Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial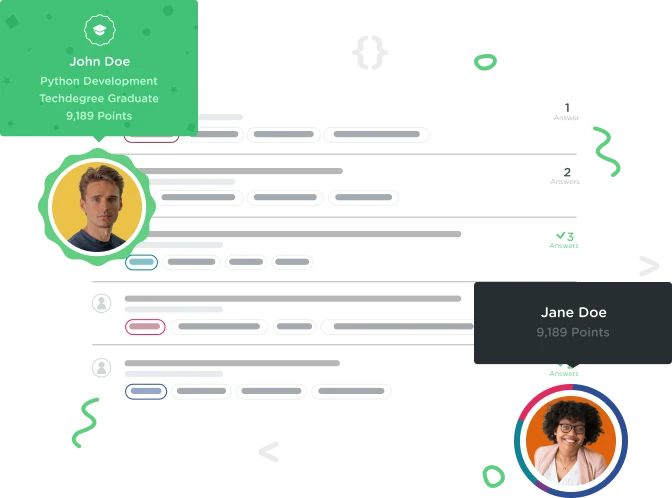
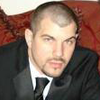
Gary Calhoun
10,317 PointsAlternative to pop up boxes to ask questions?
Hi I was wondering how could I list the question on the page itself using document.write and have it so a user can input in text fields instead of the floating pop up boxes? I know I probably need to create a form in the html code and then use concatenation to link it all together something to that extent maybe?
3 Answers

LaVaughn Haynes
12,397 PointsHere is one way you could do it
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div id="quiz">
<div class="question"></div>
<div class="answer">
<input type="text">
<button>Submit Answer</button>
</div>
</div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script>
//questions array
var questions = [
['What company makes the iPhone?', 'Apple'],
['What company makes PS4?', 'Sony'],
['what company makes Xbox One?','Microsoft']
];
//vars and cache elements
var $quiz = $('#quiz'),
$question = $quiz.find('.question'),
$answer = $quiz.find('.answer input'),
$submit = $quiz.find('.answer button'),
totalCorrect = 0,
totalWrong = 0,
currentQuestion = 0,
correctAnswerMessage = "Number of correct answers: ";
//output the question
function askNextQuestion(){
var theQuestion = questions[currentQuestion][0];
$question.html(theQuestion);
$answer.val('').focus();
}
//check the answer
function checkAnswer(){
//get the answer from the field
var theAnswer = $answer.val();
//+1 to totalCorrect tally if correct
if( theAnswer.toLowerCase() === questions[currentQuestion][1].toLowerCase() ) {
totalCorrect++;
}
//move focus to the next question
currentQuestion++;
//if not the last question ask the next, else game over
if(currentQuestion < questions.length){
askNextQuestion();
}else{
displayScore();
}
}
//game over! display score
function displayScore(){
$quiz.html( correctAnswerMessage + totalCorrect );
}
//listen for answer submissions
$submit.on('click', checkAnswer);
//ask the first question
askNextQuestion();
</script>
</body>
</html>

LaVaughn Haynes
12,397 PointsWhen you use the $() selector you are searching the whole document. Find lets you narrow the search to a specific area instead of searching the whole document.
$('#haystack').find('.needle');
For example I can have the needle class through my whole document, I'm saying just search within the element with the id of haystack
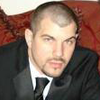
Gary Calhoun
10,317 PointsAh I see so $() is a more search everything and anything kind of thing but the find function helps zero in on specific area, nice! How does this method differentiate from the document.getElementById('my_header') for example?

LaVaughn Haynes
12,397 PointsjQuery is just a simple interface for regular javascript. When you use $() jQuery looks at your query and is smart enough to check if you are searching for a class $('.myClass') or an ID $('#myID') or element $('div') and then it calls the appropriate javascript. So $('#my_header') is pretty much the same as getElementById('my_header'). It's just easier to write $('#my_header') and have jQuery translate it to regular javascript
If you download and open the development version of jQuery you can see the regular javascript. https://jquery.com/download/
One good use of "find" (from my experience) is that you don't want to keep searching the whole document. That uses a lot of resources. So you can save your search by caching it in a variable like this
var $savedSearch = $('#my_header');
Now if you want to look for something inside your header you can use just say
var subSearch = $savedSearch.find('.someClass');
You can also search the whole document again, but why do that if you don't need to?
var subSearch = $('#my_header .someClass');
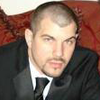
Gary Calhoun
10,317 PointsOh nice I didn't realize you can zero in on parent to child elements powerful stuff. Thanks for all the examples really appreciate it.
Gary Calhoun
10,317 PointsGary Calhoun
10,317 PointsWow awesome! So the find function is looking for the class or id in html document?