Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial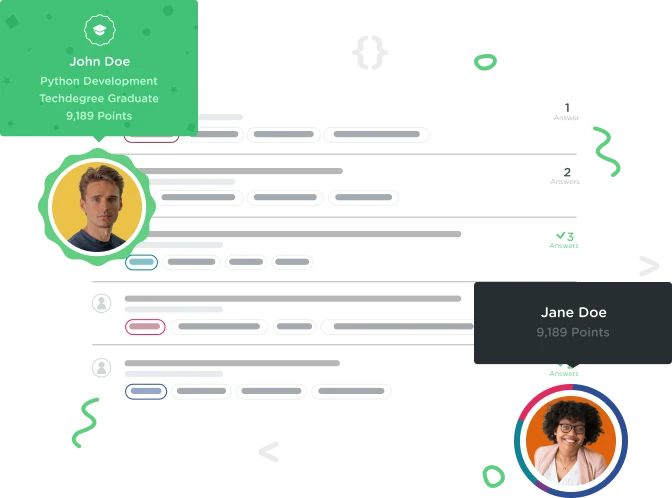
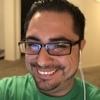
Sean Flores
Courses Plus Student 1,159 PointsAm I missing something? I keep running into the message "Bummer! Try again!" C# Basics Final Task 2 of 2
The directions say to add an if/else conditional to print the message "You must enter a positive number." When a user enters a number < 0 into the console window.
So far I've tried:
Using <= 0 as the operator for my conditional statement though this didn't work, so changed it back.
I tried commenting out the continue statement at the end of the first part of the if statement
I moved the Writeline Method that asks the user to type the number out of the Try block.
I tried adding the other exception that can be handled from the int.parse exceptions
I've been dumping the coding challenges, into Visual Studio 2017 and testing the results while I've been working on these Challenge Tasks. When I do my own testing, I find the code behaves as expected. However when I click "Check Work" with the code below I receive the message " Bummer! Try again!", with no indication of what was incorrect from the Preview window. Also after a while, the website complains of a connection error and asks me to reload the page.
Can anyone point out what I'm missing?
You help is appreciated!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
while (true)
{
var input = Console.ReadLine();
try
{
int count = int.Parse(input);
if (count < 0)
{
Console.WriteLine("You must enter a positive number.");
//continue;
}
int i = 0;
while (i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
break;
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
catch (ArgumentNullException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
catch (OverflowException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}
4 Answers
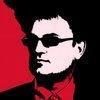
Robert Anthony
19,952 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try {
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
int count = int.Parse(input);
if (count <0) {
Console.WriteLine("You must enter a positive number.");
} else {
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
}
catch (FormatException) {
Console.WriteLine("You must enter a whole number.");
}
}
}
}
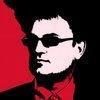
Robert Anthony
19,952 Points= means greater than or equal to zero. You want less than or equal to zero.
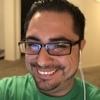
Sean Flores
Courses Plus Student 1,159 PointsSorry, there was a typo in my question. Additionally, in my code I am using only count < 0.
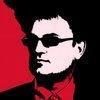
Robert Anthony
19,952 PointsYou need to put the rest of the try statements in an else, as they will currently run and this is not what is needed.
if (count < 0) {
Console.WriteLine("You must enter a positive number.");
} else {
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
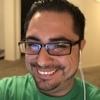
Sean Flores
Courses Plus Student 1,159 PointsHi Robert,
Thanks for your reply.
I to tried adding an else portion of the conditional statement to no avail. I also attempted commenting out the break, and continue portions of the code to see if I that would change the behavior. However, I am still encountering the "Bummer! Try again!" message. Also if I comment out the break portion of the code, the error changes to "Bummer! Your code took to long to execute!" I think this is because the while block can never finish executing without something to tell it to break out. I wonder what else I could be missing?
Below is the code I am now attempting to execute for reference:
using System;
namespace Treehouse.CodeChallenges { class Program { static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
while (true)
{
var input = Console.ReadLine();
try
{
int count = int.Parse(input);
if (count < 0)
{
Console.WriteLine("You must enter a positive number.");
continue;
}
else
{
int i = 0;
while (i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
break;
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
catch (ArgumentNullException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
catch (OverflowException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}
Sean Flores
Courses Plus Student 1,159 PointsSean Flores
Courses Plus Student 1,159 PointsThanks Robert!
I see where my error was. I added an unnecessary while loop outside the try block that was prompting the user to re-enter their input. This was to allow the code to be executed properly once the user inputted the correct string. This was not required as part of the coding challenge.