Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial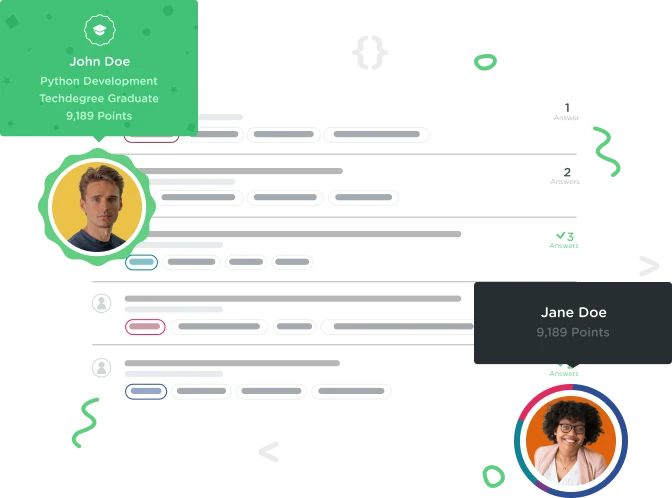

cbcbcb
16,560 PointsAdvice for dealing with frameworks and/or scripts etc that utilize the Python 2.x APIs as opposed to Python 3.x APIs?
I figured it might be helpful to provide a little information regarding my little knowledge of the differences between some of the functions contained within the core libraries/modules of Python 2.x and 3.x with the purpose of making it easier to assist in pointing me in the right direction as I know this isn't considered a reasonable question for a QA forum on a programming language.
standard output
I understand the print is treated as a function in Python 3.x as opposed to a command (it is my understanding that it is referred to as a command)
Python 2.x
Standard output example:
print "abcd";
Python 3.x
Standard output example:
print("abcd");
Unicode Support
Python 2.x
It is my vague and uncertain understanding that Python 2.x handles encoding differently and requires a built-in function to convert input and/or output when dealing with UTF-8 encoded data. Python 3.x
** It is to my understanding ,but very vague and uncertain, that python 3 source code is by default encoded in utf-8 format and/or all output is encoded in utf-8 format as well.**
Main Question
Do you have any advice on overcoming the fact that most of the tutorials and guides all provide instruction using 2.x without switching to 2.x?
- Books?
- Videos?
- Third-party modules?
- General advice?
- You're out of luck because that is the negative affect of using 3.x instead of 2.x?
Side Questions
Is a command in Python referring to a keyword as you've shown in previous videos?
For example:
a_string = "abcd";
del a_string;
Is the source code in Python 3.x encoded in utf-8 or is it the output or is it the input or both I/O or all three?
Is it the input or output that needs to be converted to utf-8 if at all and is it necessary?
Is the procedure I've seen being used extensively in Python 2.x for inserting string values into discrete strings by using a parameter keyword represented as a '%' which appears to create a pointer to another '%' which immediately follows the string object separated by a space which is then separated by a space followed by what appears to be the variable being referenced as the value to be passed in as the parameter also supported in Python 3.x?
a_string = "e"
print "abcd %" % a_string;
I've only used the following example in python 3.x which seems a bit cumbersome in terms of the amount needed to type as opposed to the following example listed for Python 2.x:
a_string = "e";
print("abcd{}".format(e));
Is there a better way to pass values into a string as a parameter when printing it at runtime in the event the Python 2.x example is not supported in Python 3.x?
2 Answers
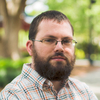
Kenneth Love
Treehouse Guest TeacherOK, lots of stuff up there so I'll try to answer as best I can. Let me know if I miss anything.
How to learn more Python since most guides are based on Python 2 instead of Python 3
This isn't actually that bad. You have two choices, as I see it.
- Translate the code examples from Python 2 to Python 3. In most cases, I imagine this will be mostly turning
print
intoprint()
and a few other syntax changes. You'll possibly have to redo some imports, as well, as some things moved between 2 and 3. - If you have Python 2 installed as well, go ahead and learn using 2. Ideally, you'd use the
from __future__ import
s to be able to write your Python 2 code like it was Python 3 so that later conversion would be easier.
As for the __future__
items, the ones you'll most likely use will be division
, print_function
, and absolute_import
. You can see the full list of them at the bottom of this page.
Is print
a command or keyword
print
in Python 2 is technically a statement or keyword. I don't think I've heard it called a "command" before but that's an OK way to think of it. The problem is that it's the only core-level functionality that has a statement dedicated to it. All of the others are functions, which is why Python 3 made print()
a function.
Python 3 and unicode
Python 3's source code (speaking only about CPython) is a mix of Python code and C. I don't know that any of it is written expressly in unicode, but the parts written in Python definitely could be since Python 3 allows unicode pretty much anywhere.
Using Python 3's default strings should mean that you never have to explicitly convert anything to UTF-8. That's the assumed default. If you need to turn a string into something else, say ASCII or UTF-16, there are methods to do so. I'd suggest reading some of the Python guide to unicode
sprintf and Python 2 and 3
The method you've been seeing, something like "Hello %s" % name
is often referred to as sprintf, or string print formatting. It's still very viable in Python 3 but isn't as obvious or clean as using str.format()
, in my opinion. You are building placeholders with the %s
(or %d
, etc) that are then filled in with values after a %
operator. If there's more than one value, you have to pass in a tuple or list w/ the correct number of positions.
One of the main reasons that I don't teach about sprintf is the fact that it uses the %
operator just like modulo math does (5 % 2
gives back 1). I feel like this is a confusing doubling of functionality for a single operator and just leads to extra headache.
OK, I hope that answered most (all?) of your questions. If not, feel free to follow up and I'll answer more.

cbcbcb
16,560 PointsYeah actually that % syntax was confusing but now I'm familiar as I remember they added printf to Java at one point to emulate the functionality of print formatting in C. Thanks for the feedback. Awesome!
Now that I you said so I reread my questions and forgot one that I discovered I had while asking another question.
Are strings objects in python or primitive values when they are not assigned a reference variable?
ex:
print("is this an object?";
Really appreciate it.
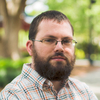
Kenneth Love
Treehouse Guest TeacherPretty much everything in Python is an object, whether it's assigned to a variable or not.
James Gill
Courses Plus Student 34,936 PointsJames Gill
Courses Plus Student 34,936 PointsKenneth, that's actually very helpful to me, too. I've got a related question: most Macs have Python 2.7.x installed, not 3.x. What do you recommend as the most painless way to upgrade to 3.x.? Will this require having two versions installed?
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherJames Gill The download at python.org is probably the most painless. You'll end up with a
python3
executable alongside your existingpython
one. I don't see a problem with this since it makes your execution more explicit.What I do, since I often need to check multiple versions of Python (even with the 2.x and 3.x branches) is to use pyenv, which lets you install Python versions per-directory or globally. This isn't for the faint of heart or Python dabblers, though, so just go with the DMG.