Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial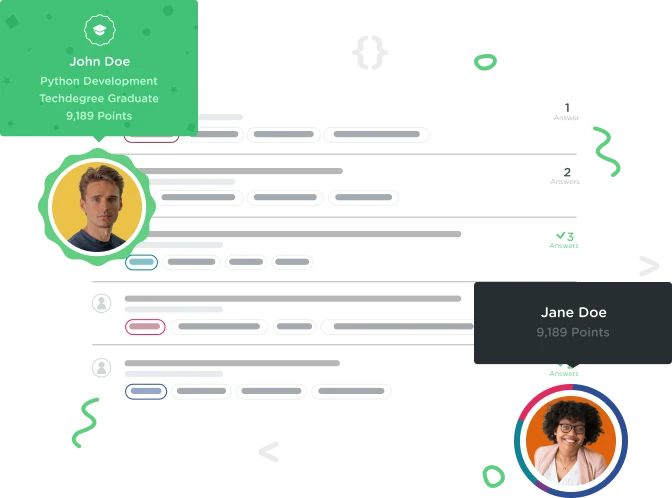
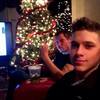
Andrew McCombs
4,309 Pointsajax code issue
Okay in the php you will see that it says echo"signup_success" which is an ajax.responseText, but for some reason its not echoing the ajax. its echoing out the words signup-success. Does anyone know why?
<?php
include 'core/init.php';
include 'includes/overall/header.php';
//Checking if the user is logged in, if so redirecting them.
if (isset($_SESSION['username'])) {
header('Location: message.php?msg=You already have an account');
exit();
}
// Ajax calls this NAME CHECK code to execute
if(isset($_POST["usernamecheck"])){
$username = preg_replace('#[^a-z0-9_]#i', '', $_POST['usernamecheck']);
$sql = "SELECT id FROM users WHERE username='$username' LIMIT 1";
$query = mysqli_query($mysqli, $sql);
$uname_check = mysqli_num_rows($query);
if (strlen($username) < 3 || strlen($username) > 16) {
echo '<strong style="color:#F00;">3 - 16 characters please</strong>';
exit();
}
if (is_numeric($username[0])) {
echo '<strong style="color:#F00;">Usernames must begin with a letter</strong>';
exit();
}
if ($uname_check < 1) {
echo '<strong style="color:#009900;">' . $username . ' is OK</strong>';
exit();
} else {
echo '<strong style="color:#F00;">' . $username . ' is taken</strong>';
exit();
}
}
// Ajax calls this REGISTRATION code to execute
if(isset($_POST["u"])){
// GATHER THE POSTED DATA INTO LOCAL VARIABLES
$u = preg_replace('#[^a-z0-9_]#i', '', $_POST['u']);
$e = mysqli_real_escape_string($mysqli, $_POST['e']);
$f = preg_replace('#[^a-z]#i', '', $_POST['f']);
$l = preg_replace('#[^a-z]#i', '', $_POST['l']);
$p = $_POST['p'];
$g = preg_replace('#[^a-z]#', '', $_POST['g']);
$c = preg_replace('#[^a-z ]#i', '', $_POST['c']);
// GET USER IP ADDRESS
$ip = preg_replace('#[^0-9.]#', '', getenv('REMOTE_ADDR'));
// DUPLICATE DATA CHECKS FOR USERNAME AND EMAIL
$sql = "SELECT id FROM users WHERE username='$u' LIMIT 1";
$query = mysqli_query($mysqli, $sql);
$u_check = mysqli_num_rows($query);
// -------------------------------------------
$sql = "SELECT id FROM users WHERE email='$e' LIMIT 1";
$query = mysqli_query($mysqli, $sql);
$e_check = mysqli_num_rows($query);
// FORM DATA ERROR HANDLING
if($u == "" || $e == "" || $f == "" || $l == "" || $p == "" || $g == "" || $c == ""){
echo "The form submission is missing values.";
exit();
} else if ($u_check > 0){
echo "The username you entered is alreay taken";
exit();
} else if ($e_check > 0){
echo "That email address is already in use in the system";
exit();
} else if (strlen($u) < 3 || strlen($u) > 16) {
echo "Username must be between 3 and 16 characters";
exit();
} else if (is_numeric($u[0])) {
echo 'Username cannot begin with a number';
exit();
} else {
// END FORM DATA ERROR HANDLING
// Begin Insertion of data into the database
// Hash the password and apply your own mysterious unique salt
// $cryptpass = crypt($p);
// $p_hash = randStrGen(20)."$cryptpass".randStrGen(20);
$p_hash = md5($p);
// Add user info into the database table for the main site table
$sql = "INSERT INTO users (username, email, firstname, lastname, password, gender, country, ip, signup, lastlogin, notescheck) VALUES('$u','$e','$f','$l','$p_hash','$g','$c','$ip',now(),now(),now())";
$query = mysqli_query($mysqli, $sql);
$uid = mysqli_insert_id($mysqli);
// Establish their row in the useroptions table
$sql = "INSERT INTO useroptions (id, username, background) VALUES ('$uid','$u','original')";
$query = mysqli_query($mysqli, $sql);
// Create directory(folder) to hold each user's files(pics, MP3s, etc.)
