Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial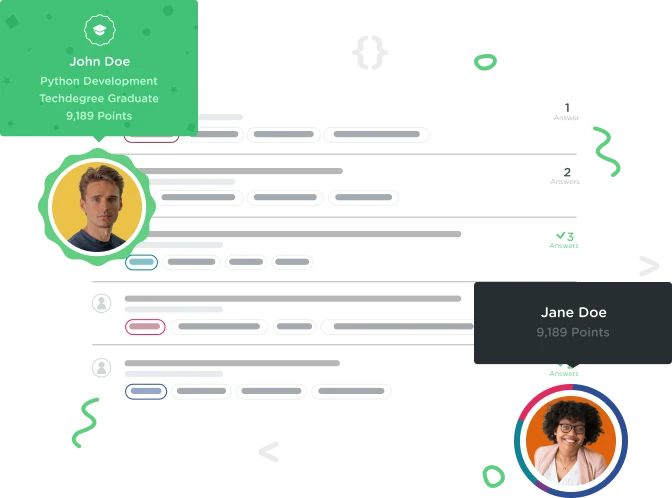
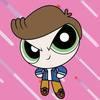
Cezary Burzykowski
18,291 PointsAJAX request not working
Hey there,
to start with, I'm trying to create a simple WEB APP using external API. The WEB APP will have three main functionalities: a) displaying movies b) displaying ratings of movies c) updating movie ratings (if user will change the rating).
For each of these functionalities I have created a separate request (using jQuery). Here is what API documentation provides me with:
a) displaying movies
instruction from API: curl -X GET -H "Content-Type: application/json" -H "Cache-Control: no-cache" 'https://movie-ranking.herokuapp.com/movies'
and here is my AJAX request which works perfectly fine:
var urlMovies = "https://movie-ranking.herokuapp.com/movies";
$.ajax(urlMovies, {
type: "GET",
dataType: "json",
contentType: "application/json",
cache: "no-cache",
success: function(response) {
console.log(response);
},
error: function() {
console.log("request no. 1 have failed");
}
});
b) displaying rating
instruction from API: curl -X GET -H "Content-Type: application/json" -H "Cache-Control: no-cache" 'https://movie-ranking.herokuapp.com/movies/1/ratings'
and here is my AJAX request which doesn't work (shows errors in console): (notice that I have added "?jsoncallback=?" at the end of the URL to enable JSONP)
var urlRatings = "https://movie-ranking.herokuapp.com/movies/1/ratings?jsoncallback=?";
$.ajax(urlRatings, {
type: "GET",
dataType: "json",
data: {
"id": 1
},
contentType: "application/json",
cache: "no-cache",
success: function(response) {
console.log(response);
},
error: function() {
console.log("request no. 2 have failed");
}
})
var urlUpdateRating = "https://movie-ranking.herokuapp.com/movies/1/ratings?jsoncallback=?";
c) updating movie ratings (if user will change the rating).
instruction from API: curl -X POST -H "Content-Type: application/json" -H "Cache-Control: no-cache" -d '{ "rating": 2 }' 'https://movie-ranking.herokuapp.com/movies/1/ratings'
and here is my AJAX request which doesn't work (shows errors in console): (notice that I have added "?jsoncallback=?" at the end of the URL to enable JSONP)
var urlUpdateRating = "https://movie-ranking.herokuapp.com/movies/1/ratings?jsoncallback=?";
$.ajax(urlUpdateRating, {
type: "POST",
dataType: "json",
data: {
"id": 1,
"movie_id": 1,
"rating": 3
},
contentType: "application/json",
cache: "no-cache",
success: function(response) {
console.log(response);
},
error: function() {
console.log("request no. 3 have failed");
}
});
At first I thought that there are some files missing on the server but now I'm thinking that I'm not seeing something (for example security limitations etc.).
Here is a link to my workspace is anyone would like to see live version (almost no HTML and no CSS, at first I want to make sure that all the requests works before coding the whole APP):
Any help would be appreciated!
3 Answers

Seth Kroger
56,413 PointsIf you open up DevTools and look at the Network tab you should see what the immediate issue is. And if you examine the response you got back from the first request you should be able to work out why you're getting the response you do from the 2nd and 3rd.
Also, why are you using JSONP? The server doesn't mention it in the docs and doesn't seem to be set up for it (the query string is ignored and returns pure JSON regardless, as far as I can tell).
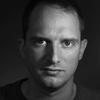
Zoltán Rajcsányi
6,426 PointsHi!
It's an interesting task.
First of all plase share on codepen or jsbin to easy to check others.
Secondly I advice to ignore backend. Just make some js with the result to analyse the js is wrong or the service is not ok (i think).
Good luck! ;)
Zoltan
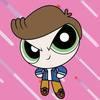
Cezary Burzykowski
18,291 PointsHey Seth!
Thank you for looking into this. I have examined "network" tab and I can see that there are logs showing that both URLs (URL for the first request and URL for second and third request) are fine and they get status "200".
Then on the next logs I can see that second request ("GET") gets status "404". So file that I want to get does not exist. I have examined my first request and I realised that response consists of the three movies (with ID 11, 12, 13). So I made a mistake in my second request - I have passed ID 1 in the body of the request, while such movie doesn't exist. I thought that will solve the problem but that's not it ....
I have used JSONP because I thought that I should. I saw error in the console "No 'Access-Control-Allow-Origin' header is present on the requested resource." and I thought that JSONP would be a way to go (I'm a begginer when it comes to AJAX).
Seth could you please tell me if my train of thought is correct or am I not looking at something correctly?