Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial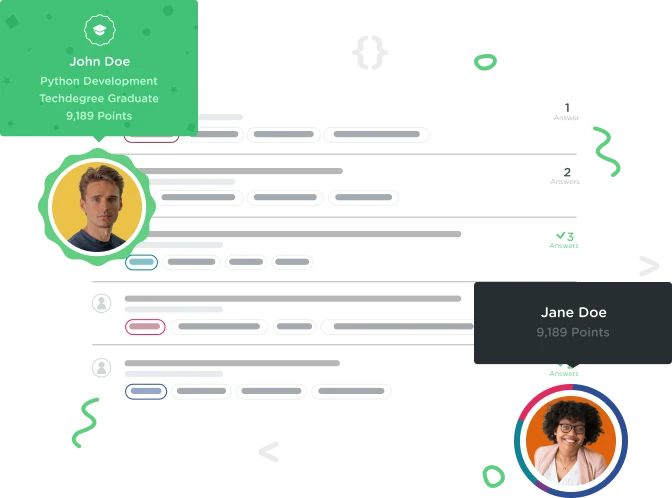

Leno Marin
Courses Plus Student 1,323 PointsAll items in an array to uppercase?
What if I wanted to make all the items in array to uppercase letters. This doesn't seem to do the trick:
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato', 'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber']; var search;
function print(message) { document.write( '<p>' + message + '</p>'); }
while(true) {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit"); inStock = inStock.toUpperCase(); search = search.toUpperCase();
if (search ==='QUIT') { break; } else if ( search === 'LIST') { print( inStock.join(', ') ); } else { if(inStock.indexOf(search) > -1) { print( 'Yes, we have ' + search + ' in the store.'); } else { print (search + ' is not in stock.'); } } }
3 Answers

Recio Jose Luis
4,495 Points.toUpperCase is a string method, if you use it with a array it doesn't run but are you sure that you need the array inStock in uppercase? If you look your code doesn't use it
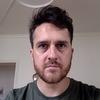
Raphaël Seguin
Full Stack JavaScript Techdegree Graduate 29,228 PointsHi, I think you need to loop through the elements of the array.
for (let i = 0; i < stock.length; i++) {
stock[i] = stock[i].toUpperCase();
}
If you need to make sure all the elements are strings, add an if statement with the condition typeof stock[i] === 'string'
for (let i = 0; i < stock.length; i++) {
if (typeof stock[i] === 'string') {
stock[i] = stock[i].toUpperCase();
}
}
Does it fit your needs ?
Raphaël

Lars Reimann
11,816 PointsAnother useful array method is map. It basically loops through all elements of an array, applies a function to them and pushes the results into a new array:
function toUpper(item) {
return item.toUpperCase();
}
var stockUppercase = inStock.map(toUpper);
Note that inside of map, we are not calling toUpper - there is no () after it. The map function will call toUpper itself on every element in the array.
If you want to get extra fancy, you can inline the function definition of toUpper:
var stockUppercase = inStock.map(function toUpper(item) {
return item.toUpperCase();
});
Naming the function is optional:
var stockUppercase = inStock.map(function (item) {
return item.toUpperCase();
});
Since version 6 of JavaScript, there is also a shorthand notation for defining functions:
var stockUppercase = inStock.map(item=> item.toUpperCase());
Don't get confused with this, it's is perfectly fine to type a simple for-loop for this kind of operation, as shown by Raphaël.