Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial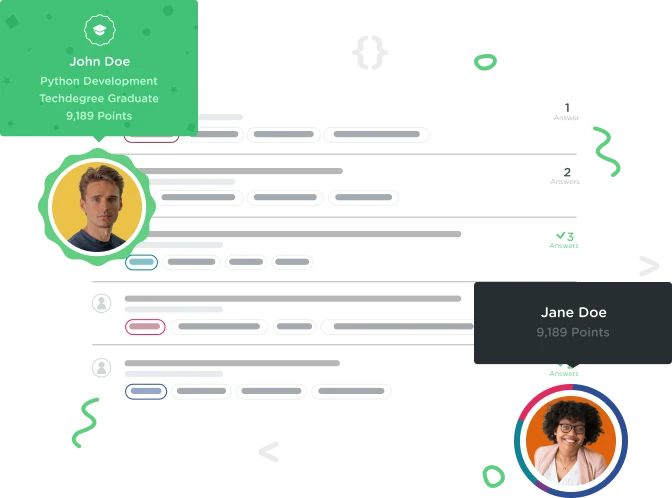

shobha gunupuru
1,137 PointsAlter the event handler on line 4 to run when the form is submitted, not just when the submit button has been clicked. L
how to solve this
const form = document.querySelector('form');
const submitButton = form.querySelector('[type=Submit]');
submitButton.addEventListener('click', (e) => {
form.preventDefault();
});
<!DOCTYPE html>
<html>
<head>
<title>Submit Event</title>
</head>
<body>
<form>
<label>Name:</label>
<input type="text" name="name">
<input type="Submit" name="submit" value="Submit">
</form>
<script src="app.js"></script>
</body>
</html>
5 Answers
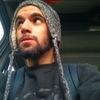
Cosimo Scarpa
14,047 PointsNeed to be
const form = document.querySelector('form');
const submitButton = form.querySelector('[type=Submit]');
form.addEventListener('submit', () => {
});
You're adding the EventListener to the form element instead of the submit button, also you need submit and not click.
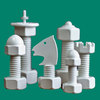
Steven Parker
229,744 PointsYou need to make two changes to how this event listener is attached.
First, you would want to attach the listener to the form itself instead of the button. Then, you would want to change the event type (the first argument to addEventListener) from "click" to "submit".
Also, it looks like you already have code added for task 2. Did you pass task 1 already somehow?
But your task 2 code isn't quite right. You have the right idea in calling preventDefault, but it should be applied to the event object (e) instead of the form.

vremea stra
Full Stack JavaScript Techdegree Student 110 Pointsconst form = document.querySelector('form'); const submitButton = form.querySelector('[type=Submit]');
form.addEventListener('submit', (e) => { e.preventDefault();
});
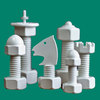
Steven Parker
229,744 PointsYou forgot to format it, but yes, that's what it would look like after you apply the hints I gave.
When posting code, always format using the instructions in the Markdown Cheatsheet pop-up, found below the "Add an Answer" area

vikram 11023
12,043 PointsAlter the event handler on line 4 to run when the form is submitted, not just when the submit button has been clicked. Leave the callback function empty for now.
const form = document.querySelector('form'); const submitButton = form.querySelector('[type=Submit]');
form.addEventListener('submit', (e) => {
const text = input.value;
input.value = '';
});

Edward Ries
7,388 PointsI could be totally wrong here but I'm thinking it's something like this:
form.addEventListener(submit, (e) => {
form.preventDefault(); // this line is preventing submit
});

Edward Ries
7,388 PointsI just checked the challenge and that worked fine. I did leave out the form.preventDefault(); since that wasn't needed.