Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial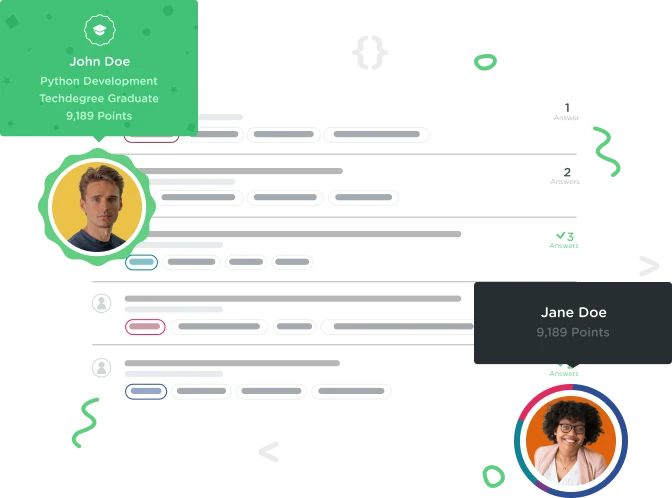

Jonathan Healy
21,601 PointsAJAX Request Not Executing On Button Click?
I can't for the life of me figure out as to why when I simply refresh the page with the xhr.send(); command and refresh, I get the proper AJAX response back in my Chrome console log. However, once inserting the button and creating the sendAJAX function, the button functions correctly however the AJAX response is no longer logged.
<head>
<meta charset="utf-8">
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<title>AJAX with JavaScript Ex1</title>
<script>
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
document.getElementById('ajax').innerHTML = xhr.responseText;
}
};
xhr.open('GET','sidebar.html');
xhr.send();
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<h1>Bring on the AJAX</h1>
<div id="ajax">
</div>
</div>
</div>
</div>
</div>
</body>
And the code with the button included...
<head>
<meta charset="utf-8">
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<title>AJAX with JavaScript Ex2</title>
<script>
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
document.getElementById('ajax').innerHTML = xhr.responseText;
}
};
xhr.open('GET','sidebar.html');
function sendAJAX(){
xhr.send();
document.getElementById('load').style.display = 'none';
}
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Bring on the AJAX</h1>
<button id="load" onclick="sendAJAX()" >Bring it!</button>
</div>
<div id="ajax">
</div>
</div>
</div>
</div>
</div>
</body>
At first I assumed it was something having to do with the onclick handler, however the button disappears so I believe the function is running correctly.
2 Answers

Craig Watson
27,930 PointsHi,
I have uploaded the working version from my workspace for you to take a quick look over.
<script>
var ajaxRequest = new XMLHttpRequest();
ajaxRequest.onreadystatechange = function () {
if ( ajaxRequest.readyState === 4 ) {
document.getElementById( 'ajax' ).innerHTML = ajaxRequest.responseText;
}
};
ajaxRequest.open( 'GET', 'sidebar.html' );
function sendAJAX() {
ajaxRequest.send();
document.getElementById( 'load' ).style.display = "none";
}
</script>
I am noticing a few differences from your code for the version including the button. Hope this helps get you going.
Craig

Aaron Price
5,974 PointsI just copy/pasted your entire code Jonathan Healy and it worked fine on my end. Are you using the treehouse workspace? If you have another environment, be sure to have the sidebar.html accessible in there too.
Jonathan Healy
21,601 PointsJonathan Healy
21,601 PointsCraig, thanks for the response! I am however unable to find any discrepancies with our code other than the variable name for the request. If you could point out where you're seeing the differences I would appreciate it. Also, you mentioned the button but I am positive that I have mine set up in the same way as Dave.
Craig Watson
27,930 PointsCraig Watson
27,930 PointsHi Jonathan,
When you click the button, the button goes but you don't get the response is that correct?
Craig
Jonathan Healy
21,601 PointsJonathan Healy
21,601 PointsCraig,
Yes that is the issue I am experiencing. However, I have that noticed that upon first loading workspaces and then viewing the applicable page in my browser and pressing the button, the AJAX response does in fact log correctly. But when I attempt to recreate the results by reloading the page and pressing the "bring it!" button, the response does not log.