Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial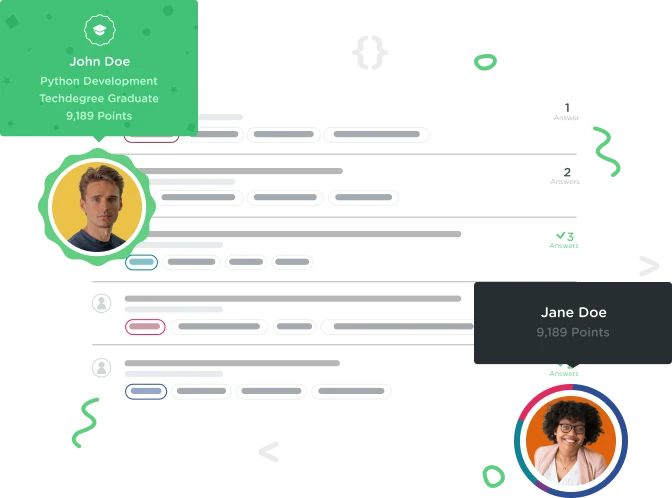

Tiago Ramos
2,380 PointsAm I finding the index correctly?
Hi friends,
I thought about getting the middle index, so I can slice the first and second half of the string, upper and lowercase them, concatenate them, and finally return the string.
Where am I going wrong? Thanks
def sillycase(string):
# find the middle index of the string
mid_index = string.length() // 2
# slice the first and second half of the string
first_half = string.slice[mid_index::-1]
second_half = string.slice[mid_index::]
# upper and lower case
lower_case = first_half.lower()
upper_case = second_half.upper()
# concatenate
string_case = lower_case + upper_case
return string_case
2 Answers
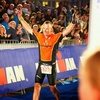
Steve Hunter
57,712 PointsHi again,
Also, your slicing isn't quite right.
You've got mid_index
now. So use string[:mid_index]
for the first half and string[mid_index:]
for the last half. You don't need to call the slice method (I don't know if it exists!) - the square brackets do that for you.
Amending your code gives you:
def sillycase(string):
# find the middle index of the string
mid_index = len(string) // 2
# slice the first and second half of the string
first_half = string[:mid_index]
second_half = string[mid_index:]
# upper and lower case
lower_case = first_half.lower()
upper_case = second_half.upper()
# concatenate
string_case = lower_case + upper_case
return string_case
You can probably tidy it up a bit by removing the first_half
, second_half
, lower_case
and upper_case
variables and just returning the two slices with the right casing:
def sillycase(string)
mid_index = len(string) // 2
return string[:mid_index].lower() + string[mid_index:].upper()
But that maybe lacks clarity; there's a middle ground, perhaps.
Steve.
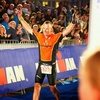
Steve Hunter
57,712 PointsHi Tiago,
It might be the way you're trying to get the length of the string.
Try passing the string as a parameter into the len()
method, like this:
mid_index = len(string) // 2
Let me know how you get on.
Steve.

Tiago Ramos
2,380 Pointshi steve thanks for the tip, but it still isn't working, do you think I need to round the mid_index? or is another block of the code that's wrong? (maybe the slices?)
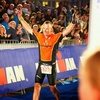
Steve Hunter
57,712 PointsYes, the slices - I added a second answer below 15 minutes ago.
Tiago Ramos
2,380 PointsTiago Ramos
2,380 Pointsthank you very much Steve :) it worked perfectly!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem! I hope you understood why it worked too.
Tiago Ramos
2,380 PointsTiago Ramos
2,380 Pointsyeah I understood well ;)
Steve Hunter
57,712 PointsSteve Hunter
57,712 Points