Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial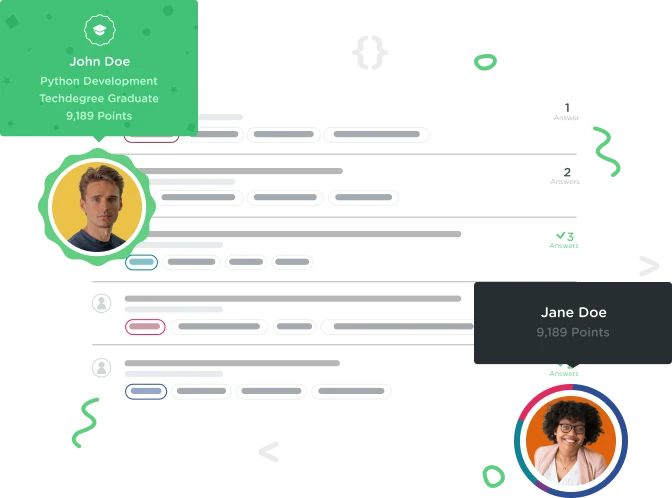
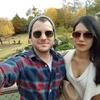
Jamie Gobeille
Full Stack JavaScript Techdegree Graduate 19,573 PointsAlternative solution
I found it to read a little better to not have to do nested if statements in the event listener that determines what button is pressed and what action to proceed with.
I just assigned the buttons a class name when buttons are generated.
const editButton = document.createElement('button');
editButton.textContent = 'edit';
editButton.className = 'edit';
li.appendChild(editButton);
const removeButton = document.createElement('button');
removeButton.textContent = 'remove';
removeButton.className = 'remove';
li.appendChild(removeButton);
Then instead of doing a two-step check process you only need to check for the class name. it reduces some code and is a little easier to read in my opinion.
ul.addEventListener('click', (e) => {
const button = e.target.className;
if(button === 'remove'){
const li = e.target.parentNode;
const ul = li.parentNode;
ul.removeChild(li);
} else if(button === 'edit') {
console.log('edit mode');
}
});
Does anyone see a problem in doing it this way?
1 Answer
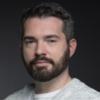
Cory Harkins
16,500 PointsHi Jamie!
Long answer short: No, there's nothing wrong with how you are accessing the buttons!
You could also use my personal fav in this situation: switch
statements.
ul.addEventListener('click', (e) => {
const button = e.target;
switch(button.className) {
case 'remove':
const li = button.parentNode;
const ul = li.parentNode;
ul.removeChild(li);
return;
case 'edit':
console.log('edit mode');
return;
default:
console.log('handle a default case');
return;
}
});