Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial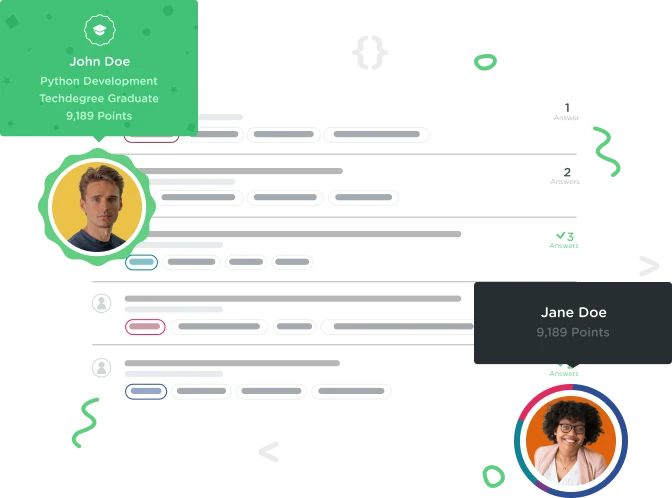
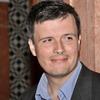
Brian Patterson
19,588 PointsAll my error messages say "undefined" in the console.
Now all my error messages say "undefined". Why is this happening?
const http = require('http');
//Print Error Messages
function printError(error) {
console.error(error.messsage);
}
//const season = 1954;
function printMessage(races, season) {
const message = `There are ${season} grandprix at the ${races} season.`;
console.log(message);
}
//printMessage(22,2017);
//Connecting to the API url (http://ergast.com/api/f1/2012.json)
function getSeason(season) {
try {
const request = http.get(
`http://ergast.com/api/f1/${season}.json`,
response => {
if (response.statusCode === 200) {
//console.dir(response.statusCode);
let body = '';
//Read the data
response.on('data', data => {
//Changing the buffer into a string.
body += data;
});
response.on('end', () => {
try {
//Parse the data
const seasonF1 = JSON.parse(body);
// console.log(body);
// console.log(typeof body);
//console.dir(seasonF1);
printMessage(season, seasonF1.MRData.RaceTable.Races.length);
//Print the data
} catch (error) {
printError(error);
}
});
} else {
const message = `There was an error getting the season for ${season} (${
http.STATUS_CODES[response.statusCode]
})`;
const statusCodeError = new Error(message);
printError(statusCodeError);
}
}
);
request.on('error', printError);
} catch (error) {
printError(error);
}
}
//accept season as a command line argument
//const season = process.argv[2];
//get(season);
// get(1978);
// get(2001);
//console.log(process.argv);
// const seasons = [1978, 1984, 1999, 1950, 1954, 2002];
// seasons.forEach(getSeason);
const seasons = process.argv.slice(2);
seasons.forEach(getSeason);
I really don't understand why this is happening. I have pretty much followed the structure of the code and added a different API.
2 Answers
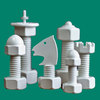
Steven Parker
231,268 PointsYou still have the same error that was pointed out in your previous question, where you have "messsage" (with 3 "s") instead of "message":
//Print Error Messages
function printError(error) {
console.error(error.messsage); // note: too many "s"
}

Matt Coale
Full Stack JavaScript Techdegree Graduate 17,884 PointsThis helped me and I forgot the "m" in my message. Lol! Thanks.
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI ran your code and uncommented the lines to call the functions, and it didn't throw any errors. How can I replicate the problem where it throws an error with no error message?