Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial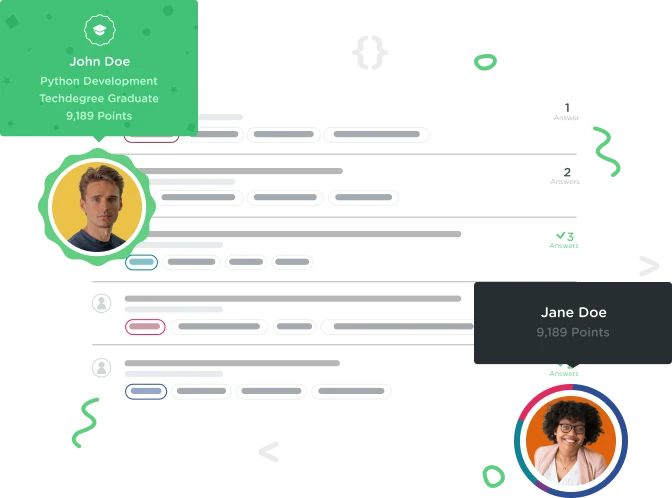

Dominik Huber
4,631 PointsAfter the finished TreehouseDefense Game, am I suppsed to build the game again on my own?
I wouldn't be able to do it. Not in the world. I get the most concepts I learned but "thinking in classes and objects" is the hard part for me.
So what would you prefer:
Trying out the projects he lists under the Teacher's Notes in "Wrap Up", or should I start again with the course and rewatch everything until it get's clear.
I was thinking of building one of my favorite real life games in the console: Yahtzee. Would you say that would be to big of a challenge for me? https://en.wikipedia.org/wiki/Yahtzee
Could anyone lead me into the right direction for this game. I mean which classes would I need for that game?
Will I need a dice class or should I make a GameBoard class where I have an array of 5 dices? Thanks guys!
PS: Great course!
1 Answer
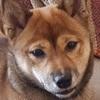
Katie Wood
19,141 PointsI think trying to make Yahtzee would be a great next step. The class model doesn't need to be complicated - you could start by making a Player class with a name and a score array, for example (or just one value for the total, if you want). It could also keep the player's current roll as an array of five generated numbers, with a method called "roll" or something like that, that will randomize numbers representing the dice and set them to that player's current roll. There are other ways to do it, but this is the first one that came to mind for me - hopefully it will be helpful as you're getting started.
The rest of the game could mostly be coded out with some player feedback and conditionals, since there are a set number of rounds in Yahtzee.
Practice is what will allow you to remember and really understand what you've learned in the course. If things are really foggy, there's nothing wrong with going back through, but when you feel comfortable I'd definitely say go for it - you have something you actually want to build, which is awesome.
Dominik Huber
4,631 PointsDominik Huber
4,631 PointsThank for the awesome answer. I think I will actually try this out.
I will now write a few lines in pseudo code how I want that to be and then start making it.
I imagine it like this as an helper "app" for the actual real life game when you have no dices around:
It asks you to choose an option:
1.) Roll dices 2.) Quit the game
After the first roll you have a third option:
3.) Roll choosen dices
Which leads to for example:
5 - 2 - 2 - 1 - 4 "Chose the dices you want to keep and reroll the others: "
The user then types in the numbers in a line like so:
22
Then the game will save the 22 in a new array and adds 514 to a new array which gets then rerolled and ask again.
If the turn is 3 (so you have 3 tries) you then have the final output and the whole program starts again.
Would that be a reasonable idea or is it completely wrong?
Katie Wood
19,141 PointsKatie Wood
19,141 PointsThat sounds cool! You may not need the player class in that case, unless you would still want it to keep track of their names and scores, for example. You could definitely have all of the dice in an array, and then print the values out. You could have the user specify which dice they would like to reroll - I'd recommend having them separate it with commas (like "3, 4, 3"). That would let you write the separate values to an array like:
string[] toReroll = userEntry.Split(',');
That would allow you to split the string they entered into separate numbers before you parse them. You don't have to do it this way, though - just a suggestion to get things going!
What you're talking about definitely sounds reasonable - you could roll, get feedback on which to reroll, and loop that so you can limit how many times they can do it. You could have a "roll" or "hand" class to keep track of the dice and do calculations for you. Give it a shot!