if (!file_exists("user/$u")) {
mkdir("user/$u", 0755);
}
// Email the user their activation link
$to = "$e";
$from = "support@bettergamerzunited.com";
$subject = 'Better Gamerz United Account Activation';
$message = '<!DOCTYPE html><html><head><meta charset="UTF-8"><title>Better Gamerz United Message</title></head><body style="margin:0px; font-family:Tahoma, Geneva, sans-serif;"><div style="padding:10px; background:#333; font-size:24px; color:#CCC;"><a href="http://www.bettergamerzunited.com"><img src="http://www.bettergamerzunited.com/img/BGU Logo.png" width="36" height="30" alt="Better Gamerz United" style="border:none; float:left;"></a>Better Gamerz United Account Activation</div><div style="margin: 0px auto; background-color:white; padding:24px; font-size:17px;">Hello '.$f.',<br /><br>You have received this email because this email address <br />was used during registration for our forums. <br />If you did not register at our forums, please disregard this <br />email. You do not need to unsubscribe or take any further action.<br /><br /><hr>Activation Instructions<hr><br>Thank you for registering. <br />We require that you "validate" your registration to ensure that <br />the email address you entered was correct. This protects against<br />unwanted spam and malicious abuse.<br /><br/>To activate your account, simply click on the following link:<a href="http://www.bettergamerzunited.com/activation.php?id='.$uid.'&u='.$u.'&e='.$e.'&p='.$p_hash.'">Activate your account now</a><br /><br />(Some email client users may need to copy and paste the link into your web <br>browser. Right Click like and copy the address).<br><br> After you activated your account please use the following username and the password you created. <br/><br/> * Username: <b>'.$u.'</b><br> * Password: Did you forget it already?<br /><br><hr>Support Instructions<hr><br>If you still cannot validate your account, it\'s possible that the account has been removed.<br>If this is the case, please contact an administrator to rectify the problem or reply to this email with the<br>subject including your username - Activation Issues/Login Issues. In the body explain the error message<br>and this includes login issue also.<br><br>Thank you for registering and enjoy your stay!<br><br><a href="http://www.bettergamerzunited.com">Better Gamerz United</a></div></body></html>';
$headers = "From: $from\n";
$headers .= "MIME-Version: 1.0\n";
$headers .= "Content-type: text/html; charset=iso-8859-1\n";
mail($to, $subject, $message, $headers);
echo "signup_success";
exit();
}
exit();
}
?>
<script>
function restrict(elem){
var tf = _(elem);
var rx = new RegExp;
if(elem == "email"){
rx = /[' "]/gi;
} else if(elem == "username"){
rx = /[^a-z0-9_]/gi;
}
tf.value = tf.value.replace(rx, "");
}
function emptyElement(x){
_(x).innerHTML = "";
}
function checkusername(){
var u = _("username").value;
if(u != ""){
_("unamestatus").innerHTML = 'checking ...';
var ajax = ajaxObj("POST", "signup.php");
ajax.onreadystatechange = function() {
if(ajaxReturn(ajax) == true) {
_("unamestatus").innerHTML = ajax.responseText;
}
}
ajax.send("usernamecheck="+u);
}
}
function signup(){
var u = _("username").value;
var e = _("email").value;
var f = _("firstname").value;
var l = _("lastname").value;
var p1 = _("pass1").value;
var p2 = _("pass2").value;
var c = _("country").value;
var g = _("gender").value;
var status = _("status");
if(u == "" || e == "" || f == "" || l == "" || p1 == "" || p2 == "" || c == "" || g == "" ){
status.innerHTML = "Fill out all of the form data";
} else if(p1 != p2){
status.innerHTML = "Your password fields do not match";
} else if( _("terms").style.display == "none"){
status.innerHTML = "Please view the terms of use";
} else {
_("signupbtn").style.display = "none";
status.innerHTML = 'please wait ...';
var ajax = ajaxObj("POST", "signup.php");
ajax.onreadystatechange = function() {
if(ajaxReturn(ajax) == true) {
if(ajax.responseText != "signup_success"){
status.innerHTML = ajax.responseText;
_("signupbtn").style.display = "block";
} else {
window.scrollTo(0,0);
_("signupform").innerHTML = "OK "+u+", check your email inbox and junk mail box at <u>"+e+"</u> in a moment to complete the sign up process by activating your account. You will not be able to do anything on the site until you successfully activate your account.";
}
}
}
