Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial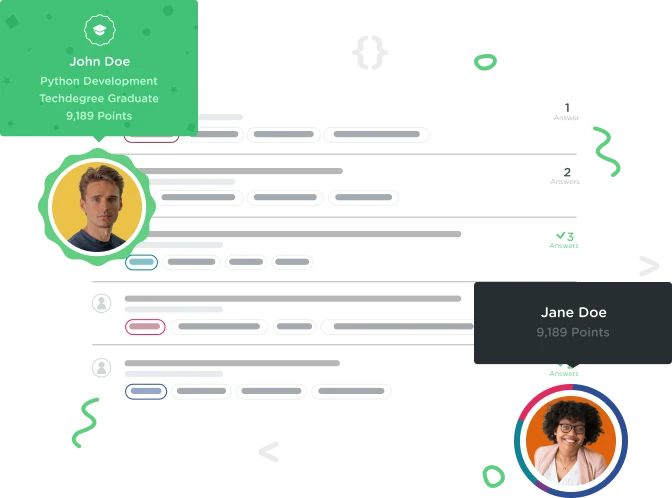

Erik Gooding
5,665 PointsAlright, I'm stumped on the Challenge.
Can someone tell me what is wrong with this? Seems perfectly fine to me.
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
boolean isFullyCharged = false;
if (!isFullyCharged()) {
mBarsCount++;
} while (mBarsCount != MAX_ENERGY_BARS);
return mBarsCount == MAX_ENERGY_BARS;
}
}
9 Answers

boog690
8,987 PointsisFullyCharged() should return a a boolean indicating whether mBarsCount == MAX_ENERGY_BARS.
Lee Reynolds Jr., in your charge() method, I think your while loop should look like:
while (! this.isFullyCharged()) {
...
}
Alternatively, your while loop can be:
while (mBarsCount != MAX_ENERGY_BARS) {
...
}
Both will get you the inequality test you're looking for.

Erik Gooding
5,665 PointsThanks a lot! I guess I didn't understand the instructions too well either.
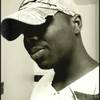
Lee Reynolds Jr.
5,160 PointsThank yo so much! That did the trick!

boog690
8,987 PointsThe while loop in charge() needs a closing bracket.
mBarsCount++ needs a semi-colon to close the statement.
There needs to be one less closing curly brace at the bottom.

Jacob Martin
4,182 PointsThanks
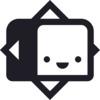
Jake Fleming
Treehouse Guest TeacherSo I know this is already solved, but it seems like you guys might be over complicating the solution. Here's how I solved it:
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}

Kingsley Ubani
22,631 PointsThis is the way I solved it as well. At first I thought I'd follow the model in the lesson but then I remembered: keep it simple.
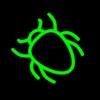
Matthew Stanciu
4,222 PointsThanks, I realized that right before you replied.

boog690
8,987 PointsGreat. Glad you got it on your own. :)

