Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial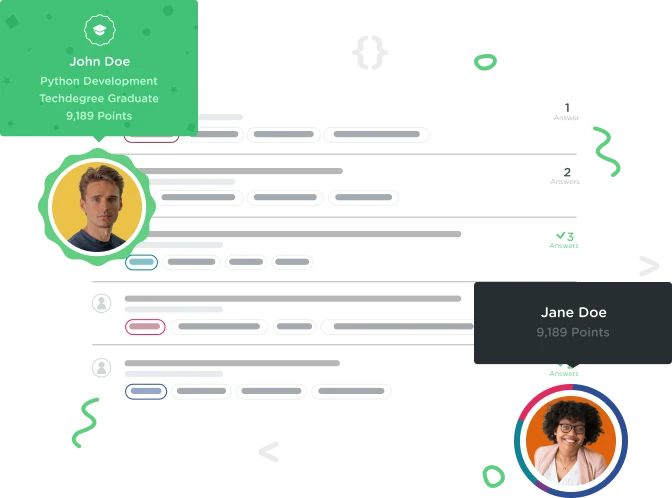
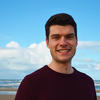
Marco Kühbauch
10,099 PointsAjax Stage 4 Challenge My Solution
I recreated the flickr photo feeder made in this course in pure JavaScript (except the ajax request). I also combined the three buttons and the search form. Any suggestions on how to improve? You can see a working example on CodePen: http://codepen.io/Mkuehb/pen/PNxjpO
// Select all buttons
var btn = document.getElementsByTagName('button');
// Select the form submit
var formSub = document.getElementById('submit');
// Declare animal variable globally, the value will be declared either on clicking a button or using the search form
var animal;
// Declare inputText variable globally
var inputText;
// Loop over the buttons and attach an EventListener to every single one
for (var i = 0; i < btn.length; i++) {
btn[i].addEventListener('click', classChange, false);
}
// When a button is clicked, the classChange function is called
function classChange() {
// Loop over all buttons and remove the selected class
for (var i= 0; i < btn.length; i++) {
btn[i].classList.remove('selected');
}
// Add the selected class to the clicked button to highlight it
this.classList.add('selected');
// Save the buttons text in the animal variable
animal = this.textContent;
// Call the function to make the AJAX request and display the photos
makeAjaxRequest();
}
// When the submit button is clicked
formSub.addEventListener('click', function(e) {
// Stop the default event
e.preventDefault();
// Select the input
inputText = document.getElementById('search');
// Select the inputs text value
var inputTextVal = inputText.value;
// Save the inputs text in the animal variable
animal = inputTextVal;
// Loop over all buttons and remove the selected class
for (var i= 0; i < btn.length; i++) {
btn[i].classList.remove('selected');
}
// Disable the search input while searching
inputText.setAttribute('disabled', true);
// Change the submit buttons text while searching
formSub.value = 'Searching...';
// Disable the submit button while searching
formSub.setAttribute('disabled', true);
// Call the function to make the AJAX request and display the photos
makeAjaxRequest();
});
function makeAjaxRequest() {
// flickr URL
var flickerAPI = 'https://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?';
// flickr options
var flickrOptions = {
tags: animal,
format: 'json'
};
// function to display the photos
function displayPhotos(data) {
// create an unordered list
var photoHTML = '<ul>';
// loop over the retrieved items and create a list item with a link & image
for (var i = 0, photo, n = data.items.length; i < n; i++) {
photo = data.items[i];
photoHTML += '<li>';
photoHTML += '<a href=' + photo.link + '" class="image">';
photoHTML += '<img src="' + photo.media.m + '"></a></li>';
}
photoHTML += '</ul>';
// add the photos to the div with the id of photos
var photos = document.getElementById('photos');
photos.innerHTML = photoHTML;
if (formSub.hasAttribute('disabled')) {
// Re-enable the search input while searching
inputText.removeAttribute('disabled');
// Change back the submit buttons text while searching
formSub.value = 'Search';
// Re-enable the submit button while searching
formSub.removeAttribute('disabled');
}
}
// Make the AJAX request with jQuery's getJSON
$.getJSON(flickerAPI, flickrOptions, displayPhotos);
}