Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial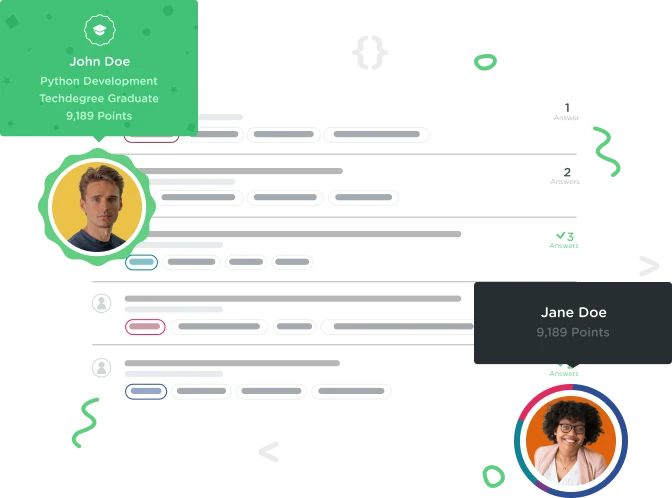

Alexisca hall
23,683 PointsAlter the event handler on line 4 to run when the form is submitted, not just when the submit button has been clicked. L
Alter the event handler on line 4 to run when the form is submitted, not just when the submit button has been clicked. Leave the callback function empty for now.
const form = document.querySelector('form');
const submitButton = form.querySelector('[type=Submit]');
submitButton.addEventListener('click', () => {
});
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>submit demo</title>
<style>
p {
margin: 0;
color: blue;
}
div,p {
margin-left: 10px;
}
span {
color: red;
}
</style>
<script src="https://code.jquery.com/jquery-1.10.2.js"></script>
</head>
<body>
<p>Type 'correct' to validate.</p>
<form action="javascript:alert( 'success!' );">
<div>
<input type="text">
<input type="submit">
</div>
</form>
<span></span>
<script>
$( "form" ).submit(function( event ) {
if ( $( "input:first" ).val() === "correct" ) {
$( "span" ).text( "Validated..." ).show();
return;
}
$( "span" ).text( "Not valid!" ).show().fadeOut( 1000 );
event.preventDefault();
});
</script>
</body>
</html>
3 Answers
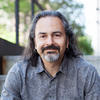
Michael Nicholas
22,697 PointsLine 4 should read:
form.addEventListener('submit', () => {
As Danielle mentioned, you're adding the event listener to the form element instead of the submit button, and changing the event from 'click' to 'submit'.

Daniele Gubbiotti
24,505 PointsHi, you have to shift the focus on the form by selecting the form itself and then change the event type paying attention to lower case of submit type. Hope i was helpful ;)
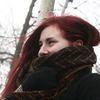
Ella Ruokokoski
20,881 PointsYou shouldn't add any code to the html file. Just edit the code in the app.js file.