Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial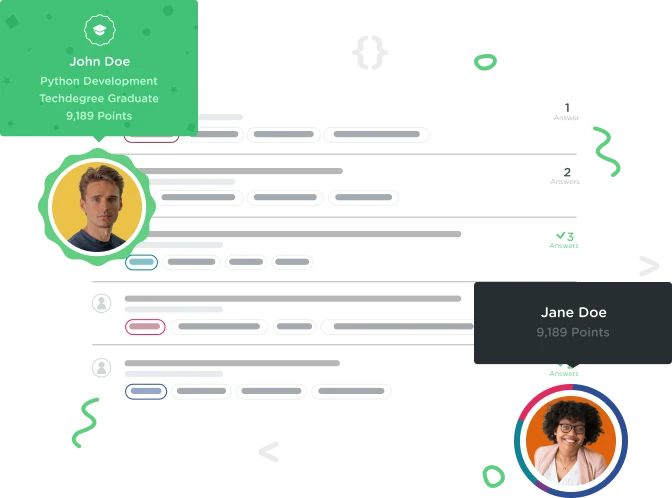
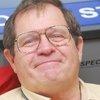
Joe Mackey
20,418 PointsAlright! Now I need you to add a new property called doubles. It should return True if both of the dice have the same va
Adding new property "Doubles" Wanting to return True if doubles are rolled. Am I getting close or have I veered off the track again?
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size = 2, die_class = D6)
@property # I think my mistake is in here, just not sure what I am doing wrong
def doubles(self):
for num in self._sets.values():
if num > 1:
return True
return False # if the loop does not return True allows method to exit gracefully
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
3 Answers
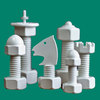
Steven Parker
231,269 PointsIt looks like you just have some indentation issues. The "def" should line up with "@property" above it, and the final "return" needs to line up with the "def".

Brian Smith
4,957 Pointsfrom dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self, *args, **kwargs):
super().__init__(size=2, die_class=D6, *args, **kwargs)
@property
def doubles(self):
return self[0] == self[1]
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}

David Capella
4,126 PointsYou already set self
as two D6, so you only have to compare yourself twice, [0]
and [1]
. Such as:
def doubles(self):
return self[0] == self[1]
If they match it will equal True
and return it. If they do not match it will equal False
and return that.
Taig Mac Carthy
8,139 PointsTaig Mac Carthy
8,139 PointsLegend