Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial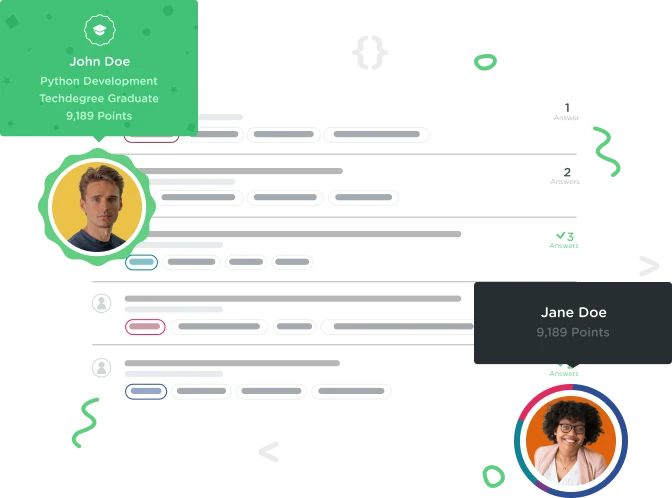

Phillip Hurst
4,204 PointsAiming with an empty shotgun!
I am a couple months new to programming of any kind. In this challenge (as in others) I find myself looking at the TODOs and seeing fragments of ideas swirling around in my head; however, being unable to figure out what exactly to do.
Is this just a lack of experience with Java? How do I proceed as this can be very frustrating: Here is an example of my thought process:
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
// TODO:csd - Print out the results that were gathered here by rendering the template
// try1:pdh -
System.out.print(results);
System.out.print(tmpl.render());
???
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) {
// TODO:csd - Prompt the user for the response to the phrase, make sure the word is censored, loop until you get a good response.
// try1:pdh -
System.out.printf("Please provide the following: %s %n", phrase);
if (phrase.contains(mCensoredWords.get)) {
System.out.printf("%s contains an invalid word. Please try again %n", phrase);
System.out.printf("Please provide valid words for the following: %s %n", phrase);
}
return "???";
}
I realize my lines of code are probably rudimentary and I see that they don't actually allow for input (I don't think)... maybe I need to find a in person class where I can get some coaching in real time until I can form good Java ideas?
6 Answers

Craig Dennis
Treehouse TeacherHmmm. Try not lowercasing the word they entered. You can use that to check, but keep the values in the same case for the results.
Great job sticking with it! This looks great!

Craig Dennis
Treehouse Teachertmpl.render
takes the results
as a parameter.
I don't see you receiving input from the user in the promptForWord
. That is what you should check and return.
mCensoredWords
is a java.util.Set
and it has a contains
method. It expects a parameter of what it should look for, you want to check what the user entered, and loop until it is valid.
Does that help?

Phillip Hurst
4,204 PointsIt does help. been working java coding methods at codingbat.com/java and my confidence is returning.
I am also recovering from Carpal Tunnel Release surgery; so I will probably be rewinding a few of the lessons to get back into this.
Thanks for the feedback.

Phillip Hurst
4,204 Points public String promptForWord(String phrase) throws IOException {
// TODO:csd - Prompt the user for the response to the phrase, make sure the word is censored, loop until you get a good response.
// try1:pdh - The following line outputs to console So I know I am in this section of the code.
System.out.println("Here I check for words");
mReader.readLine();
return "";
Ready for a bit more guidance. I still do not have an actual 'prompt' like:
("Please provide a %s %n", name);
Also there is nothing done with the words I pass in; probably will need to do something with the Return line(?) not sure what yet. I tried the following:
mReader.readLine("What is your name? %n");
I get the following error: Error:(81, 26) java: incompatible types: java.lang.String cannot be converted to boolean
readLine is a boolean? help.

Craig Dennis
Treehouse TeachermReader.readLine()
is reading a line of input from the console and it returns a string. Phrase
is the text between the double underscores in the template. You know the fill in the blank request.
System.out.printf("Enter a %s: ", phrase);
String word = mReader.readLine();
// TODO: Ensure the word is valid
return word;
Hope it helps! Keep me posted!

Phillip Hurst
4,204 PointsYay! Now that I see it written out it makes sense. 'phrase' is already defined so we pass it in the printf, then create a string to contain whatever is input (I mistakenly thought something had to be passed in by the program instead of just waiting for input from the console). The returned 'word' is handed back to the method that called promptForWord().
Now on to the comparing method...

Phillip Hurst
4,204 PointsI have everything working; however I still need to prompt for the user's story template.

Phillip Hurst
4,204 PointsUpdate:
Program runs fine in IntelliJIDEA but when copy/paste into challenge I am getting this error.
Looks like you didn't present the TreeStory(3)
Here is my code:
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = prompter.promptForStory();
Template tmpl = new Template(story);
prompter.getReader();
prompter.run(tmpl);
List<String> prompterResults = prompter.newWords;
String results = tmpl.render(prompterResults);
System.out.printf("Your TreeStory:%n%n%s", results);
}
}
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public List<String> newWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
public BufferedReader getReader() {
return mReader;
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
newWords = results;
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
System.out.printf("Enter a %s: ", phrase);
String word = mReader.readLine().toLowerCase();
while (mCensoredWords.contains(word)) {
System.out.printf("That word is not allowed. Please enter another %s: ", phrase);
String newWord = mReader.readLine().toLowerCase();
word = newWord;
}
return word;
}
public String promptForStory() {
System.out.printf("Enter the story: ");
String story = null;
try {
story = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return story;
}
}
Phillip Hurst
4,204 PointsPhillip Hurst
4,204 PointsOK... challenge complete; however, I don't really understand why.
I removed the .toLowerCase() methods out of promptForWord(){} and it passed the check.
I added the toLowerCase() originally because Dork or dORK were getting accepted into the story.
Thanks for the help along the way! Now forward>>>