Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial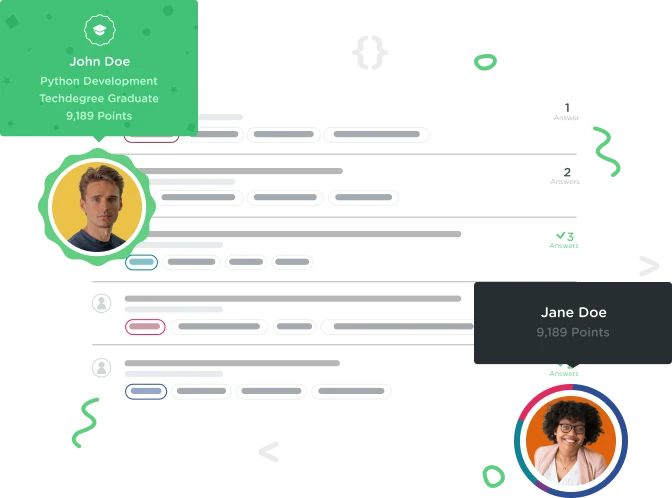
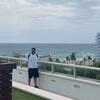
Daniel Corio-Rosas
6,995 PointsAll of my promises returned pending, why?
const astrosUrl = 'http://api.open-notify.org/astros.json'; const wikiUrl = 'https://en.wikipefdia.org/api/rest_v1/page/summary/'; const peopleList = document.getElementById('people'); const btn = document.querySelector('button');
function getJSON(url) { return new Promise((resolve, reject) => { const xhr = new XMLHttpRequest(); xhr.open('GET', url); xhr.onload = () => { if (xhr.status === 200) { let data = JSON.parse(xhr.responseText); resolve(data); } else { reject(Error(xhr.statusText)); } }; xhr.onerror = () => reject(Error('A network error occurred')); xhr.send(); });
}
function getProfiles(json) { const profiles = json.people.map(person => { return getJSON(wikiUrl + person.name); }); return profiles; }
// Generate the markup for each profile
function generateHTML(data) {
const section = document.createElement('section');
peopleList.appendChild(section);
// Check if request returns a 'standard' page from Wiki
if (data.type === 'standard') {
section.innerHTML =
<img src=${data.thumbnail.source}>
<h2>${data.title}</h2>
<p>${data.description}</p>
<p>${data.extract}</p>
;
} else {
section.innerHTML =
<img src="img/profile.jpg" alt="ocean clouds seen from space">
<h2>${data.title}</h2>
<p>Results unavailable for ${data.title}</p>
${data.extract_html}
;
}
}
btn.addEventListener('click', (event) => { getJSON(astrosUrl) .then(getProfiles) .then(data => console.log(data)) .catch(err => console.log(err))
event.target.remove();
});
2 Answers
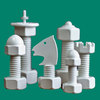
Steven Parker
229,757 PointsSince promises are asynchronous by nature, it's normal for them to be in the pending state when first created. They will keep working alongside your program, and when they resolve (or are rejected) the status will change and your callback will be invoked.
It doesn't look like you needed to post code for this question, but for future questions you may want to take a look at these videos about using Markdown formatting to preserve the code's appearance, and this one about sharing a snapshot of your entire workspace.
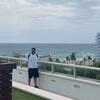
Daniel Corio-Rosas
6,995 PointsWoooow, thats exactly it! Thanks parker
Daniel Corio-Rosas
6,995 PointsDaniel Corio-Rosas
6,995 Pointshttps://w.trhou.se/c7vt8z1ebp
Steven Parker
229,757 PointsSteven Parker
229,757 PointsI don't see any issues with your workspace, but it's possible that your browser settings are blocking some of the data being requested.
Newer browsers, specifically those created after this course was released, generally don't allow mixed content (secured via https and insecure via http) by default. If you change your browser settings to allow insecure content (instead of blocking it), the requests will be accepted and processed.
Steven Parker
229,757 PointsSteven Parker
229,757 PointsDaniel Corio-Rosas — Glad to help. You can mark a question solved by choosing a "best answer".
And happy coding!