Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial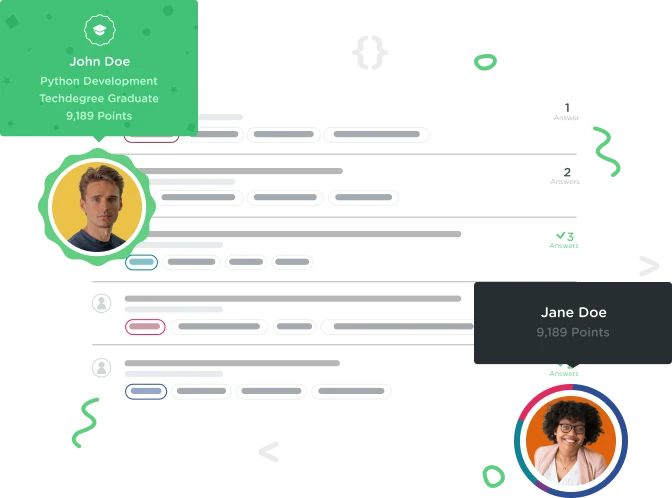
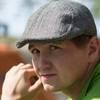
AJ Longstreet
Treehouse Project ReviewerAdvice on efficiency. Python
I just finished a number guessing game similar to the video. It works but, I was just wondering if any of you professional programs have any advice for me. Something I could have or should have done differently or better?
Thanks.
EDIT
I added a try: to catch the ValueError if someone tried to guess with a letter or something silly.
import random
import numbers
guess_count = 0
guess = 8888
answer = 9999
finished = 0
game = "y"
while True:
print("Number Guessing Game")
game = input("Would you like to play? y or n? >> ")
if game == "y":
finished = 0
answer = random.randint(1,10)
while (finished == 0) and (guess_count <= 5):
guess = int(input("Guess a whole number between 1 and 10 > "))
if (guess >= 1) and (guess <= 10) and (finished != 1):
if guess == answer:
print("That was the right answer!")
finished = 1
elif guess_count == 4:
print("You ran out of guesses!")
break
elif guess < answer:
print("That guess is too low")
guess_count += 1
elif guess > answer:
print("That guess is too high")
guess_count += 1
else:
print("That is not a number between 1 and 10")
break
else:
break
print("Goodbye!")
1 Answer
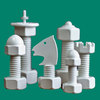
Steven Parker
231,141 PointsI found two bugs and have two suggestions:
- you need to reset guess_count at game start
- bad inputs still break it (and I didn't see any "
try
" in the code) - you don't need to check if "
finished != 1
" since the loop checks that it is 0 - you could eliminate finished completely and just break on a right answer