Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial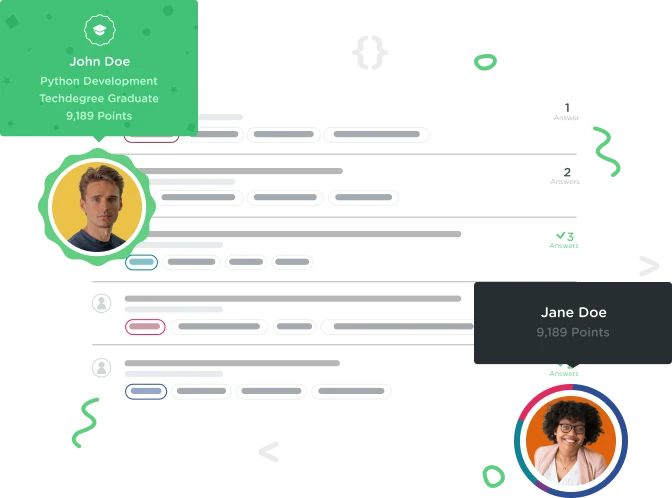

Lucas Atayde
6,784 PointsAlternative ways of getting the Icon Id?
This is more of a java question but I think it would be good to know. I am also aware you could also use a switch statement to set the iconId. I am wondering how would you deal with this type of problem if you had hundreds or thousands of icons? Writing that long of an if else or switch statement seems like it would take way too long and be very bulky.
4 Answers
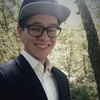
Jacob Bergdahl
29,119 PointsIt's up to personal preference whether you want to use an if/else statement or a switch statement, however for these matters a switch statement is typically recommended. Now, if you had hundreds or thousands of icons, you could use a different naming convention and assign them in a for-loop instead, using an array of images and an array of ids!

Sam McDavid
20,242 Points public int getIconId() {
HashMap<String, Integer> icons = new HashMap<String, Integer>();
icons.put("clear-day", R.drawable.clear_day);
icons.put("clear-night", R.drawable.clear_night);
icons.put("rain", R.drawable.rain);
icons.put("snow", R.drawable.snow);
icons.put("sleet", R.drawable.sleet);
icons.put("wind", R.drawable.wind);
icons.put("fog", R.drawable.fog);
icons.put("cloudy", R.drawable.cloudy);
icons.put("partly-cloudy-day", R.drawable.partly_cloudy);
icons.put("partly-cloudy-night", R.drawable.cloudy_night);
return (icons.get(mIcon) != null) ? icons.get(mIcon) : R.drawable.clear_day;
}
This is store all of the possible variations to be used in a HashMap with the string value from the forecast API as the key and the drawable id as the value. The ternary return
statement reads as "If I have an icon set, give me the icon else, give me the clear_day icon."

Samuel Tissot-Jobin
7,350 PointsI was a little bothered with the if/else if statement as well so this is what I did:
I declared a static member viable to hold that data ( did it as a member variable for easy alteration if other icon are added in the future):
private static String[] mIconPossibilities = {"clear-day", "clear-night", "rain", "snow",
"sleet", "wind", "fog", "cloudy", "partly-cloudy-day", "partly-cloudy-night"};
---------------------the function--------------------------
public int getIconId(Context context){
//initialize value with default clear-day
int iconId = R.drawable.clear_day;
//check for matches
if (Arrays.asList(mIconPossibilities).contains(mIcon)){
String formatted = mIcon.replace("-", "_");
String uri = "@drawable/"+formatted;
iconId = context.getResources().getIdentifier(uri, null, context.getPackageName());
}
return iconId;
}
----------------------------------the call----------------
//need to be call from an activity
getIconId(this);
---------------NOTE --- UNSURE----- QUESTION !! ----------------------
I am not totally certain if this would be a more efficient way. Does passing the context around is a good idea? I don't know.
the functions: getResources, getIdentifier, getPackageName and replace are probably more resource intensive than a simple switch or if/else if.
thoughts on this anyone ?
cheers

Samuel Tissot-Jobin
7,350 PointsAlso I have noticed that the naming convention although almost exact, does not always match. you should change the image file name in the drawable folder from:
cloudy_night TO partly_cloudy_night
partly_cloudy TO partly_cloudy_day
in order to match the response from forecast.
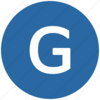
Gerardo Guzman
4,480 PointsI need to be able to access to the precise image resource among 200, so I've tried to implement this approach, but I had problem passing context.
Now, I am trying to adapt this solution:

Samuel Tissot-Jobin
7,350 Pointshello for context be careful if you are in an inline function something like:
Let say that the below code is in the class that extend Activity like so
public class MainActivity extends Activity {
//doing stuff here
}
yourButtonName.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// YOU WANT TO PASS CONTEXT LIKE THIS
yourPassingContextFcnt(MainActivity.this);
}
});
there is also the context class :http://developer.android.com/reference/android/content/Context.html
Hope that help I am far from an expert. so if I'm wrong please correct me.
cheers