Heather Crummer
13,558 PointsBecause the challenge specifically asked us to use the isFullyCharged method, a while loop, and !, I changed charge to this:
public void charge() { while (!isFullyCharged()) { mBarsCount++; } }
That's all I did and it worked.
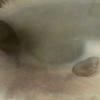
Juan Rodríguez
782 PointsThis worked... I was stucked a while too, instructions aren't clear at all.
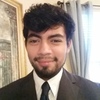
Giancarlo M.
1,502 PointsBecause I failed so miserably the first time I reviewed the lesson after a few days of rest. Now I am confused again and forgot how I solved it the first time. I just can't seem to understand How the while loop. I can get as far as Erik G. did and not have any "error" messages. But I just don't know what I am missing. I know what Boog said, but I don't understand what he means by his comment. Then I was just even more confused by his answer to Lee. Ug, I feel silly admitting all this. However I don't want to continue with my lessons as long as I am this boggled.
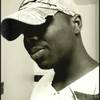
Lee Reynolds Jr.
5,160 PointsWhat is confusing you specifically? I Would like to try and assist with the clarification if I can.
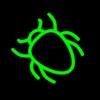
Matthew Stanciu
4,222 PointsCan you copy/paste the code you have?
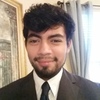
Giancarlo M.
1,502 PointsApplying the "while loop" is really confusing me, it makes me doubt everything.
public class GoKart { public static final int MAX_ENERGY_BARS = 8; private String mColor; private int mBarsCount;
public GoKart(String color) { mColor = color; mBarsCount = 0; }
public String getColor() { return mColor; }
public void charge() { mBarsCount = MAX_ENERGY_BARS; //or does the while loop go here? mBarsCount++; }
public boolean isBatteryEmpty() { return mBarsCount == 0; }
public boolean isFullyCharged() { boolean isFullyCharged = false; if (!isBatteryEmpty()) { mBarsCount++; isFullyCharged = true; } return mBarsCount == MAX_ENERGY_BARS; //Does the while loop go here? while (isFullyCharged != MAX_ENERGY_BARS) { System.out.println("Charging"); if (mBarsCount = isFullyCharged) System.out.println("is fully charged"); } } }
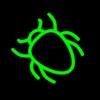
Matthew Stanciu
4,222 PointsOk, so your code is something like this:
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color; mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS; //or does the while loop go here? mBarsCount++;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
boolean isFullyCharged = false;
if (!isBatteryEmpty()) {
mBarsCount++;
isFullyCharged = true;
}
}
return mBarsCount == MAX_ENERGY_BARS; //Does the while loop go here?
while (isFullyCharged != MAX_ENERGY_BARS) {
System.out.println("Charging");
if (mBarsCount = isFullyCharged) {
System.out.println("is fully charged");
}
}
}
I wrapped your code into a code block. This may have been just because you didn't put it in a code block, but I had to add in some extra brackets. You may want to check for that first.
From what I see (but I may see wrong), I think you may have mixed up your isFullyCharged() and charge() methods. Your while loop should go in charge(). isFullyCharged() should only be used for returning mBarsCount == MAX_ENERGY_BARS since it's a boolean.
If you need any more help, let me know.
EDIT: One thing that got me is that it asked me to call isFullyCharged() inside my while loop. So the while loop could be
while (mBarsCount != MAX_ENERGY_BARS) {
mBarsCount++;
}
but when I did that it asked me to call isFullyCharged(), so my while loop ended up being
while (!this.isFullyCharged()) {
mBarsCount++;
}
just like in the original answer by boog690.
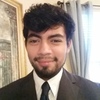
Giancarlo M.
1,502 PointsThank you for all your quick responses I really appreciate it. I think I understand...but I'm still not getting it right. This is what I have now. Also I don't know how to place it in a code block.
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color; mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while (!this.isFullyCharged()) {
mBarsCount++;}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
boolean isFullyCharged = false;
if (!isFullyCharged()) {
mBarsCount++;
isFullyCharged = true;}
return mBarsCount == MAX_ENERGY_BARS;
}
}
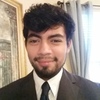
Giancarlo M.
1,502 PointsHAHAH! AWESOME! I JUST LEARNED HOW!!! ^_^
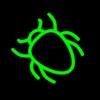
Matthew Stanciu
4,222 PointsHa. Great! Code blocks help.
Delete the if statement in your isFullyCharged() method. That section is already covered in your while loop.
EDIT: Also instead of setting isFullyCharged to false, set it instead to mBarsCount == MAX_ENERGY_BARS. Then it should work.
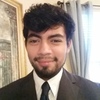
Giancarlo M.
1,502 PointsOH! Is it written in this way to simplify the code? Was I overcomplicating this?
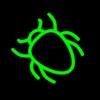
Matthew Stanciu
4,222 PointsI think so. Try what I suggested, see if it works.
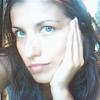
Alina Sirbeanu
992 PointsI dint get it either :(..What mean "this" in the while loop and how and why implements to the program.
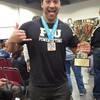
Gabriel Llanes
2,894 Pointsthank god finally an answer, I agree these instructions could be clearer
Lee Reynolds Jr.
5,160 PointsLee Reynolds Jr.
5,160 PointsThis is what I've come up with and it still didn't work. Can someone please assist in the finding of the answer. I don't think that I completely understand the instructions. I've tried adding the while loop in as well but I constantly get an error message about using the wrong operand in my code.
public class GoKart { public static final int MAX_ENERGY_BARS = 8; private String mColor; private int mBarsCount;
public GoKart(String color) { mColor = color; mBarsCount = 0; }
public String getColor() { return mColor; }
public void charge() {
while (!mBarsCount = isFullyCharged()){ mBarsCount++; } }
public boolean isBatteryEmpty() { return mBarsCount == 0; }
public boolean isFullyCharged() { return mBarsCount == MAX_ENERGY_BARS; }
}