Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial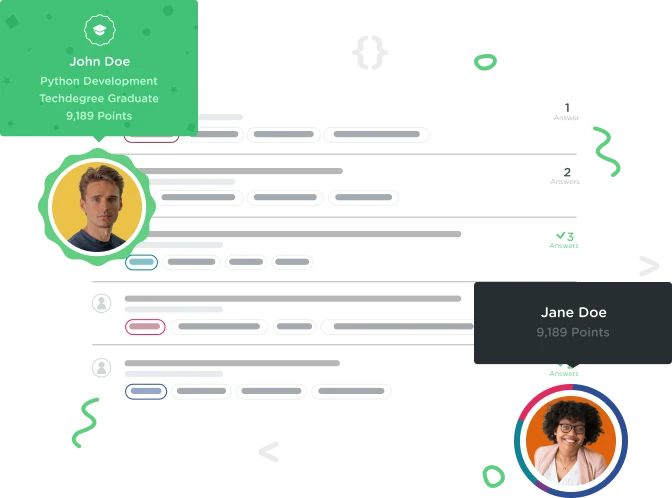

liamthornback2
9,618 PointsAll player elements still update when I change one score
Here is my code (note: I'm not passing the player index down to the counter component because I wrote my changeScore method differently):
import React, { PureComponent } from 'react';
import Counter from './Counter';
class Player extends PureComponent {
render() {
console.log(this.props.name + " Rendered");
return (
<div className="player">
<span className="player-name">
<button className="remove-player" onClick={() => this.props.removePlayer(this.props.id)}>✖</button>
{ this.props.name }
</span>
<Counter
score={this.props.score}
changeScore={this.props.changeScore}
/>
</div>
);
}
}
export default Player;
And here is the changeScore function:
handleChangeScore = (index) => {
//returning a function that is called by the Counter component
return (change) => {
//updating the score
this.setState(prevState => ({ score: prevState.players[index].score += change }));
};
}
And how I pass it to the player component:
{this.state.players.map((player, index) =>
<Player
name={player.name}
score={player.score}
id={player.id}
key={player.id.toString()}
changeScore={this.handleChangeScore(index)}
removePlayer={this.handleRemovePlayer}
/>
)}
If anyone has any idea why this might not be working correctly for me that would be great.
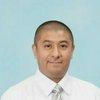
Omar Gonzalez
Full Stack JavaScript Techdegree Graduate 18,444 PointsHello,
The reason there are no changes is that you are missing index={this.props.index}
between score and changeScore
<Counter
score={this.props.score}
changeScore={this.props.changeScore}
/>
I hope that helps
Anton Escalante
1,558 PointsAnton Escalante
1,558 PointsWere you able to figure this one?
Happening to me as well.