Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial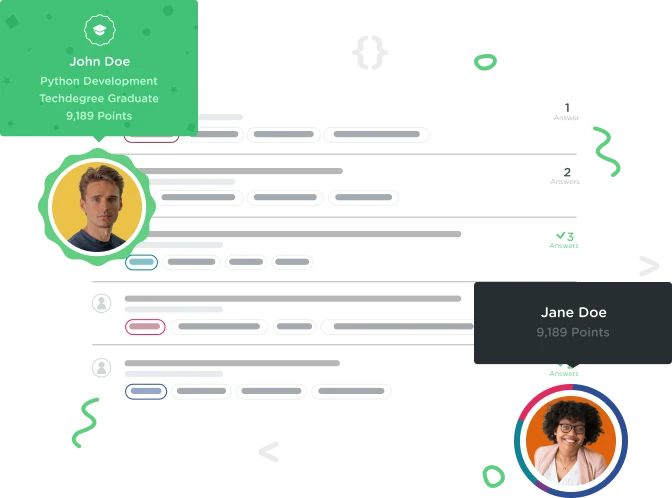

Chris Butcher
12,198 PointsAdmin editing other user details - Rails 4.1 + latest Devise + latest Simple Form
Hi everyone, I am writing my first Ruby project and I've dived right in the deep end, so apologies if my lack of deep Rails understanding is showing through.
I am trying to create a user management system, so admins can edit the details of users in the system without being logged in as them. I understand Devise does not have such functionality built in and that's what I'm trying to hack together myself.
I am nearly there. I have a 'Manage Users' page that successfully lists users in the system along with an 'Edit User' link next to each one:
app/views/admin/manage_users.html.erb
<h2>Manage Users</h2>
<p>As an administrator, you can add new users to the system. You can also edit or delete existing ones.</p>
<p>
<% @users = User.all %>
<ul class="users">
<% @users.each do |user| %>
<li><%= user.id %> <%= user.first_name %> <%= user.second_name %> <%= user.email %> <%= link_to "Edit User", edit_user_details_path(id: user.id) %></li>
<% end %>
</ul>
</p>
app/views/admin/edit_user_details.html.erb
Clicking on 'Edit User' correctly renders an 'Edit User Details' page according to the user selected (using id to identify):
<h2>Edit user details</h2>
<p>
<%= @user.first_name %>
<%= @user.second_name %>
<%= @user.email %>
</p>
I now want to implement a form (using simple form) on the page shown above that allows the admin to make changes to the user's first name, second name, email, and an option to reset password. It should ideally behave like Devise's built in 'edit registrations' page but change a user who is not currently linked in. I'm completely stumped as to how I will achieve this. Any help appreciated.
Here is my Admin controller file for context:
app/controllers/admin_controller.rb
class AdminController < ApplicationController
def manage_users
end
def edit_user_details
@user = User.find(params[:id])
end
end
3 Answers
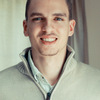
Andrew Lincoln
8,880 PointsThis is a complex as I am not as seasoned of a rails dev as some of our patrons at treehouse. Have you looked for any helper methods in the rails API that could help you solve your challenge. I also suggest getting better understanding of the rails architecture before diving to the deep end. the MVC pattern etc..

Chris Butcher
12,198 PointsHi Andrew, Thanks for your help, it is a difficult problem. In the end I uninstalled Devise and wrote my own user model from scratch since augmenting Devise and a separate user model to handle CRUD actions was proving too complicated at this stage.
Thanks again
Chris
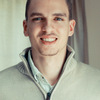
Andrew Lincoln
8,880 PointsNo problem Chris!
All those rails generators can really confuse new rails developers like ourselves haha. Good luck with your project!