Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial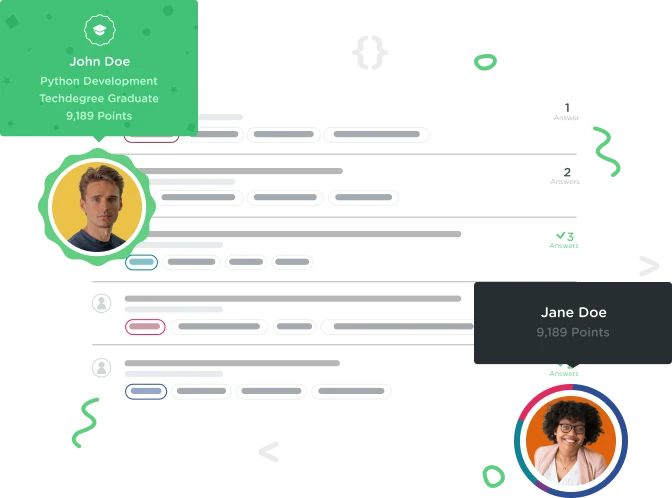

Raghda Talaa't
2,944 PointsAlternative to the DateUtils
This helper class is working well, use it instead
(From the Google I/O 2012 App):
/*
* Copyright 2012 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
private static final int SECOND_MILLIS = 1000;
private static final int MINUTE_MILLIS = 60 * SECOND_MILLIS;
private static final int HOUR_MILLIS = 60 * MINUTE_MILLIS;
private static final int DAY_MILLIS = 24 * HOUR_MILLIS;
public static String getTimeAgo(long time, Context ctx) {
if (time < 1000000000000L) {
// if timestamp given in seconds, convert to millis
time *= 1000;
}
long now = getCurrentTime(ctx);
if (time > now || time <= 0) {
return null;
}
// TODO: localize
final long diff = now - time;
if (diff < MINUTE_MILLIS) {
return "just now";
} else if (diff < 2 * MINUTE_MILLIS) {
return "a minute ago";
} else if (diff < 50 * MINUTE_MILLIS) {
return diff / MINUTE_MILLIS + " minutes ago";
} else if (diff < 90 * MINUTE_MILLIS) {
return "an hour ago";
} else if (diff < 24 * HOUR_MILLIS) {
return diff / HOUR_MILLIS + " hours ago";
} else if (diff < 48 * HOUR_MILLIS) {
return "yesterday";
} else {
return diff / DAY_MILLIS + " days ago";
}
}
1 Answer

Andres Soto
3,521 PointsI am using joda library to do this date stuff, it is very helpful and just wanted to throw it out there for anyone wanting to use it instead.
You just have to add this line in your dependencies
compile 'joda-time:joda-time:2.8.2'
And then it will work, my code for setting the time is below
Date now = new Date();
DateTime nowDateTime = new DateTime(now);
DateTime messageDateTime = new DateTime(message.getCreatedAt());
String timeDifference = "";
if(Minutes.minutesBetween(messageDateTime, nowDateTime).getMinutes() == 0){
timeDifference = "Just sent";
}
else if(Minutes.minutesBetween(messageDateTime, nowDateTime).getMinutes() == 1){
timeDifference = Minutes.minutesBetween(messageDateTime, nowDateTime).getMinutes() + " minute ago";
}
else if(Minutes.minutesBetween(messageDateTime, nowDateTime).getMinutes() < 60){
timeDifference = Minutes.minutesBetween(messageDateTime, nowDateTime).getMinutes() + " minutes ago";
}
else if (Hours.hoursBetween(messageDateTime, nowDateTime).getHours() == 1){
timeDifference = Hours.hoursBetween(messageDateTime, nowDateTime).getHours() + " hour ago";
}
else if (Hours.hoursBetween(messageDateTime, nowDateTime).getHours() < 24){
timeDifference = Hours.hoursBetween(messageDateTime, nowDateTime).getHours() + " hours ago";
}
else if (Days.daysBetween(messageDateTime, nowDateTime).getDays() == 1){
timeDifference = Days.daysBetween(messageDateTime, nowDateTime).getDays() + " day ago";
}
else{
timeDifference = Days.daysBetween(messageDateTime, nowDateTime).getDays() + " days ago";
}
holder.timeLabel.setText("~" + timeDifference);
Daniel Whitaker
10,996 PointsDaniel Whitaker
10,996 PointsThanks, it does work and I like that I can see how it converts the time differences to messages.
I just had to replace long now = getCurrentTime(ctx); with long now = new Date().getTime();