Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial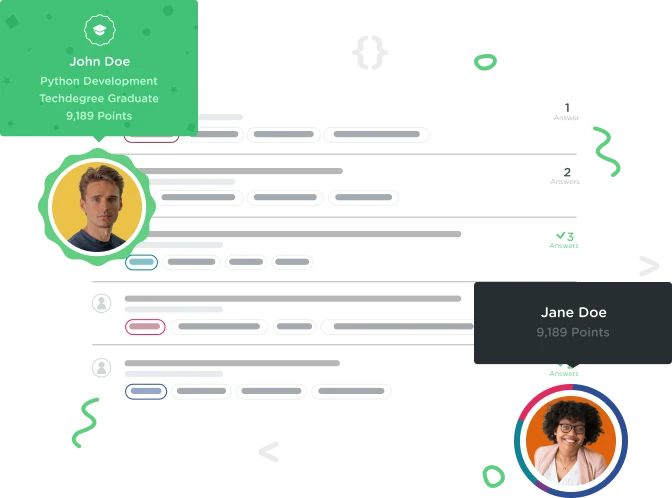
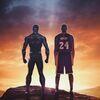
Ruben Sarkisyan
8,473 PointsAfter creating the todo list, I try to add an item and it appends successfully but doesn't show the caption of the task
//If someone would like to compare their final version of the app.js file, please feel free. I would assume the problem lies in the addTask function
//Problem: User interaction doesn't provide desired results. //Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById('new-task'); //new-task var addButton = document.getElementsByTagName('button')[0]; //first-button var incompleteTasksHolder = document.getElementById('incomplete-tasks'); //incomplete-tasks var completedTasksHolder = document.getElementById('completed-tasks'); //completed-tasks
//New Task list item var createNewTaskElement = function(taskString) { //Create list item var listItem = document.createElement('li');
//input(checkbox) var checkBox = document.createElement('input'); //checkbox //label var label = document.createElement('label'); //input(text) var editInput = document.createElement('input'); //text
//button.edit var editButton = document.createElement('button') //button.delete var deleteButton = document.createElement('button')
//Each element needs modifying checkBox.type = 'checkbox'; editInput.type = 'text';
editButton.innerTEXT = 'Edit'; editButton.className = 'edit'; deleteButton.innerTEXT = 'Delete'; deleteButton.className = 'delete';
label.innerTEXT = taskString;
//Each element needs appending listItem.appendChild(checkBox); listItem.appendChild(label); listItem.appendChild(editInput); listItem.appendChild(editButton); listItem.appendChild(deleteButton);
return listItem; }
//Add a new task var addTask = function() { console.log('Add task'); //Create a new list item with the text from #new-task var listItem = createNewTaskElement(taskInput.value); //Append listItem to incompleteTasksHolder incompleteTasksHolder.appendChild(listItem); bindTaskEvents(listItem, taskCompleted);
taskInput.value = ''; }
//Edit an existing task var editTask = function () { console.log('Edit task');
var listItem = this.parentNode;
var editInput = listItem.querySelector('input[type=text]'); var label = listItem.querySelector('label'); var containsClass = listItem.classList.contains('editMode');
//if the class of the parent is .editMode if (containsClass) { //Switch from .editMode //label text becomes the inputs value label.innerTEXT = editInput.value; } else { //switch to .editMode //input value becomes the labels text editInput.value = label.innerTEXT; } //toggle .editMode listItem.classList.toggle('editMode'); }
//Delete an existing task var deleteTask = function() { console.log('Delete task'); //When the delete button is pressed var listItem = this.parentNode; var ul = listItem.parentNode; //Remove the parent list item from the ul ul.removeChild(listItem); }
//Mark a task as complete var taskCompleted = function() { console.log('Complete task'); //Append the task list item in the #completed task var listItem = this.parentNode; completedTasksHolder.appendChild(listItem); bindTaskEvents(listItem, taskIncomplete); }
//Mark a task as incomplete var taskIncomplete = function() { console.log('Incomplete task'); //Append the task list item to the #incomplete-tasks var listItem = this.parentNode; incompleteTasksHolder.appendChild(listItem); bindTaskEvents(listItem, taskCompleted); }
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) { console.log('Bind list item events'); //select taskListItem children var checkBox = taskListItem.querySelector('input[type=checkbox]'); var editButton = taskListItem.querySelector('button.edit'); var deleteButton = taskListItem.querySelector('button.delete');
//bind editTask to edit button editButton.onclick = editTask;
//bind deleteTask to delete button deleteButton.onclick = deleteTask;
//bind checkboxEventHandler to checkbox checkBox.onchange = checkBoxEventHandler; }
var ajaxRequest = function() { console.log('AJAX request'); }
//Set the click handler to the addTask function addButton.addEventListener('click', addTask); addButton.addEventListener('click', ajaxRequest);
//Cycle over incompleteTasksHolder ul list items for (var i = 0; i < incompleteTasksHolder.children.length; i++) { //bind events to list items children (taskCompleted) bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted); }
//Cycle over completeTasksHolder ul list items for (var i = 0; i < completedTasksHolder.children.length; i++) { //bind events to list items children (taskIncomplete) bindTaskEvents(completedTasksHolder.children[i], taskIncomplete); }