Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial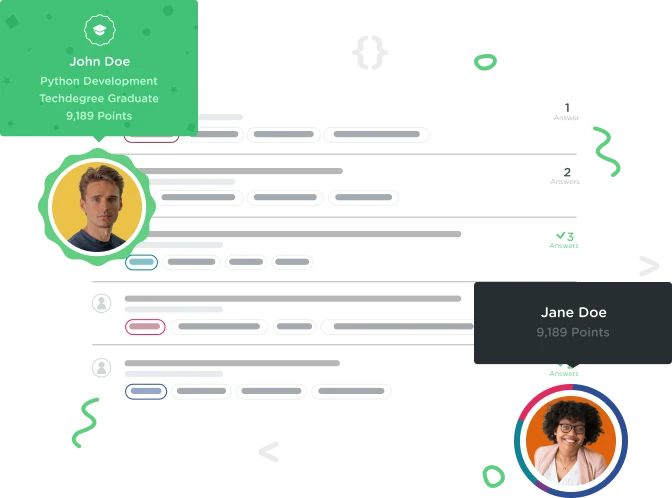
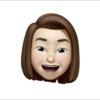
kg10
7,911 PointsAlternative solution
I still find it very hard to understand if alternative solutions are inefficient in some way or it's just it - an alternative answer... Any tips are very much appreciated!
let quiz = [
['Which country does opera singer Pavarotti come from?', 'Italy'],
['Which swimming stroke is named after an insect?', 'Butterfly'],
['What is a female deer called?', 'Doe']
];
let guess;
let correct = [];
let wrong = [];
function print(message) {
let outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function printAnswers(answer) {
let listHTML = '<ol>';
for (let i = 0; i < answer.length; i += 1) {
listHTML += '<li>' + answer[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
for (let i = 0; i < quiz.length; i += 1) {
guess = prompt(quiz[i][0]);
guess = guess.toUpperCase();
if ( guess === quiz[i][1].toUpperCase() ) {
correct.push(quiz[i][0]);
} else {
wrong.push(quiz[i][0]);
}
}
html = `<p>You got ${correct.length} question(s) right.</p>`;
html += `<h2>You got these questions correct:</h2>`;
html += printAnswers(correct);
html += `<h2>You got these questions wrong:</h2>`;
html += printAnswers(wrong);
print(html);
2 Answers

Zimri Leijen
11,835 PointsIn most cases it hardly matters.
unless you're working with really big data, or write extremely inefficient code, the processing power we have available nowadays makes it pretty irrelevant.
In most cases, developer time is more valuable than process time, in other words, the time a developer would spend to optimize the program is more expensive than the hypothetical time saved running the program.
There are of course cases where optimization matters, but you probably wouldn't be using javascript there anyway.

James Croxford
12,723 PointsMine is similar to yours. I used the same prompt style as yourself (not creating a separate variable).
guess = prompt(quiz[i][0]);
I added a few extras (such as checking for blank responses from the user), but the main difference from for me was using template literals over standard string literals, as to me they're neater and more readable.
I think ultimately though as Zimri says most of the possible optimisations, whatever they may be, either I'm not aware of yet, aren't necessary for this type of basic JS file, or in some cases can offer a trade off (less readable code to others/yourself upon returning to it)?

Zimri Leijen
11,835 Pointsreadability and maintainability are indeed often the most important thing to keep in mind.