Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial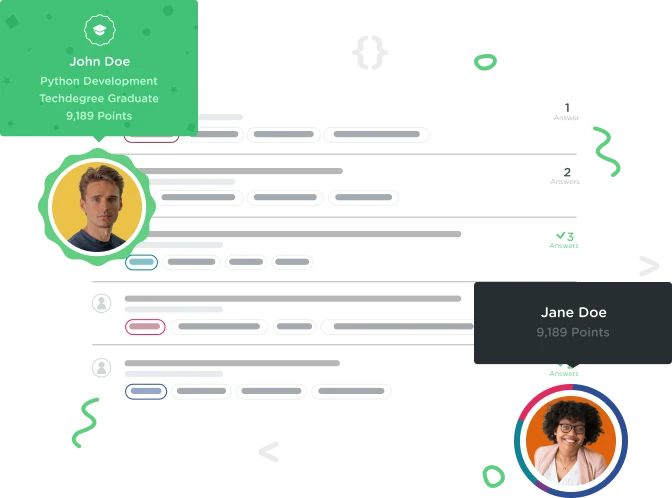
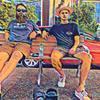
Frederick Bogdanoff
15,338 PointsAm I following this correctly? Or way off?
var userNumber = prompt('Give me a number!');
var userNumber2 = prompt('Give me another number!');
var num1 = parseInt(userNumber);
var num2 = parseInt(userNumber2);
var newNumber = Math.floor(Math.random() * 100) + 1 + num1 + num2;
document.write(newNumber);
Mod Note: Added Forum Markdown for the code
3 Answers
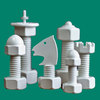
Steven Parker
231,268 PointsYou need to modify the formula slightly if you want to generate a random number between the input numbers. Assuming the first one will be the lower one:
var newNumber = Math.floor(Math.random() * (num2 - num1 + 1)) + num1;
And when posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

Neil McPartlin
14,662 PointsJonathan Grieve has correctly identified the troubled line 5, but the 2nd challenge is to generate a random number between the 2 numbers entered by a user. Currently your code arrives at a number that is too high. Jonathan has removed the * 100 which helps but there is more to do.
Think of it this way. Math.random() creates a random number between 0 and 1. Let's pretend its 0.4. Your revised code needs to achieve this.
The 2 numbers entered are say 40 and 50.
So we subtract 40 from 50 and get 10. We multiply 10 by 0.4 and get 4.
But to arrive at a random number between 40 and 50, we must add back the first number which is 40 to arrive at 44.
When I first did this challenge, I thought I needed to know which number was the lower and which the higher, but now I see it does not matter. So with the above example, had the numbers entered been switched i.e. 50 first then 40, the answer now would be 46 (Because now we 'add' -4 to 50).. But given we're looking for a random number, no problem.
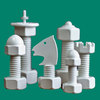
Steven Parker
231,268 PointsThe order does make a difference, because when the smaller value is entered first, the formula will generate a range that includes the endpoints Reversing the numbers gives you a smaller range that excludes the endpoints.

Neil McPartlin
14,662 PointsYes. Good point about whether you intend to include the end points. The resulting number is still positive though.
Edit: We both posted at the same time so I am referring to my own code.
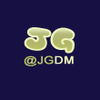
Jonathan Grieve
Treehouse Moderator 91,253 PointsYou're very very close.
The code could use a function or 2 but maybe you haven't got that far in the course yet.
You just need to amend your Random method slightly to do the right calculation, which I assume is adding the 2 numbers together, right?
var newNumber = Math.floor(Math.random() * 1) + num1 + num2;
By the way, don't forget to checkout the Markdown Basics course here on Treehouse for when you're adding code to your posts. Like I did with the line of code above. It'll help you write clearer code for the forums. Thanks :)
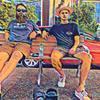
Frederick Bogdanoff
15,338 PointsHelped a lot! I’ll get on to that markdown down asap.
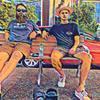
Frederick Bogdanoff
15,338 PointsMy bad. I didn't give it a close enough look, it kept giving me the same value. I'm a bit confused now about how this works. Perhaps I need to get myself a couple of math books. Steven your answer correct.
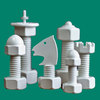
Steven Parker
231,268 PointsAnything multiplied by 1 stays the same, and since the random function generates floating point values less than 1, taking the floor of it will always yield 0.