Katie Wood
19,141 PointsKatie Wood
19,141 PointsJust thought of one other thing you might want to think about - if you ask for which they want to reroll, you don't necessarily have to write the ones they want to keep to a new array. You can search the array for each value they entered, and reroll the die and overwrite the number right there. Just a thought - there are many ways to set this up, so don't worry too much about being "wrong" - try to get it to work first, then you can look through and see if you can streamline things. :)
Dominik Huber
4,631 PointsDominik Huber
4,631 PointsThank you once again Katie :) I'm actually on programming it but I have the first problem. I googled it and I think I know what the problem is, but I don't know how to fix it.
I have this code:
When I instantiate a new Dice object it throws an StackOverflowException. I googled it and the problem could be because I set the value with the setter it then will call it again and again? Or is the problem lying elsewhere?
Here I have another class
I then put it all together in main like so:
What is the problem? Can you give me tips what I can do better in my classes so far (in the sense of OOP)? Thx!
Katie Wood
19,141 PointsKatie Wood
19,141 PointsYou're off to a good start - I have a suggestion for the Dice class, and it could possibly fix the error. You may want to try writing the code below the Random something like:
The reason I do it this way is to make sure users can only set the value of the dice by calling the Roll method on them. It also would let you reroll a single die by selecting it in your hand object and calling Roll on it, which might be handy when you have a list of specific ones the user wants to reroll. I tried instantiating this and it seemed to work - hope this helps!
Dominik Huber
4,631 PointsDominik Huber
4,631 PointsHi,
I just finished the first prototype or MVP from my Yahtzee Game. I have pushed it to Github: https://github.com/dhuber666/Yahtzee-Console
Maybe you can take a quick look at it and tell me what you think about it and what I could do better about it.
It doesn't handle every exception atm but most of critical erros like when the user does not put anything in console or not an integer.
The basic idea works. Now I have to fine tune it tomorrow.
Thank you for all your help!
Katie Wood
19,141 PointsKatie Wood
19,141 PointsHello again!
I took a quick look, and you're doing great! It works pretty well, and the console interface is pretty easy to navigate. You can always go through and make names more descriptive (for example, the way it's implemented here, toReroll is actually indicating which to keep, which is a little counter-intuitive - could change it to toKeep or something instead if you wanted to make it more descriptive of what it actually does). I'll leave that up to you though - that part is more about what you think makes the code easiest to read and understand if you were to, say, come back to it six months from now.
The only functional thing I found is this: it looks like the program currently rerolls a die if it is not found in the array of items the user wants to keep. This works just fine if all of the values are different, or if the user wants to keep all dice of the same value. However, what if the user has two 4s, for example, but only wants to keep one? The way it works now, it will keep both, even if the user only wanted to keep one, because typing one '4' puts it in the array of items to keep, which keeps it from being rerolled.
You have some options here - one would be to have the user select which position (1-5) they want to keep, and then reroll by position in the array rather than value (remember that any position they give will need to have 1 subtracted to get the array index, since arrays start at 0). Another option would be to remove an item from the list of numbers to keep once a match is found for it - that would keep it from matching more than once, unless it actually appears more than once in the user's input.
Great job so far - keep at it!
Dominik Huber
4,631 PointsDominik Huber
4,631 PointsYou are awesome!
Thx, didn't figured that I named the method completely wrong. Of course it should be named toKeep instead of toReroll.
You are right I haven't thought about it yet. I will look into it today and fix it and add more functionality to the game. Maybe a little KI where the computer also rolls and scores? :)
Dominik Huber
4,631 PointsDominik Huber
4,631 PointsHi
I proudly present you my first working prototype of Blackjack played in the console :D Here is the link if you want to check it out: https://github.com/dhuber666/BlackjackConsole It was a very nice experience to make this game. I learned a ton of stuff and also get a good idea why OOP is so nice.
I will now go on with the Track and learn something new about the syntax. In the future I will add a bid system to the game and also remake it with a guy. (Want to take the .net WEB course next). Thx again for your help.
Katie Wood
19,141 PointsKatie Wood
19,141 PointsI'm glad I was able to help - that's awesome!