Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial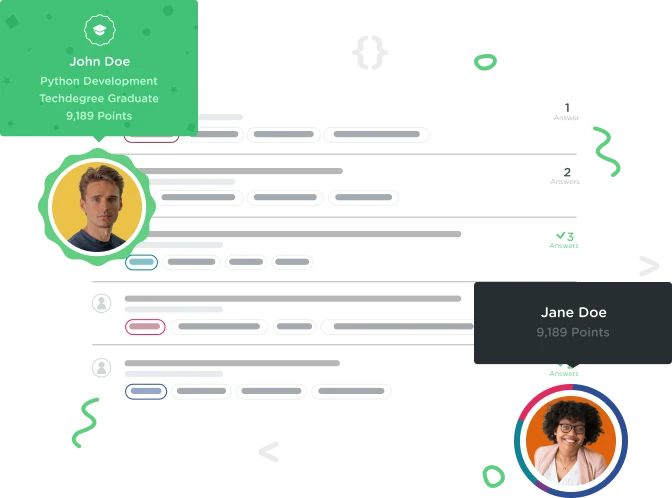

JASON LEE
17,352 PointsAlternate solution using Promises instead of async/await
Using Async await
// Find a record
const toyStory = await Movie.findByPk(1);
// Delete a record
await toyStory.destroy();
Using Promise (with catch clause)
// Find and Delete a record
Movie.findByPk(5).then(result => result.destroy())
.catch(()=>{console.log("An error occurred in destroying the record.")});
My (newbie) understanding is that async-await is newer and supersedes Promises, but it's good to be familiar with both. I noticed a key difference here is that with async-await -> when a Primary Key record doesn't exist in the database, the program will crash. However with Promises, the .catch()
clause can prevent crashing.
1 Answer
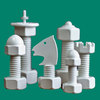
Steven Parker
231,236 PointsThe async/await syntax is used with promises, it does not superseded them. But you're right about it being newer, promises were released first and required complicated chaining to achieve the same functionality that async/await now simplifies.