Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial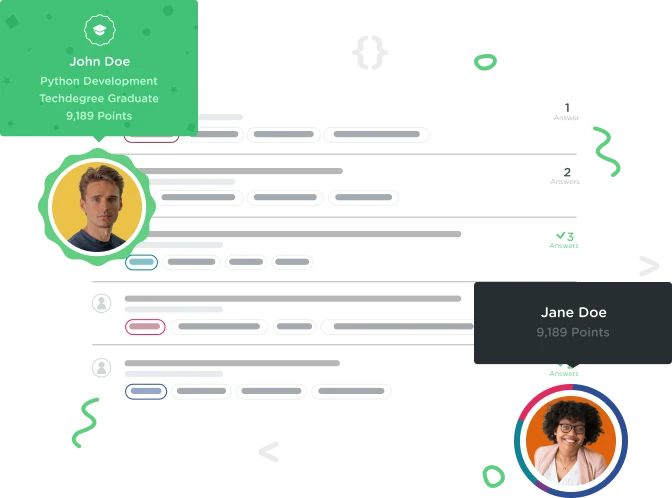

Shawna Duvall
Python Development Techdegree Student 1,706 PointsAfter raise for quantity, not a number exception prints computer error message.
I got to the end after the part where we raise an exception for the quantity being more than the tickets_remaining and get the proper message when it fires off. However, I decided to go and check the original "please enter a number" error and now that one fires off this message.
Oh no, we ran into an issue. invalid literal for int() with base 10: 'ggg'. Please try again.
How do I keep the original error message and have the second one called with the raise"?
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What is your name? ")
print("Welcome, {}! There are {} tickets remaining".format(name, tickets_remaining))
quantity = input("How many tickets would you like? ")
try:
quantity = int(quantity)
if quantity > tickets_remaining:
raise ValueError("There are only {} tickets remaining.".format(tickets_remaining))
except ValueError as err:
print("Oh no, we ran into an issue. {}. Please try again.".format(err))
else:
total = quantity * TICKET_PRICE
print("For {} tickets the total price is ${}".format(quantity, total))
confirm = input("Would you like to continue. Enter Y/N ")
if input == "Y" or "y":
print("SOLD!")
tickets_remaining -= quantity
else:
print("We are sorry to hear that, {}! Maybe next time.".format(name))
print("Sorry, we are sold out! :(")

Christian Vasquez
Python Development Techdegree Student 820 PointsWhat I did was that on line 15, I deleted the brackets and the ".format(err)" and it got rid of the error message. I tested the code to make sure it worked fine, and it did work for me.
3 Answers
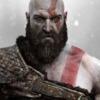
boi
14,242 PointsThe error you're getting (invalid literal for int() with base 10: 'ggg'.) is completely normal, it is the standard error handling in Python. Whenever you try to perform an int
type operation on something that is other than an int
type, you get this error.
If you want to preserve your original text error message, there is another way to do it. š
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What is your name? ")
print("Welcome, {}! There are {} tickets remaining".format(name, tickets_remaining))
quantity = input("How many tickets would you like? ")
try:
quantity = int(quantity)
if quantity > tickets_remaining:
raise ValueError š # Made change here
except ValueError as err:
if "invalid" in str(err): š # Made change here
print("Oh no, we ran into an issue. Please try again.") š # Made change here
else: š # added else here
print("There are only {} tickets remaining.".format(tickets_remaining)) š # added error msg here
else:
total = quantity * TICKET_PRICE
print("For {} tickets the total price is ${}".format(quantity, total))
confirm = input("Would you like to continue. Enter Y/N ")
if input == "Y" or "y":
print("SOLD!")
tickets_remaining -= quantity
else:
print("We are sorry to hear that, {}! Maybe next time.".format(name))
print("Sorry, we are sold out! :(")
Your code also has some additional errors which are not being caught, try entering 0 in "How many tickets would you like?"
For future code comments please use proper code format, to keep the original color format, indentation, and better help to solve your problems.

Robs K
7,146 Points-
*I don't think it is necessary to be redundant *
Why print("We have {number of tickets remaining}") and when the user logs in again you print the same message?
- Your conditional to check for
'Y'
or'N'
doesn't use the confirm variable for validation and there is no option for a user who inputsNo
.
- Your conditional to check for
Try this
ticket_price = 10
tickets_remaining = 100
while(tickets_remaining):
try:
name = input('What\'s your name? ')
print(f"Welcome {name}. There are {tickets_remaining} tickets remaining.") #used python 3.5+ string formating
quantity = int(input('How many tickets do you want? '))
if quantity > tickets_remaining:
print(f"There are only {tickets_remaining} tickets remaining.") # changed this line
else:
total = quantity * ticket_price
print(f"For {quantity} tickets the total price is ${total}")
confirm = input("Would you like to continue. Enter Y/N ")
if confirm == "Y": #if confirm
print("SOLD!")
tickets_remaining -= quantity
if confirm == 'N': # if !confirm
print(f"We are sorry to hear that, {name}! Maybe next time.")
except:
print("Sorry, we are sold out! :(")
break # if we do not have any more tickets, the loop stops

Oscar P
3,690 PointsIn order to keep the original more user friendly message on the code, the way I found to do it was to use 2 except statements and create a Custom Exception that I could raise. I named the exception "ValueUnsupported" at the top of the code. Hope this helps.
class ValueUnsupported(Exception):
pass
TICKET_PRICE = 10
SERVICE_CHARGE = 2
# TODO gather credit card information
def cost_service(tic_rem):
return tic_rem * TICKET_PRICE + SERVICE_CHARGE
tickets_remaining = 100
user_name = input("Welcome, please enter your name: ").title()
while tickets_remaining > 0:
print("There are {} tickets remaining.".format(tickets_remaining))
tickets_requested = input("Hello {}, how many tickets would you like to purchase today? ".format(user_name))
try:
tickets_requested = int(tickets_requested)
if tickets_requested > tickets_remaining:
raise ValueUnsupported("Sorry you are trying or order more tickets than are available")
except ValueError:
print("We ran into an issue, please try again")
except ValueUnsupported as err:
print("({})".format(err))
else:
ticket_total = cost_service(tickets_requested)
print("Your total cost for the tickets will be ${}".format(ticket_total))
confirmation = input("Would you like to purchase the {} tickers for ${}?\nPlease "
"enter yes/no: ".format(tickets_requested, ticket_total))
if confirmation.lower() == "yes":
print("Sold!, the tickets are yours.")
tickets_remaining -= tickets_requested
else:
print("Thank you anyways, {}".format(user_name))
print("Sorry there are not more tickets remaining")
Heidi Fryzell
Front End Web Development Treehouse Moderator 25,178 PointsHeidi Fryzell
Front End Web Development Treehouse Moderator 25,178 PointsThanks for asking this question Shanwa... I noticed the same thing. I guess if we never went back and checked entering a non-number, we never would have known. I tried to use some "if" statements to print two different errors based on if they did not enter an integer or if they entered too many tickets but I could not get it to work. The answer from Boi below makes sense but I would not have known how to do that from what we learned in this course. š¤·š