Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial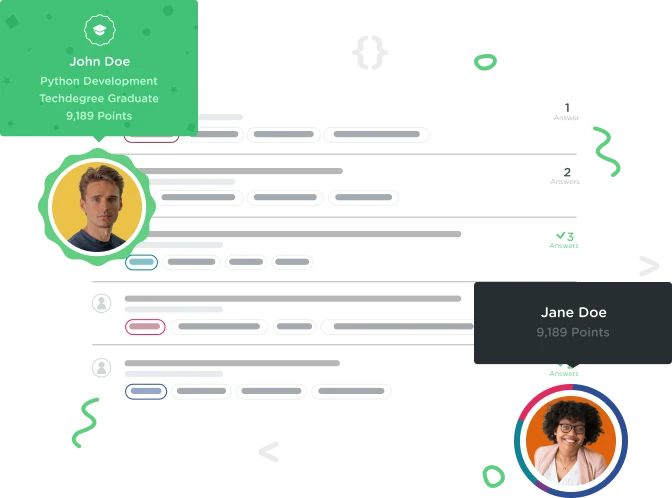
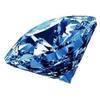
jalil damian
1,452 PointsAlright, this one can be tricky but I'm sure you can do it. Create a function named combo that takes two ordered
。
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(a, b):
15 Answers
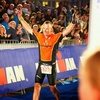
Steve Hunter
57,712 PointsHi Jalil,
I started by passing in two iterable parameters into the method.
Inside the method, I initialised a blank list, I called it output
but that's open for renaming!
Next, because I wanted to access the iterables using their index I set up a for loop with a true counter variable that's a number.
To do this you need to make the for loop run on numbers. Because the iterables are both of the same length we can use that to our advantage. Loop through from zero to the length of the iterable and the loop variable (let's call it i
) will count.
That could look like:
for i in range(0, len(iter1)):
The variable iter1 is one of the parameters passed in to the method like this:
def combo(iter1, iter2):
So iter1
and iter2
are the same length and can contain the example contents suggested in the challenge, or anything else we choose.
Using the for
loop in this way loops through a range object which is a range of numbers between the two parameters passed in. The first parameter is zero (the starting index number of the iterable) and the second is the length of iter1
. This will generate a sequence of counter values allowing you to use square brackets to access each element of both, indeed any, iterables.
At the each loop, access iter1[i]
and iter2[i]
to access the relevant parts of each parameter. turn them into a tuple and add that to the blank list you started the method with. I called it output
, remember? To make the tuple, surround the above two iterable accessors with parentheses and separate them with a comma. Also, remember that items in a list are also separated by commas, and that's what we're returning; a list. So, include a comma after the closing parenthesis. Just add that to the output
list with +=
.
Once the loop has finished, return output
.
That all looks like this - which is my solution and by no means the only way to do this:
def combo(iter1, iter2):
output = []
for i in range(0, len(iter1)):
output += (iter1[i], iter2[i]),
return output
I hope that works for you and you understand my ramblings!
Steve.

Petko Delchev
Courses Plus Student 2,103 PointsThere is now way I can ever do this by my own...

Matthew Schwer
Python Web Development Techdegree Student 975 PointsYa I feel like I have to cheat my way through this whole course... They said immerse yourself so maybe this is the immersion aspect? Maybe this just isn't for me. Its like when I read the question the coding aspects completely elude me. Then as soon as I see the solution it makes sense. Am I the only one who feels they are cheating their way through?
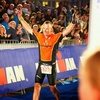
Steve Hunter
57,712 PointsMatthew - this is very common. Don't worry about it; your brain is working fine! It will come in time after a number of repetitions. The concepts are new and quite esoteric so it takes time to adjust. Give it that time and don't stress about it. You'll have your moment when, all of a sudden, it just makes sense! I found reading through questions and answers in the Community pages helped me - seeing how other people articulate both their problem and the solution helped fix the concept inn my head.
Good luck!
Steve.

bryantan4
Courses Plus Student 1,391 PointsThanks a lot steve
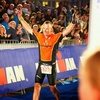
Steve Hunter
57,712 Points
Morris Pietrobon
2,537 Pointsthanks loads, really helped

