Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial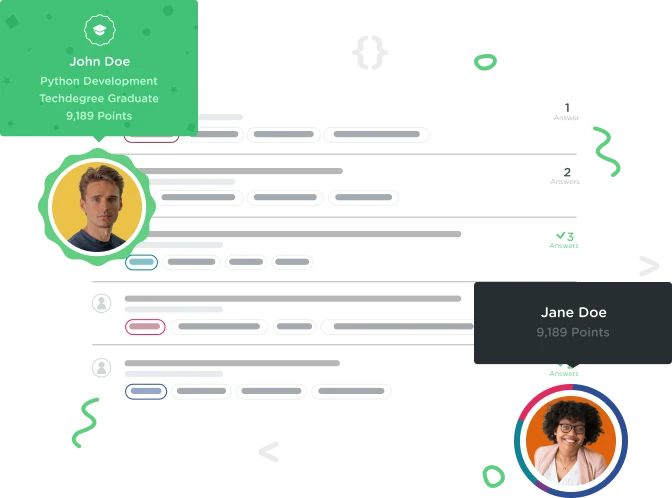
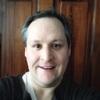
givens
7,484 PointsAlternative for loop
Alternative loop statement:
for i in range(len(shopping_list)):
print("{}. {}".format(i, shopping_list[i]))
Does this seem cleaner?
1 Answer
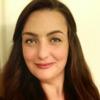
Jennifer Nordell
Treehouse TeacherHi there! I actually really like that and do think it looks a bit cleaner. That being said, it doesn't really function like the one in the video as it will start the numbering at 0. So assuming the first item in the shopping list is "bread", you will see output like:
0. bread
instead of...
1. bread
But that is easily fixed! You can actually include a + 1
inside the print
statement to take care of that. Take a look:
print("{}. {}".format(i + 1 , shopping_list[i]))
But I dare say you could even make that a little cleaner, and it is supported in workspaces but likely will not be introduced until later down the line. It's called an "f string". You can get that same output with this line. Take a look:
print(f"{i+1}. {shopping_list[i]}")
I think that last line is about as concise as you're going to get it. Hope this helps and way to ask great questions!
edited for additional information
I don't really think you're at this stage yet, but you could also use the builtin function enumerate
here. You could rewrite that for
loop like so:
for index, value in enumerate(shopping_list, 1):
print(f"{index}. {value}")
This takes every index and its corresponding value and prints them until it reaches the length of shopping_list
. The 1 at the end of the arguments is the offset for the starting value of the index
.