Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial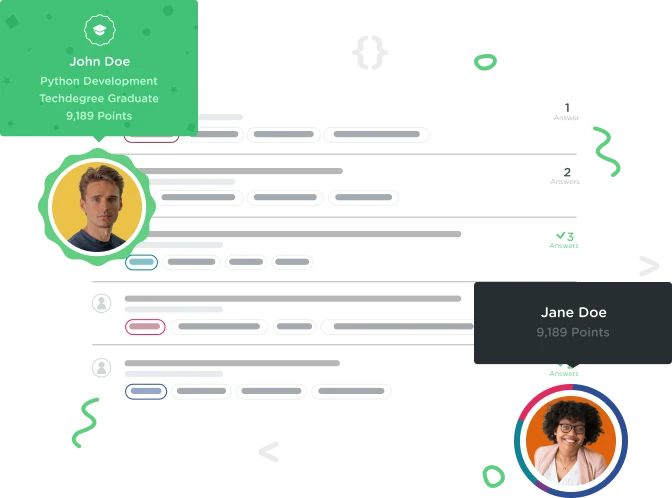

Michael Duncan
5,548 PointsAm I handling errors in my callback correctly in Node.js? (Error-first callback pattern)
TLDR: Am I handling errors in my callback function correctly?
<br>
<br>
I am working on a Node.js command-line application similar to the one in the exercise here. But I wanted to add more methods to the application and did not want to repeat the same request code in every one. So I've created a request helper method to handle requests using callbacks to pass the data around asynchronously (using this pattern).
If I follow this convention, however, each of my methods will all have the same bit of code to handle potential errors. Again, more repeated code that I feel like I should be avoiding.
Is this a correct way of doing this or is there a more efficient less repetitious way to handle the errors that are passed into my methods? Thanks for your help!
A method example:
ErgastAPI.prototype.seasonsTotal = function(callback) {
this._get(function(error, data) {
if (error) {
console.error(error);
return
}
callback(null, data.total);
});
}
All of my code:
"use strict";
var http = require('http');
var ErgastAPI = function() {
}
ErgastAPI.prototype._get = function(callback) {
http.get('http://ergast.com/api/f1/seasons2.json', function(res) {
var body = '';
res.on('data', function(chunk) {
body += chunk;
});
res.on('end', function() {
if (res.statusCode === 200) {
try {
var parsed = JSON.parse(body);
} catch(error) {
return callback('Unable to parse response as JSON. (' + error.message + ')');
}
callback(null, parsed.MRData)
} else {
callback('Error with request. (' + res.statusCode + ')');
}
});
}).on('error', function(error) {
callback('Error with the request. (' + error.message + ')');
});
}
ErgastAPI.prototype.seasonsTotal = function(callback) {
this._get(function(error, data) {
if (error) {
console.error(error);
return
}
callback(null, data.total);
});
}
var api = new ErgastAPI();
api.seasonsTotal(function(error, seasons) {
console.log('There have been ' + seasons + ' Formula 1 seasons.');
})
2 Answers
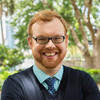
Andrew Chalkley
Treehouse Guest TeacherI prefer to use the EventEmitter pattern. Basically you emit events depending on what your code is doing and implement handlers to those events.
Take a look at my code here : https://github.com/treehouse/treehouse_blog.js and here : https://github.com/treehouse/treehouse_profile.js for inspiration.
Regards
Andrew

Michael Duncan
5,548 PointsThanks for the response Andrew, I will take a look at this pattern and see what I can do.
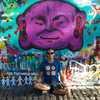
Andrew Stelmach
12,583 PointsI'm not brilliant with Javascript and Node, but it doesn't seem repetitious to me, because I can't think of a way to reduce the error handling down any more than you already have.
Colin Marshall
32,861 PointsColin Marshall
32,861 PointsThis might be a good question for Andrew Chalkley or @Huston Hedinger. For reasons unknown, Huston is not showing up in the list of names when I try to tag him in this post.