Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial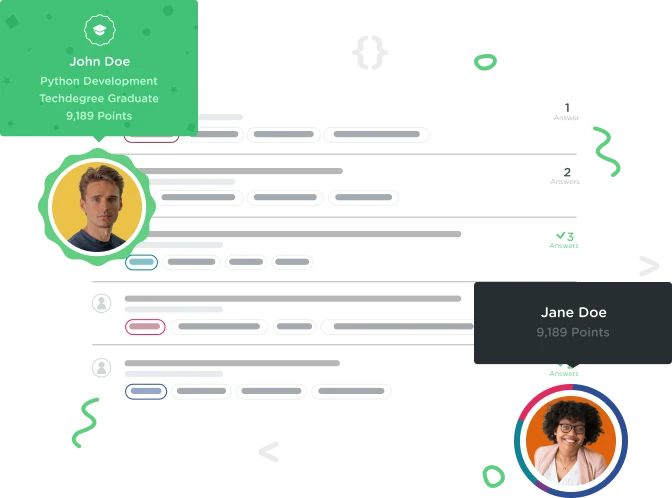

Tiago Ramos
2,380 PointsAm I acessing the values in the array correctly?
Hi,
I tried to solve this problem in two ways, using a for() loop and another way using a foreach() loop (commented bellow)
It seems to me that it should work, can you help me?
Thanks :)
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
int i = 1;
//use a for loop
for(i=0; i < sequence.Length; i++)
{
// comparing the actual value in the array with the previous one
if(i > 0 && sequence[i] == sequence[i-1])
{
return true;
}
else
{
return false;
}
}
/*
// use a foreach loop
foreach(int val in sequence)
{
// comparing the actual value in the array with the previous one
if( sequence[i] == sequence[i-1] )
{
// return true if it is the same
return true;
}
else
{
return false;
}
i++;
}
*/
}
}
}
1 Answer

andren
28,558 PointsThere are two issues, one code issue and one logic issue. Both of them has to do with how you define your return
statements.
In C# all (non void
) methods have to return a value. If C# detects that there is a possible scenario where a method will not return a value, then the code will not compile.
Now what will happen if your method is sent an empty list? The length of it will be 0, so your loop will never run. And since you don't have any return
statements outside the loop, your method would not actually return anything. This is why your code does not compile.
The second issue is the logic of your conditional statement. Let's say I sent in a list that looks like this : [1, 2, 3, 3]
the method should return true
for that list, but your method would return false
.
During the first iteration of the loop it would compare 1
and 2
and find that they are not equal, and then it would run the else
statement which returns false
. The return
statement causes a method to return a value and then exit. So the loop never ends up continuing past that first iteration.
The solution to both of the those issues is to move the return false
statement outside of the loop. Like this:
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
//use a for loop
for(int i=0; i < sequence.Length; i++)
{
// comparing the actual value in the array with the previous one
if (i > 0 && sequence[i] == sequence[i-1])
{
return true;
}
}
return false;
}
}
}
That way your function will always return something. And when two numbers don't match the loop will simply continue to its next iteration. rather than returning right away.
Tiago Ramos
2,380 PointsTiago Ramos
2,380 PointsThanks andren :) now i totally understand where I went wrong! your help was priceless!