ajax.send("u="+u+"&e="+e+"&f="+f+"&l="+l+"&p="+p1+"&c="+c+"&g="+g);
}
}
function openTerms(){
_("terms").style.display = "block";
emptyElement("status");
}
/* function addEvents(){
_("elemID").addEventListener("click", func, false);
}
window.onload = addEvents; */
</script>
<div class="container background">
<p class="heading-title">Register</p>
<form name="signupform" id="signupform" onsubmit="return false" class="form-horizontal" role="form">
<div class="form-group">
<label for="username" class="col-sm-2 control-label">Username</label>
<div class="col-sm-10">
<input type="text" onblur="checkusername()" onkeyup="restrict=('username')" maxlength="16" name="username" class="form-control" id="username" value="" autocomplete="off" placeholder="Username">
</div>
<div class="info" id="unamestatus"></div>
</div>
<div class="form-group">
<label for="email" class="col-sm-2 control-label">Email</label>
<div class="col-sm-10">
<input type="email" onfocus="emptyElement('status')" onkeyup="restrict=('email')" maxlength="88" name="email" class="form-control" id="email" value="" autocomplete="off" placeholder="Email Address">
</div>
</div>
<div class="form-group">
<label for="firstname" class="col-sm-2 control-label">First Name</label>
<div class="col-sm-10">
<input type="text" onfocus="emptyElement('status')" name="first_name" class="form-control" id="firstname" value="" autocomplete="off" placeholder="First Name">
</div>
</div>
<div class="form-group">
<label for="lastname" class="col-sm-2 control-label">Last Name</label>
<div class="col-sm-10">
<input type="text" onfocus="emptyElement('status')" name="last_name" class="form-control" id="lastname" value="" autocomplete="off" placeholder="Last Name">
</div>
</div>
<div class="form-group">
<label for="pass1" class="col-sm-2 control-label">Password</label>
<div class="col-sm-10">
<input type="password" onfocus="emptyElement('status')" maxlength="16" name="password" class="form-control" id="pass1" autocomplete="off" placeholder="Password">
</div>
</div>
<div class="form-group">
<label for="pass2" class="col-sm-2 control-label">Validate Password</label>
<div class="col-sm-10">
<input type="password" onfocus="emptyElement('status')" maxlength="16" name="password_again" class="form-control" id="pass2" autocomplete="off" placeholder="Validate Password">
</div>
</div>
<div class="form-group">
<label for="gender" class="col-sm-2 control-label">Gender</label>
<div class="col-sm-10">
<select id="gender" onfocus="emptyElement('status')" class="form-control">
<option value="">Gender</option>
<option value="m">Male</option>
<option value="f">Female</option>
</select>
</div>
</div>
<div class="form-group">
<label for="country" class="col-sm-2 control-label">Country</label>
<div class="col-sm-10">
<select id="country" onfocus="emptyElement('status')" class="form-control">
<option value="">Country...</option>
</select>
</div>
</div>
<div class="form-group">
<div class="col-sm-10">
<a href="#" class="btn btn-primary tos" onclick="return false" onmousedown="openTerms()">
View Terms Of Service
</a>
</div>
</div>
<div class="form-group">
<div class="col-sm-10">
<div id="terms" style="display:none;">
<h3 class="tos">Better Gamerz United Terms of Service</h3>
<p class="tos">Terms of Service are still under construction!</p>
<p class="tos">Please be aware that this does not mean that you are a member of Better Gamerz United. If you would like to be come a member please
contuine with the registration and then fill out our application. Other wise you will have limited use of this site. Thank you Team BGU!!</p>
</div>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 ">
<span class='pull-right text-danger' id="status"></span>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 col-sm-10">
<button type="submit" id="signupbtn" onclick="signup()"onclick class="btn btn-primary pull-right register">Register</button>
</div>
</div>
</form>
</div>
<?php include 'includes/overall/footer.php';
1 Answer
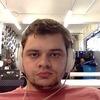
Iago Wandalsen Prates
21,699 PointsWhat you mean by signup.success being an ajax.responseText? you are saying echo "string", that's going to print an string.
Andrew McCombs
4,309 PointsAndrew McCombs
4,309 PointsIn the script code:
Instead of displaying the interHTMl
What do I need to do to send the signup_success to ajax to so that ajax clears the signupform and adds the text above.