Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial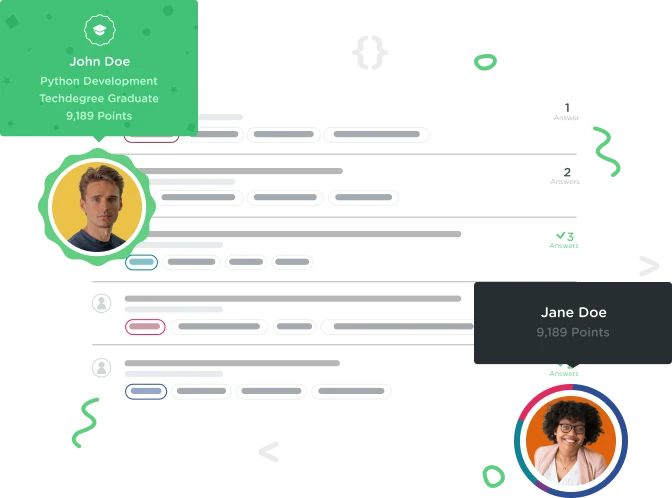
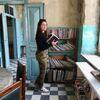
Esther AlQaisi
2,311 PointsAlert box not appearing, even after I removed the value attribute from the input element. Help!
Hi! I removed the value attribute from the input element, expecting the program to display an alert box when I ran it. However, no alert box is appearing. Can someone spot my error? Here's the code:
function isFieldEmpty() { const field = document.querySelector('#info'); if (field.value === ' ') { return true; } else { return false; }
}
const fieldTest = isFieldEmpty();
if (fieldTest === true){ alert("Please provide your information!"); }
2 Answers
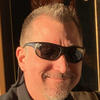
Peter Vann
36,429 PointsHi Ester!
I tested your code and the issue seems to be that you are testing field.value against a one-space string and not an empty one.
You have this:
if (field.value === ' ') // One space
When what you want is this:
if (field.value === '') // No space (empty string)
You could also do this (reacting to user interaction):
const field = document.querySelector('#info');
field.addEventListener('input', (e) => {
if (e.target.value === '') {
setTimeout( () => {
alert("Please provide your information!");
}, 50);
}
});
Note: I had to add the brief setTimeout or the alert will pop up before the input is fully empty.
You can test it here:
https://www.w3schools.com/js/tryit.asp?filename=tryjs_array
Using this:
<!DOCTYPE html>
<html>
<body>
<h2>Empty Input Alert</h2>
<input id="info" type="text" value="Boo!" />
<script>
const field = document.querySelector('#info');
field.addEventListener('input', (e) => {
if (e.target.value === '') {
setTimeout( () => {
alert("Please provide your information!");
}, 50);
}
});
</script>
</body>
</html>
Just paste the HTML code above in the w3schools left pane, run it, and try to delete the "Boo!" text.
I hope that helps.
Stay safe and happy coding!
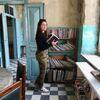
Esther AlQaisi
2,311 PointsThat makes so much sense! I thought I was testing the field value against an empty string, but obviously if I hit the space bar then it's not empty! I'm learning a lot through my errors, thank you for your time!