Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial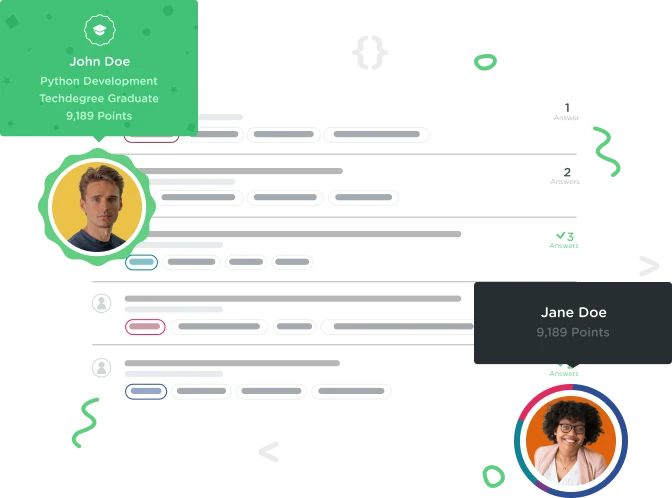
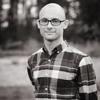
Mark Brady
6,239 PointsAjax and Workspaces
Can I use workspaces to make a Ajax request to an API? I'm new to this an have been stuck here for a while.
Here is my code:
<!doctype html>
<html>
<head>
<title>Random Quotes</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.5/css/bootstrap.min.css" integrity="sha384-AysaV+vQoT3kOAXZkl02PThvDr8HYKPZhNT5h/CXfBThSRXQ6jW5DO2ekP5ViFdi" crossorigin="anonymous">
<link rel="stylesheet" href="css/main.css">
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script src="js/quote.js"></script>
</head>
<body>
<div id="quote-box">
<h4 id="quote">So, you're interested in a random quote, are you not?</h4>
<p id="from-who">Origin of qoute</p>
<div class="clearfix">
<button class="float-l mb-1" id="social">Social</button>
<button class="float-r mb-1" id="new">New Quote</button>
</div>
</div> <!-- quote-box -->
<p id="copyright">By <span class="font-italic"><a target= "_blank"href="https://www.freecodecamp.com/fcc51292858">Mark Brady</a></span></p>
</body>
$(document).ready(function () {
// Ajax
var url = 'http://quotes.stormconsultancy.co.uk/random.json';
$.ajax({
type: 'GET',
url: url,
success: function(data) {
console.log(data);
});
}
}); // ajax
}); // end ready
2 Answers
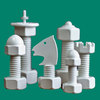
Steven Parker
231,008 PointsYou just have a line with some stray punctuation.
By my count, it's line 10. The one that follows "console.log(data);
", which has these 3 symbols:
});
That entire line is stray punctuation and should be removed.

Ryan S
27,276 PointsHi Mark,
I'm not an expert with this (still learning myself), but I'll share what I can.
You can't make a cross-domain AJAX request to an API. Instead, the workaround is to request a "JSONP" script. (It is possible to do this in workspaces.)
It is very common to use the .getJSON()
method for this purpose. In this case, you will need to let the target API know that you want JSONP. You do this by adding the following to the end of the API URL: ?callback=?
(It may be slightly different depending on the server side API)
But if you use .ajax()
, I believe you will need to specify one more parameter: dataType: "jsonp"
. This should automatically add the "?callback=?" string above.
If you haven't gone through AJAX basics, specifically the last stage where you make a request to the Flickr API, I highly recommend it. It goes through a lot of this in detail.
Hopefully this helps.
Mark Brady
6,239 PointsMark Brady
6,239 PointsWow, yeah you're right. I removed it, but I'm still getting a 404 error. I'm not even sure I'm doing it right or using the api correctly.
Any thoughts?
Steven Parker
231,008 PointsSteven Parker
231,008 PointsAll I can tell you is that as soon as I removed the stray line, it fetched a quote and logged it to the console.