Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial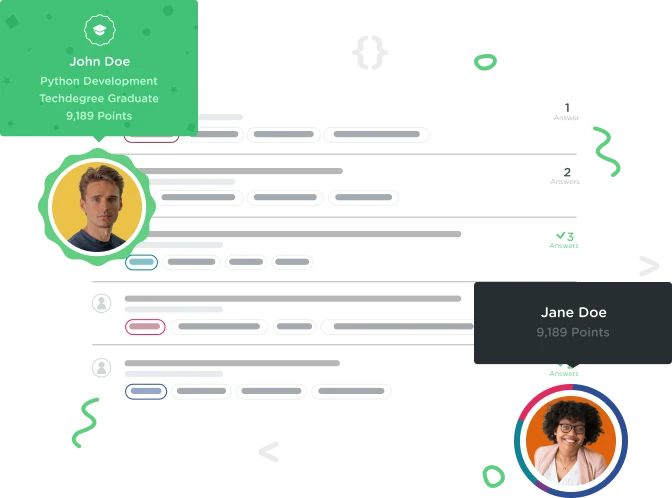

Ryan Lail
4,386 PointsAfter I add the 'try' statement, if the user enters an invalid data type how can I make my program carry on...
... at the indicated point?
Here is my code:
shopping_list = []
def show_help():
print("\nSeparate each item with a comma. ")
print("Type DONE to quit, SHOW to see the current list, and HELP to get this message. ")
def show_list():
count = 1
for item in shopping_list:
print("{}: {}".format(count, item))
count += 1
print("Give me a list of things you want to shop for. ")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "DONE":
print("\nHere's your list: ")
show_list()
break
elif new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
show_list()
continue
else:
new_list = new_stuff.split(",")
index = input("Add this at a certain spot? Press enter for the end of the list, "
"or give me a number. Currently {} items in the list. ".format(len(shopping_list))) #...to back up here
try:
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
except:
print("That wasn't a integr!")
continue #I want it to go from here...
4 Answers
Pier Yos
15,721 PointsHey Ryan,
I don't know if this is the best solution. Since continue goes back to the beginning of the while loop, I simply take it out of the while loop. I created a item_spot method so you can keep calling it when you need it.
shopping_list = []
def show_help():
print("\nSeparate each item with a comma. ")
print("Type DONE to quit, SHOW to see the current list, and HELP to get this message. ")
def show_list():
count = 1
for item in shopping_list:
print("{}: {}".format(count, item))
count += 1
def item_spot():
index = input("Add this at a certain spot? Press enter for the end of the list, "
"or give me a number. Currently {} items in the list. ".format(len(shopping_list))) #...to back up here
try:
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
except:
print("That wasn't a integr!")
item_spot()
print("Give me a list of things you want to shop for. ")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "DONE":
print("\nHere's your list: ")
show_list()
break
elif new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
show_list()
continue
else:
new_list = new_stuff.split(",")
item_spot()
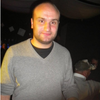
Vittorio Somaschini
33,371 PointsHello Pier.
Just to let you know, I have changed your comment into an answer.
;)
Vittorio
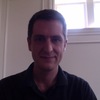
Max Hirsh
16,773 PointsThe way to allow python to keep going when an exception is raised is to use the "pass" command. I've read that the try-except-pass structure is considered a python antipattern because it allows code to keep going after a malfunction. This can make troubleshooting more difficult later on. That being said, using "pass" for the code challenge is perfectly fine.
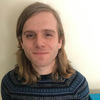
sradms0
Treehouse Project ReviewerCreating the function is more clean. Your try statement was in the wrong spot, it should be under 'if index:' The input will try to be converted to an integer if the input is anything but a number and this won't work, so here, except will give the message and continue through the loop without adding the items to the list. The user will have to add them again.
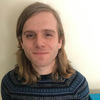
sradms0
Treehouse Project Reviewer if index:
try:
index_spot = int(index)
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot +=1
except:
print("ERROR: No characters allowed.")
for item in new_list:
shopping_list.append(item.strip())
continue
else:
for item in new_list:
shopping_list.append(item.strip())
Creating a function is more clean, but if you don't want a function.: Your try and else statements are in the wrong spot.
Ryan Lail
4,386 PointsRyan Lail
4,386 PointsYou need to scroll across on my code to see it all