Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial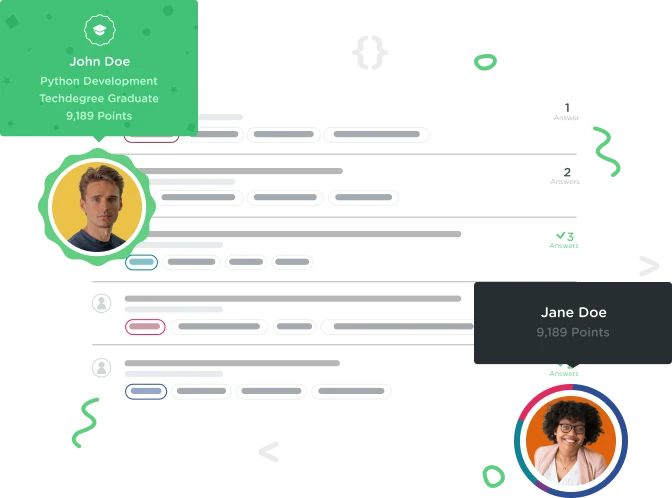
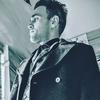
Adam Marasli-Zaade
2,966 PointsAll seem to work fine but whenever I am typing something irrelevant to an int, I get an error.
import random
def game():
#generate a random number between 1 and 10
secret_num = random.randint(1, 10)
guesses=[]
while len(guesses) < 5:
try:
#get a number guess from the player
guess = int(input("Guess a number betwwen 1 and 10: "))
except ValueError:
print("{} isn't a number 'mon.".format(guess))
else:
#compare guess to secret number
if guess == secret_num:
print("You got it 'mon! My number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {} 'mon.".format(guess))
else:
print("My number is lowen than {} 'mon.".format(guess))
guesses.append(guess)
else:
print("You didn't get it 'mon. My number was {}.".format(secret_num))
play_again = input("Do ya want to play again 'mon? Y/n ")
if play_again.lower() != 'n':
game()
else:
print("Bye 'mon!")
game()
The Error:
Traceback (most recent call last):
File "number_game.py", line 12, in game
guess = int(input("Guess a number betwwen 1 and 10: "))
ValueError: invalid literal for int() with base 10: 'k'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "number_game.py", line 34, in <module>
game()
File "number_game.py", line 14, in game
print("{} isn't a number 'mon.".format(guess))
UnboundLocalError: local variable 'guess' referenced before assignment
what do you think? :) thnx
ps. In memory of Vol'jin. :P
2 Answers

Ryan S
27,276 PointsHi Adam,
Your error is the result of trying to convert the input to an int in the same line (line 12).
Say you start the program and enter a non-numeric string as your first guess. It will go to the except:
block as you'd expect, but the problem is that in the string format line, you are trying to use the variable "guess" which hasn't actually been defined yet because it threw a ValueError. So then it throws the error that you are receiving.
You can test this another way by guessing a number first, then guessing a non-numeric string on your second try. You'll notice that it won't give you that error, but "guess" will be defined as the last variable that got successfully assigned to it (i.e., the last number you guessed.)
So in order to fix this, you'd need to first assign "guess" to the input prompt (you can do this outside the try block). Then in your try block you'd try to convert it to an int:
while len(guesses) < 5:
#get a number guess from the player
guess = input("Guess a number betwwen 1 and 10: ")
try:
guess = int(guess)
except ValueError:
print("{} isn't a number 'mon.".format(guess))

Nilton S Bossolan
Courses Plus Student 1,047 PointsWorkaround for this would be to exclude guess input value when printing exception message.
But no idea how to make game print non int in exception.
fahad lashari
7,693 Pointsfahad lashari
7,693 PointsThanks so much dude!
Nahum Smith
3,699 PointsNahum Smith
3,699 PointsHi Ryan,
I tried refactoring using your above method and I still seem to be getting the same error: I even eliminated the reference to the guess variable in the exception string, but that ALSO did not work.
Here is my error: Traceback (most recent call last): File "number_game.py", line 50, in <module> guess_a_num_game() File "number_game.py", line 20, in guess_a_num_game guess = input("Guess a number between 1 and 10: ") File "<string>", line 1, in <module> NameError: name 'ba' is not defined
below is the code (obviously):