Mark Tripney
6,009 PointsI had a solution very similar to Steve's, above, but using *args
...
def combo(*args):
new_list = []
for i in range(0, len(args[0])):
new_list += (args[0][i], args[1][i]),
return new_list
print(combo([1, 2, 3], "abc"))
I've got a little (a very little) experience with JavaScript, and this solution presented itself to me as quite a common JS pattern, iterating through items using a counter... It's something that I've seen come up quite early in a lot of JS courses, but not so much with Python. Hmm.
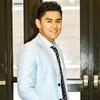
Francisco Ozuna
21,453 Pointsdef combo(iterable1, iterable2):
# creates an empty list which will hold the list of tuples
list_of_tuples = []
# Since we can assume the iterables will be the same length we use
# the enumerate keyword to give us an index
for index, item in enumerate(iterable1):
# uses the index variable that the enumerate keyword gives us
# to pull the item at that index for each iterable
# creates a tuple which includes those items and adds the tuple
# to our empty list, list_of_tuples
list_of_tuples += [ (iterable1[index], iterable2[index]) ]
return list_of_tuples
# hope this helps!

f4u57
6,743 PointsHere is how I did it
def combo(iterable_1,iterable_2):
list_of_tuples = []
for index, item in enumerate(iterable_2, start = iterable_1[0]):
list_of_tuples.append((index,item))
return list_of_tuples

Jassim Alhatem
20,883 PointsThat was way more understandable, thanks!

Jassim Alhatem
20,883 Pointsbut why did you throw in 'start = iterable_1[0]', can you please explain that me.
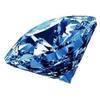
jalil damian
1,452 Pointstanks

Terry Felder
1,738 Pointsi ran combo('abc', 123) why does 123 increase 123,124,125
p.s
i just went back into my repl.it, removed item2 from enumerate and turned 123, into[1,2,3]...im not entirely sure why i did that but it worked.....i just want to be sure of what i am doing! Thank you for your time!
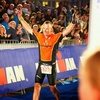
Steve Hunter
57,712 PointsHi Terry,
The enumerate
method does this. The counter variable is incremented at each loop. Have a look at this.
Steve.

Nataly Rifold
12,431 PointsHi, I don't understand why I need to base this code on index. The iterables are ordered iterables so why use range? is there a simpler way?
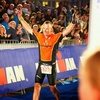
Steve Hunter
57,712 PointsHi Iris,
I'm not saying you need to base this solution on index
- this was just my solution. I don't see a better way but I'm sure there is one; I am not a Python programmer!
The reason I used an index
was to ensure we were accessing the same element of both iterables within each repetition of the loop.
Steve.

ADE YALSHIRE
18,049 PointsOf course, there is a lot of place for improvement
def combo(myString1,myString2):
myList=[]
if len(myString1)==len(myString2):
recup1=0
while recup1 < len(myString1) :
mytuple=()
myTuple=(myString1[recup1],myString2[recup1])
myList.append(myTuple)
recup1+=1
return myList

Jatin Mehta
13,169 Pointsdef combo(lists,string): f_list=[] f_tuple=() for i in range(0,len(lists)): f_tuple=(lists[i],string[i]) f_list.append(f_tuple) return f_list

Corey Maller
4,076 Pointsdef combo(x,y): print(set(zip(x,y)))

Terry Felder
1,738 Pointsdef combo(item1,item2):
new_list = []
for a, b in enumerate(item1,item2):
together = a,b
new = tuple(together)
new_list.append(new)
return new_list
print(combo('abc',123))
i know this is wrong but can someone explain why my numbers increased?
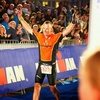
Steve Hunter
57,712 PointsHi Terry,
Can you explain what your code did that you hadn't expected it to do?
Steve.
Ben Fairchild
10,108 PointsBen Fairchild
10,108 PointsSteve,
This was insanely helpful. I loved the explanation, thank you for taking the time to do that!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem!

sajjan poudel
447 Pointssajjan poudel
447 PointsSteve Hunter
output += (iter1[i], iter2[i]),
why have you added comma at the last what happens if we don't use it
whats the difference ?????
Jeremy Nootenboom
Python Web Development Techdegree Student 6,876 PointsJeremy Nootenboom
Python Web Development Techdegree Student 6,876 PointsI would like to know the answer to Sajjan's question as well. Is the presence of the comma what makes it a tuple? I thought this was already accomplished with the parenthesis and comma in "(iter1[i], iter2[i])"
Andrew Wiegerig
4,754 PointsAndrew Wiegerig
4,754 PointsJeremy Nootenboom
Without the comma you just get a list in return rather than a list of tuples.
Maybe this is more explicit:
output += ((a[i], b[i]),)
anthony pizarro
1,983 Pointsanthony pizarro
1,983 Points// for i in range in range (0, len(iter1)):// for this code to work wouldn't you have to import a package?