Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial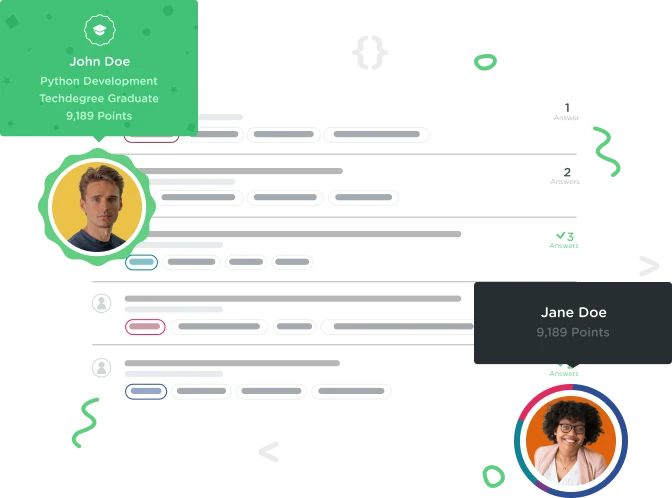

Leslie Lewis
1,088 PointsAgain, it works fine in my console.
I'm getting even more tired of this
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = "red"
self.fuel_remaining = "40"
for key, value in kwargs.items():
setattr(self, key, value)
1 Answer
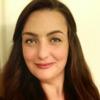
Jennifer Nordell
Treehouse TeacherHi there, Leslie Lewis ! Yes, it partially works in your console, but not quite like we're expecting. The point of an __init__
is to set up the initial values on an instance of an object. However, in your code, your __init__
takes a color
and a fuel_remaining
. We want to set those at the time we create the instance.
Here's your code and the creation of an instance and a print statement of the color
attribute.
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = "red"
self.fuel_remaining = "40"
for key, value in kwargs.items():
setattr(self, key, value)
my_car = RaceCar("blue", 20)
print(my_car.color)
That print statement will print out "red". Every time you make a new RaceCar
you are hard-coding the color to red, even if you tell it you want it to be blue or some other color. Also, the fuel_remaining
is meant to be an integer and not a string.
But if you do this:
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
my_car = RaceCar("blue", 20)
print(my_car.color)
Then the color
attribute of my_car
will be "blue" because you set it through the arguments at the time it was created.
Just remember that just because something works in your console, doesn't necessarily mean that it does exactly what the code challenge is expecting. The lack of a syntax error does not eliminate the possibility that the functionality is somewhat off.
Hope this helps!