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AddressBook
{
class Helpers
{
public static void Instructions()
{
Console.WriteLine("Type 'new' to add a new person to your 'Address Book'.");
Console.WriteLine("Type 'quit' to exit the program.");
Console.WriteLine("Type 'help' to get the instructions again.");
}
public static void AddNewPerson()
{
Console.Write("First Name: ");
var firstName = Console.ReadLine();
Console.Write("Last Name: ");
var lastName = Console.ReadLine();
Console.Write("Address: ");
var address = Console.ReadLine();
Console.Write("Phone Number: ");
var phoneNumber = Console.ReadLine();
Console.Write("Email: ");
var email = Console.ReadLine();
Console.WriteLine("\n" + firstName + "\n" + lastName + "\n" + address + "\n" + phoneNumber + "\n" + email);
}
}
}
1 Answer

Diar Selimi
1,341 PointsI looks perfect :D just keep going, the logic is good as the part of the code you always can make it better.