Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial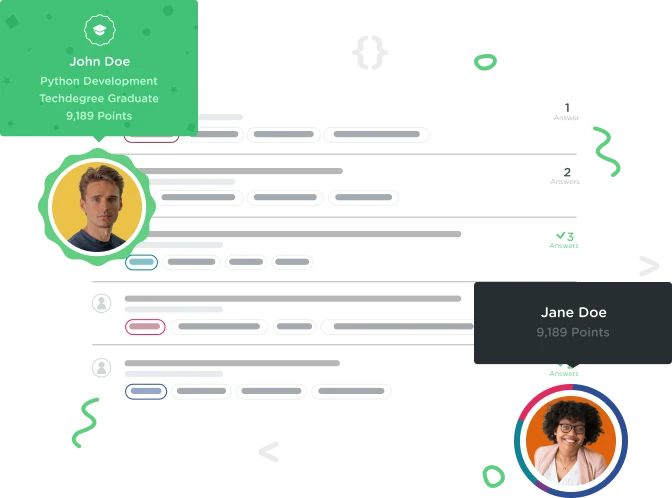
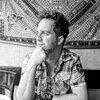
jlampstack
23,932 PointsAlternative Render Solution???
I find it cleaner and easier to understand if we are to getRecipeTitles() in the Render display instead of when we call the Render.
public static function listRecipeTitles($collection)
{
$titles = $collection->getRecipeTitles();
asort($titles);
return implode("<BR>", $titles);
}
This way when we call the Render we only need
echo Render::listRecipeTitles($cookbook);
Instead of
echo Render::listRecipeTitles($cookbook->getRecipeTitles());
Is there a downside to doing it this way?
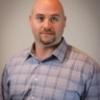
Ben Payne
1,464 PointsI'll throw in there that doing it your way would mean that $collection
would always have to have the getRecipeTitles()
method. So, an even better way would be to use an interface and rely on that. So that if a cookbook, or a stack of index cards, or some other collection were passed in you know they will implement that contract.
<?php
interface RecipeCollectionContract
{
public function getRecipeTitles();
}
class Cookbook implements RecipeCollectionContract
{
public function getRecipeTitles() // throws fatal if not implemented
{
// return titles
}
}
class IndexCards implements RecipeCollectionContract
{
public function getRecipeTitles() // throws fatal if not implemented
{
// return titles
}
}
class Render
{
public static function listRecipeTitles(RecipeCollectionContract $collection) // $collection must be an implementation of the contract
{
$titles = $collection->getRecipeTitles(); // Whatever is passed in adheres to the contract.
asort($titles);
return implode("<BR>", $titles);
}
}
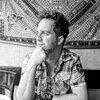
jlampstack
23,932 PointsBen Payne , Excellent points. Thank you.
This is the reason I posted this question for insight that might be above my understanding. I haven't learned about contracts or implementing yet. I guess I will cross that bridge when I get there. If you're able to repost your thoughts as an answer, instead of a comment, I can mark it as best answer and we can close this topic.
Many Thanks!
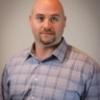
Ben Payne
1,464 PointsAll set. Good on you for getting out of your comfort zone.
_Ben
1 Answer
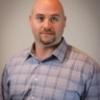
Ben Payne
1,464 PointsPer the D (Dependency Inversion Principle) in SOLID it might be better use an interface (abstraction) and rely on that. So that if a cookbook, or a stack of index cards, or some other collection were passed in you know it will implement that contract.
<?php
interface RecipeCollectionContract
{
public function getRecipeTitles();
}
class Cookbook implements RecipeCollectionContract
{
public function getRecipeTitles() // throws fatal if not implemented
{
// return titles
}
}
class IndexCards implements RecipeCollectionContract
{
public function getRecipeTitles() // throws fatal if not implemented
{
// return titles
}
}
class Render
{
public static function listRecipeTitles(RecipeCollectionContract $collection) // $collection must be an implementation of the contract
{
$titles = $collection->getRecipeTitles(); // Whatever is passed in adheres to the contract.
asort($titles);
return implode("<BR>", $titles);
}
}
Now the listRecipeTitles
method has a dependency however its an abstraction. It doesn't know or care what is passed in just that it has the getRecipeTitles()
method.
jamesjones21
9,260 Pointsjamesjones21
9,260 PointsI think I share you verdict on this as it is easier to understand it your way when putting the method within the static method.
but I think Aleana is just stating what you can do, by naming the object and then passing it to the method.